Showing
246 changed files
with
4789 additions
and
0 deletions
node_modules/.bin/ejs
0 → 100644
1 | +#!/bin/sh | ||
2 | +basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')") | ||
3 | + | ||
4 | +case `uname` in | ||
5 | + *CYGWIN*|*MINGW*|*MSYS*) basedir=`cygpath -w "$basedir"`;; | ||
6 | +esac | ||
7 | + | ||
8 | +if [ -x "$basedir/node" ]; then | ||
9 | + "$basedir/node" "$basedir/../ejs/bin/cli.js" "$@" | ||
10 | + ret=$? | ||
11 | +else | ||
12 | + node "$basedir/../ejs/bin/cli.js" "$@" | ||
13 | + ret=$? | ||
14 | +fi | ||
15 | +exit $ret |
node_modules/.bin/ejs.cmd
0 → 100644
1 | +@ECHO off | ||
2 | +SETLOCAL | ||
3 | +CALL :find_dp0 | ||
4 | + | ||
5 | +IF EXIST "%dp0%\node.exe" ( | ||
6 | + SET "_prog=%dp0%\node.exe" | ||
7 | +) ELSE ( | ||
8 | + SET "_prog=node" | ||
9 | + SET PATHEXT=%PATHEXT:;.JS;=;% | ||
10 | +) | ||
11 | + | ||
12 | +"%_prog%" "%dp0%\..\ejs\bin\cli.js" %* | ||
13 | +ENDLOCAL | ||
14 | +EXIT /b %errorlevel% | ||
15 | +:find_dp0 | ||
16 | +SET dp0=%~dp0 | ||
17 | +EXIT /b |
node_modules/.bin/ejs.ps1
0 → 100644
1 | +#!/usr/bin/env pwsh | ||
2 | +$basedir=Split-Path $MyInvocation.MyCommand.Definition -Parent | ||
3 | + | ||
4 | +$exe="" | ||
5 | +if ($PSVersionTable.PSVersion -lt "6.0" -or $IsWindows) { | ||
6 | + # Fix case when both the Windows and Linux builds of Node | ||
7 | + # are installed in the same directory | ||
8 | + $exe=".exe" | ||
9 | +} | ||
10 | +$ret=0 | ||
11 | +if (Test-Path "$basedir/node$exe") { | ||
12 | + & "$basedir/node$exe" "$basedir/../ejs/bin/cli.js" $args | ||
13 | + $ret=$LASTEXITCODE | ||
14 | +} else { | ||
15 | + & "node$exe" "$basedir/../ejs/bin/cli.js" $args | ||
16 | + $ret=$LASTEXITCODE | ||
17 | +} | ||
18 | +exit $ret |
node_modules/.bin/jake
0 → 100644
1 | +#!/bin/sh | ||
2 | +basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')") | ||
3 | + | ||
4 | +case `uname` in | ||
5 | + *CYGWIN*|*MINGW*|*MSYS*) basedir=`cygpath -w "$basedir"`;; | ||
6 | +esac | ||
7 | + | ||
8 | +if [ -x "$basedir/node" ]; then | ||
9 | + "$basedir/node" "$basedir/../jake/bin/cli.js" "$@" | ||
10 | + ret=$? | ||
11 | +else | ||
12 | + node "$basedir/../jake/bin/cli.js" "$@" | ||
13 | + ret=$? | ||
14 | +fi | ||
15 | +exit $ret |
node_modules/.bin/jake.cmd
0 → 100644
1 | +@ECHO off | ||
2 | +SETLOCAL | ||
3 | +CALL :find_dp0 | ||
4 | + | ||
5 | +IF EXIST "%dp0%\node.exe" ( | ||
6 | + SET "_prog=%dp0%\node.exe" | ||
7 | +) ELSE ( | ||
8 | + SET "_prog=node" | ||
9 | + SET PATHEXT=%PATHEXT:;.JS;=;% | ||
10 | +) | ||
11 | + | ||
12 | +"%_prog%" "%dp0%\..\jake\bin\cli.js" %* | ||
13 | +ENDLOCAL | ||
14 | +EXIT /b %errorlevel% | ||
15 | +:find_dp0 | ||
16 | +SET dp0=%~dp0 | ||
17 | +EXIT /b |
node_modules/.bin/jake.ps1
0 → 100644
1 | +#!/usr/bin/env pwsh | ||
2 | +$basedir=Split-Path $MyInvocation.MyCommand.Definition -Parent | ||
3 | + | ||
4 | +$exe="" | ||
5 | +if ($PSVersionTable.PSVersion -lt "6.0" -or $IsWindows) { | ||
6 | + # Fix case when both the Windows and Linux builds of Node | ||
7 | + # are installed in the same directory | ||
8 | + $exe=".exe" | ||
9 | +} | ||
10 | +$ret=0 | ||
11 | +if (Test-Path "$basedir/node$exe") { | ||
12 | + & "$basedir/node$exe" "$basedir/../jake/bin/cli.js" $args | ||
13 | + $ret=$LASTEXITCODE | ||
14 | +} else { | ||
15 | + & "node$exe" "$basedir/../jake/bin/cli.js" $args | ||
16 | + $ret=$LASTEXITCODE | ||
17 | +} | ||
18 | +exit $ret |
node_modules/ansi-styles/index.js
0 → 100644
1 | +'use strict'; | ||
2 | +const colorConvert = require('color-convert'); | ||
3 | + | ||
4 | +const wrapAnsi16 = (fn, offset) => function () { | ||
5 | + const code = fn.apply(colorConvert, arguments); | ||
6 | + return `\u001B[${code + offset}m`; | ||
7 | +}; | ||
8 | + | ||
9 | +const wrapAnsi256 = (fn, offset) => function () { | ||
10 | + const code = fn.apply(colorConvert, arguments); | ||
11 | + return `\u001B[${38 + offset};5;${code}m`; | ||
12 | +}; | ||
13 | + | ||
14 | +const wrapAnsi16m = (fn, offset) => function () { | ||
15 | + const rgb = fn.apply(colorConvert, arguments); | ||
16 | + return `\u001B[${38 + offset};2;${rgb[0]};${rgb[1]};${rgb[2]}m`; | ||
17 | +}; | ||
18 | + | ||
19 | +function assembleStyles() { | ||
20 | + const codes = new Map(); | ||
21 | + const styles = { | ||
22 | + modifier: { | ||
23 | + reset: [0, 0], | ||
24 | + // 21 isn't widely supported and 22 does the same thing | ||
25 | + bold: [1, 22], | ||
26 | + dim: [2, 22], | ||
27 | + italic: [3, 23], | ||
28 | + underline: [4, 24], | ||
29 | + inverse: [7, 27], | ||
30 | + hidden: [8, 28], | ||
31 | + strikethrough: [9, 29] | ||
32 | + }, | ||
33 | + color: { | ||
34 | + black: [30, 39], | ||
35 | + red: [31, 39], | ||
36 | + green: [32, 39], | ||
37 | + yellow: [33, 39], | ||
38 | + blue: [34, 39], | ||
39 | + magenta: [35, 39], | ||
40 | + cyan: [36, 39], | ||
41 | + white: [37, 39], | ||
42 | + gray: [90, 39], | ||
43 | + | ||
44 | + // Bright color | ||
45 | + redBright: [91, 39], | ||
46 | + greenBright: [92, 39], | ||
47 | + yellowBright: [93, 39], | ||
48 | + blueBright: [94, 39], | ||
49 | + magentaBright: [95, 39], | ||
50 | + cyanBright: [96, 39], | ||
51 | + whiteBright: [97, 39] | ||
52 | + }, | ||
53 | + bgColor: { | ||
54 | + bgBlack: [40, 49], | ||
55 | + bgRed: [41, 49], | ||
56 | + bgGreen: [42, 49], | ||
57 | + bgYellow: [43, 49], | ||
58 | + bgBlue: [44, 49], | ||
59 | + bgMagenta: [45, 49], | ||
60 | + bgCyan: [46, 49], | ||
61 | + bgWhite: [47, 49], | ||
62 | + | ||
63 | + // Bright color | ||
64 | + bgBlackBright: [100, 49], | ||
65 | + bgRedBright: [101, 49], | ||
66 | + bgGreenBright: [102, 49], | ||
67 | + bgYellowBright: [103, 49], | ||
68 | + bgBlueBright: [104, 49], | ||
69 | + bgMagentaBright: [105, 49], | ||
70 | + bgCyanBright: [106, 49], | ||
71 | + bgWhiteBright: [107, 49] | ||
72 | + } | ||
73 | + }; | ||
74 | + | ||
75 | + // Fix humans | ||
76 | + styles.color.grey = styles.color.gray; | ||
77 | + | ||
78 | + for (const groupName of Object.keys(styles)) { | ||
79 | + const group = styles[groupName]; | ||
80 | + | ||
81 | + for (const styleName of Object.keys(group)) { | ||
82 | + const style = group[styleName]; | ||
83 | + | ||
84 | + styles[styleName] = { | ||
85 | + open: `\u001B[${style[0]}m`, | ||
86 | + close: `\u001B[${style[1]}m` | ||
87 | + }; | ||
88 | + | ||
89 | + group[styleName] = styles[styleName]; | ||
90 | + | ||
91 | + codes.set(style[0], style[1]); | ||
92 | + } | ||
93 | + | ||
94 | + Object.defineProperty(styles, groupName, { | ||
95 | + value: group, | ||
96 | + enumerable: false | ||
97 | + }); | ||
98 | + | ||
99 | + Object.defineProperty(styles, 'codes', { | ||
100 | + value: codes, | ||
101 | + enumerable: false | ||
102 | + }); | ||
103 | + } | ||
104 | + | ||
105 | + const ansi2ansi = n => n; | ||
106 | + const rgb2rgb = (r, g, b) => [r, g, b]; | ||
107 | + | ||
108 | + styles.color.close = '\u001B[39m'; | ||
109 | + styles.bgColor.close = '\u001B[49m'; | ||
110 | + | ||
111 | + styles.color.ansi = { | ||
112 | + ansi: wrapAnsi16(ansi2ansi, 0) | ||
113 | + }; | ||
114 | + styles.color.ansi256 = { | ||
115 | + ansi256: wrapAnsi256(ansi2ansi, 0) | ||
116 | + }; | ||
117 | + styles.color.ansi16m = { | ||
118 | + rgb: wrapAnsi16m(rgb2rgb, 0) | ||
119 | + }; | ||
120 | + | ||
121 | + styles.bgColor.ansi = { | ||
122 | + ansi: wrapAnsi16(ansi2ansi, 10) | ||
123 | + }; | ||
124 | + styles.bgColor.ansi256 = { | ||
125 | + ansi256: wrapAnsi256(ansi2ansi, 10) | ||
126 | + }; | ||
127 | + styles.bgColor.ansi16m = { | ||
128 | + rgb: wrapAnsi16m(rgb2rgb, 10) | ||
129 | + }; | ||
130 | + | ||
131 | + for (let key of Object.keys(colorConvert)) { | ||
132 | + if (typeof colorConvert[key] !== 'object') { | ||
133 | + continue; | ||
134 | + } | ||
135 | + | ||
136 | + const suite = colorConvert[key]; | ||
137 | + | ||
138 | + if (key === 'ansi16') { | ||
139 | + key = 'ansi'; | ||
140 | + } | ||
141 | + | ||
142 | + if ('ansi16' in suite) { | ||
143 | + styles.color.ansi[key] = wrapAnsi16(suite.ansi16, 0); | ||
144 | + styles.bgColor.ansi[key] = wrapAnsi16(suite.ansi16, 10); | ||
145 | + } | ||
146 | + | ||
147 | + if ('ansi256' in suite) { | ||
148 | + styles.color.ansi256[key] = wrapAnsi256(suite.ansi256, 0); | ||
149 | + styles.bgColor.ansi256[key] = wrapAnsi256(suite.ansi256, 10); | ||
150 | + } | ||
151 | + | ||
152 | + if ('rgb' in suite) { | ||
153 | + styles.color.ansi16m[key] = wrapAnsi16m(suite.rgb, 0); | ||
154 | + styles.bgColor.ansi16m[key] = wrapAnsi16m(suite.rgb, 10); | ||
155 | + } | ||
156 | + } | ||
157 | + | ||
158 | + return styles; | ||
159 | +} | ||
160 | + | ||
161 | +// Make the export immutable | ||
162 | +Object.defineProperty(module, 'exports', { | ||
163 | + enumerable: true, | ||
164 | + get: assembleStyles | ||
165 | +}); |
node_modules/ansi-styles/license
0 → 100644
1 | +MIT License | ||
2 | + | ||
3 | +Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: | ||
6 | + | ||
7 | +The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. | ||
8 | + | ||
9 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
node_modules/ansi-styles/package.json
0 → 100644
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "ansi-styles@3.2.1", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "ansi-styles@3.2.1", | ||
9 | + "_id": "ansi-styles@3.2.1", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha512-VT0ZI6kZRdTh8YyJw3SMbYm/u+NqfsAxEpWO0Pf9sq8/e94WxxOpPKx9FR1FlyCtOVDNOQ+8ntlqFxiRc+r5qA==", | ||
12 | + "_location": "/ansi-styles", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "ansi-styles@3.2.1", | ||
18 | + "name": "ansi-styles", | ||
19 | + "escapedName": "ansi-styles", | ||
20 | + "rawSpec": "3.2.1", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "3.2.1" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/chalk" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/ansi-styles/-/ansi-styles-3.2.1.tgz", | ||
28 | + "_spec": "3.2.1", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "author": { | ||
31 | + "name": "Sindre Sorhus", | ||
32 | + "email": "sindresorhus@gmail.com", | ||
33 | + "url": "sindresorhus.com" | ||
34 | + }, | ||
35 | + "ava": { | ||
36 | + "require": "babel-polyfill" | ||
37 | + }, | ||
38 | + "bugs": { | ||
39 | + "url": "https://github.com/chalk/ansi-styles/issues" | ||
40 | + }, | ||
41 | + "dependencies": { | ||
42 | + "color-convert": "^1.9.0" | ||
43 | + }, | ||
44 | + "description": "ANSI escape codes for styling strings in the terminal", | ||
45 | + "devDependencies": { | ||
46 | + "ava": "*", | ||
47 | + "babel-polyfill": "^6.23.0", | ||
48 | + "svg-term-cli": "^2.1.1", | ||
49 | + "xo": "*" | ||
50 | + }, | ||
51 | + "engines": { | ||
52 | + "node": ">=4" | ||
53 | + }, | ||
54 | + "files": [ | ||
55 | + "index.js" | ||
56 | + ], | ||
57 | + "homepage": "https://github.com/chalk/ansi-styles#readme", | ||
58 | + "keywords": [ | ||
59 | + "ansi", | ||
60 | + "styles", | ||
61 | + "color", | ||
62 | + "colour", | ||
63 | + "colors", | ||
64 | + "terminal", | ||
65 | + "console", | ||
66 | + "cli", | ||
67 | + "string", | ||
68 | + "tty", | ||
69 | + "escape", | ||
70 | + "formatting", | ||
71 | + "rgb", | ||
72 | + "256", | ||
73 | + "shell", | ||
74 | + "xterm", | ||
75 | + "log", | ||
76 | + "logging", | ||
77 | + "command-line", | ||
78 | + "text" | ||
79 | + ], | ||
80 | + "license": "MIT", | ||
81 | + "name": "ansi-styles", | ||
82 | + "repository": { | ||
83 | + "type": "git", | ||
84 | + "url": "git+https://github.com/chalk/ansi-styles.git" | ||
85 | + }, | ||
86 | + "scripts": { | ||
87 | + "screenshot": "svg-term --command='node screenshot' --out=screenshot.svg --padding=3 --width=55 --height=3 --at=1000 --no-cursor", | ||
88 | + "test": "xo && ava" | ||
89 | + }, | ||
90 | + "version": "3.2.1" | ||
91 | +} |
node_modules/ansi-styles/readme.md
0 → 100644
1 | +# ansi-styles [](https://travis-ci.org/chalk/ansi-styles) | ||
2 | + | ||
3 | +> [ANSI escape codes](http://en.wikipedia.org/wiki/ANSI_escape_code#Colors_and_Styles) for styling strings in the terminal | ||
4 | + | ||
5 | +You probably want the higher-level [chalk](https://github.com/chalk/chalk) module for styling your strings. | ||
6 | + | ||
7 | +<img src="https://cdn.rawgit.com/chalk/ansi-styles/8261697c95bf34b6c7767e2cbe9941a851d59385/screenshot.svg" width="900"> | ||
8 | + | ||
9 | + | ||
10 | +## Install | ||
11 | + | ||
12 | +``` | ||
13 | +$ npm install ansi-styles | ||
14 | +``` | ||
15 | + | ||
16 | + | ||
17 | +## Usage | ||
18 | + | ||
19 | +```js | ||
20 | +const style = require('ansi-styles'); | ||
21 | + | ||
22 | +console.log(`${style.green.open}Hello world!${style.green.close}`); | ||
23 | + | ||
24 | + | ||
25 | +// Color conversion between 16/256/truecolor | ||
26 | +// NOTE: If conversion goes to 16 colors or 256 colors, the original color | ||
27 | +// may be degraded to fit that color palette. This means terminals | ||
28 | +// that do not support 16 million colors will best-match the | ||
29 | +// original color. | ||
30 | +console.log(style.bgColor.ansi.hsl(120, 80, 72) + 'Hello world!' + style.bgColor.close); | ||
31 | +console.log(style.color.ansi256.rgb(199, 20, 250) + 'Hello world!' + style.color.close); | ||
32 | +console.log(style.color.ansi16m.hex('#ABCDEF') + 'Hello world!' + style.color.close); | ||
33 | +``` | ||
34 | + | ||
35 | +## API | ||
36 | + | ||
37 | +Each style has an `open` and `close` property. | ||
38 | + | ||
39 | + | ||
40 | +## Styles | ||
41 | + | ||
42 | +### Modifiers | ||
43 | + | ||
44 | +- `reset` | ||
45 | +- `bold` | ||
46 | +- `dim` | ||
47 | +- `italic` *(Not widely supported)* | ||
48 | +- `underline` | ||
49 | +- `inverse` | ||
50 | +- `hidden` | ||
51 | +- `strikethrough` *(Not widely supported)* | ||
52 | + | ||
53 | +### Colors | ||
54 | + | ||
55 | +- `black` | ||
56 | +- `red` | ||
57 | +- `green` | ||
58 | +- `yellow` | ||
59 | +- `blue` | ||
60 | +- `magenta` | ||
61 | +- `cyan` | ||
62 | +- `white` | ||
63 | +- `gray` ("bright black") | ||
64 | +- `redBright` | ||
65 | +- `greenBright` | ||
66 | +- `yellowBright` | ||
67 | +- `blueBright` | ||
68 | +- `magentaBright` | ||
69 | +- `cyanBright` | ||
70 | +- `whiteBright` | ||
71 | + | ||
72 | +### Background colors | ||
73 | + | ||
74 | +- `bgBlack` | ||
75 | +- `bgRed` | ||
76 | +- `bgGreen` | ||
77 | +- `bgYellow` | ||
78 | +- `bgBlue` | ||
79 | +- `bgMagenta` | ||
80 | +- `bgCyan` | ||
81 | +- `bgWhite` | ||
82 | +- `bgBlackBright` | ||
83 | +- `bgRedBright` | ||
84 | +- `bgGreenBright` | ||
85 | +- `bgYellowBright` | ||
86 | +- `bgBlueBright` | ||
87 | +- `bgMagentaBright` | ||
88 | +- `bgCyanBright` | ||
89 | +- `bgWhiteBright` | ||
90 | + | ||
91 | + | ||
92 | +## Advanced usage | ||
93 | + | ||
94 | +By default, you get a map of styles, but the styles are also available as groups. They are non-enumerable so they don't show up unless you access them explicitly. This makes it easier to expose only a subset in a higher-level module. | ||
95 | + | ||
96 | +- `style.modifier` | ||
97 | +- `style.color` | ||
98 | +- `style.bgColor` | ||
99 | + | ||
100 | +###### Example | ||
101 | + | ||
102 | +```js | ||
103 | +console.log(style.color.green.open); | ||
104 | +``` | ||
105 | + | ||
106 | +Raw escape codes (i.e. without the CSI escape prefix `\u001B[` and render mode postfix `m`) are available under `style.codes`, which returns a `Map` with the open codes as keys and close codes as values. | ||
107 | + | ||
108 | +###### Example | ||
109 | + | ||
110 | +```js | ||
111 | +console.log(style.codes.get(36)); | ||
112 | +//=> 39 | ||
113 | +``` | ||
114 | + | ||
115 | + | ||
116 | +## [256 / 16 million (TrueColor) support](https://gist.github.com/XVilka/8346728) | ||
117 | + | ||
118 | +`ansi-styles` uses the [`color-convert`](https://github.com/Qix-/color-convert) package to allow for converting between various colors and ANSI escapes, with support for 256 and 16 million colors. | ||
119 | + | ||
120 | +To use these, call the associated conversion function with the intended output, for example: | ||
121 | + | ||
122 | +```js | ||
123 | +style.color.ansi.rgb(100, 200, 15); // RGB to 16 color ansi foreground code | ||
124 | +style.bgColor.ansi.rgb(100, 200, 15); // RGB to 16 color ansi background code | ||
125 | + | ||
126 | +style.color.ansi256.hsl(120, 100, 60); // HSL to 256 color ansi foreground code | ||
127 | +style.bgColor.ansi256.hsl(120, 100, 60); // HSL to 256 color ansi foreground code | ||
128 | + | ||
129 | +style.color.ansi16m.hex('#C0FFEE'); // Hex (RGB) to 16 million color foreground code | ||
130 | +style.bgColor.ansi16m.hex('#C0FFEE'); // Hex (RGB) to 16 million color background code | ||
131 | +``` | ||
132 | + | ||
133 | + | ||
134 | +## Related | ||
135 | + | ||
136 | +- [ansi-escapes](https://github.com/sindresorhus/ansi-escapes) - ANSI escape codes for manipulating the terminal | ||
137 | + | ||
138 | + | ||
139 | +## Maintainers | ||
140 | + | ||
141 | +- [Sindre Sorhus](https://github.com/sindresorhus) | ||
142 | +- [Josh Junon](https://github.com/qix-) | ||
143 | + | ||
144 | + | ||
145 | +## License | ||
146 | + | ||
147 | +MIT |
node_modules/async/.travis.yml
0 → 100644
node_modules/async/LICENSE
0 → 100644
1 | +Copyright (c) 2010-2014 Caolan McMahon | ||
2 | + | ||
3 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
4 | +of this software and associated documentation files (the "Software"), to deal | ||
5 | +in the Software without restriction, including without limitation the rights | ||
6 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
7 | +copies of the Software, and to permit persons to whom the Software is | ||
8 | +furnished to do so, subject to the following conditions: | ||
9 | + | ||
10 | +The above copyright notice and this permission notice shall be included in | ||
11 | +all copies or substantial portions of the Software. | ||
12 | + | ||
13 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
14 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
15 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
16 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
17 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
18 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN | ||
19 | +THE SOFTWARE. |
node_modules/async/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/async/bower.json
0 → 100644
1 | +{ | ||
2 | + "name": "async", | ||
3 | + "description": "Higher-order functions and common patterns for asynchronous code", | ||
4 | + "version": "0.9.2", | ||
5 | + "main": "lib/async.js", | ||
6 | + "keywords": [ | ||
7 | + "async", | ||
8 | + "callback", | ||
9 | + "utility", | ||
10 | + "module" | ||
11 | + ], | ||
12 | + "license": "MIT", | ||
13 | + "repository": { | ||
14 | + "type": "git", | ||
15 | + "url": "https://github.com/caolan/async.git" | ||
16 | + }, | ||
17 | + "devDependencies": { | ||
18 | + "nodeunit": ">0.0.0", | ||
19 | + "uglify-js": "1.2.x", | ||
20 | + "nodelint": ">0.0.0", | ||
21 | + "lodash": ">=2.4.1" | ||
22 | + }, | ||
23 | + "moduleType": [ | ||
24 | + "amd", | ||
25 | + "globals", | ||
26 | + "node" | ||
27 | + ], | ||
28 | + "ignore": [ | ||
29 | + "**/.*", | ||
30 | + "node_modules", | ||
31 | + "bower_components", | ||
32 | + "test", | ||
33 | + "tests" | ||
34 | + ], | ||
35 | + "authors": [ | ||
36 | + "Caolan McMahon" | ||
37 | + ] | ||
38 | +} | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
node_modules/async/component.json
0 → 100644
1 | +{ | ||
2 | + "name": "async", | ||
3 | + "description": "Higher-order functions and common patterns for asynchronous code", | ||
4 | + "version": "0.9.2", | ||
5 | + "keywords": [ | ||
6 | + "async", | ||
7 | + "callback", | ||
8 | + "utility", | ||
9 | + "module" | ||
10 | + ], | ||
11 | + "license": "MIT", | ||
12 | + "repository": "caolan/async", | ||
13 | + "scripts": [ | ||
14 | + "lib/async.js" | ||
15 | + ] | ||
16 | +} | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
node_modules/async/lib/async.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/async/package.json
0 → 100644
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "async@0.9.2", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "async@0.9.2", | ||
9 | + "_id": "async@0.9.2", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha1-rqdNXmHB+JlhO/ZL2mbUx48v0X0=", | ||
12 | + "_location": "/async", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "async@0.9.2", | ||
18 | + "name": "async", | ||
19 | + "escapedName": "async", | ||
20 | + "rawSpec": "0.9.2", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "0.9.2" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/jake" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/async/-/async-0.9.2.tgz", | ||
28 | + "_spec": "0.9.2", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "author": { | ||
31 | + "name": "Caolan McMahon" | ||
32 | + }, | ||
33 | + "bugs": { | ||
34 | + "url": "https://github.com/caolan/async/issues" | ||
35 | + }, | ||
36 | + "description": "Higher-order functions and common patterns for asynchronous code", | ||
37 | + "devDependencies": { | ||
38 | + "lodash": ">=2.4.1", | ||
39 | + "nodelint": ">0.0.0", | ||
40 | + "nodeunit": ">0.0.0", | ||
41 | + "uglify-js": "1.2.x" | ||
42 | + }, | ||
43 | + "homepage": "https://github.com/caolan/async#readme", | ||
44 | + "jam": { | ||
45 | + "main": "lib/async.js", | ||
46 | + "include": [ | ||
47 | + "lib/async.js", | ||
48 | + "README.md", | ||
49 | + "LICENSE" | ||
50 | + ], | ||
51 | + "categories": [ | ||
52 | + "Utilities" | ||
53 | + ] | ||
54 | + }, | ||
55 | + "keywords": [ | ||
56 | + "async", | ||
57 | + "callback", | ||
58 | + "utility", | ||
59 | + "module" | ||
60 | + ], | ||
61 | + "license": "MIT", | ||
62 | + "main": "lib/async.js", | ||
63 | + "name": "async", | ||
64 | + "repository": { | ||
65 | + "type": "git", | ||
66 | + "url": "git+https://github.com/caolan/async.git" | ||
67 | + }, | ||
68 | + "scripts": { | ||
69 | + "test": "nodeunit test/test-async.js" | ||
70 | + }, | ||
71 | + "spm": { | ||
72 | + "main": "lib/async.js" | ||
73 | + }, | ||
74 | + "version": "0.9.2", | ||
75 | + "volo": { | ||
76 | + "main": "lib/async.js", | ||
77 | + "ignore": [ | ||
78 | + "**/.*", | ||
79 | + "node_modules", | ||
80 | + "bower_components", | ||
81 | + "test", | ||
82 | + "tests" | ||
83 | + ] | ||
84 | + } | ||
85 | +} |
1 | +#!/usr/bin/env node | ||
2 | + | ||
3 | +// This should probably be its own module but complaints about bower/etc. | ||
4 | +// support keep coming up and I'd rather just enable the workflow here for now | ||
5 | +// and figure out where this should live later. -- @beaugunderson | ||
6 | + | ||
7 | +var fs = require('fs'); | ||
8 | +var _ = require('lodash'); | ||
9 | + | ||
10 | +var packageJson = require('../package.json'); | ||
11 | + | ||
12 | +var IGNORES = ['**/.*', 'node_modules', 'bower_components', 'test', 'tests']; | ||
13 | +var INCLUDES = ['lib/async.js', 'README.md', 'LICENSE']; | ||
14 | +var REPOSITORY_NAME = 'caolan/async'; | ||
15 | + | ||
16 | +packageJson.jam = { | ||
17 | + main: packageJson.main, | ||
18 | + include: INCLUDES, | ||
19 | + categories: ['Utilities'] | ||
20 | +}; | ||
21 | + | ||
22 | +packageJson.spm = { | ||
23 | + main: packageJson.main | ||
24 | +}; | ||
25 | + | ||
26 | +packageJson.volo = { | ||
27 | + main: packageJson.main, | ||
28 | + ignore: IGNORES | ||
29 | +}; | ||
30 | + | ||
31 | +var bowerSpecific = { | ||
32 | + moduleType: ['amd', 'globals', 'node'], | ||
33 | + ignore: IGNORES, | ||
34 | + authors: [packageJson.author] | ||
35 | +}; | ||
36 | + | ||
37 | +var bowerInclude = ['name', 'description', 'version', 'main', 'keywords', | ||
38 | + 'license', 'homepage', 'repository', 'devDependencies']; | ||
39 | + | ||
40 | +var componentSpecific = { | ||
41 | + repository: REPOSITORY_NAME, | ||
42 | + scripts: [packageJson.main] | ||
43 | +}; | ||
44 | + | ||
45 | +var componentInclude = ['name', 'description', 'version', 'keywords', | ||
46 | + 'license']; | ||
47 | + | ||
48 | +var bowerJson = _.merge({}, _.pick(packageJson, bowerInclude), bowerSpecific); | ||
49 | +var componentJson = _.merge({}, _.pick(packageJson, componentInclude), componentSpecific); | ||
50 | + | ||
51 | +fs.writeFileSync('./bower.json', JSON.stringify(bowerJson, null, 2)); | ||
52 | +fs.writeFileSync('./component.json', JSON.stringify(componentJson, null, 2)); | ||
53 | +fs.writeFileSync('./package.json', JSON.stringify(packageJson, null, 2)); |
node_modules/balanced-match/LICENSE.md
0 → 100644
1 | +(MIT) | ||
2 | + | ||
3 | +Copyright (c) 2013 Julian Gruber <julian@juliangruber.com> | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
6 | +this software and associated documentation files (the "Software"), to deal in | ||
7 | +the Software without restriction, including without limitation the rights to | ||
8 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies | ||
9 | +of the Software, and to permit persons to whom the Software is furnished to do | ||
10 | +so, subject to the following conditions: | ||
11 | + | ||
12 | +The above copyright notice and this permission notice shall be included in all | ||
13 | +copies or substantial portions of the Software. | ||
14 | + | ||
15 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
16 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
17 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
18 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
19 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
20 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
21 | +SOFTWARE. |
node_modules/balanced-match/README.md
0 → 100644
1 | +# balanced-match | ||
2 | + | ||
3 | +Match balanced string pairs, like `{` and `}` or `<b>` and `</b>`. Supports regular expressions as well! | ||
4 | + | ||
5 | +[](http://travis-ci.org/juliangruber/balanced-match) | ||
6 | +[](https://www.npmjs.org/package/balanced-match) | ||
7 | + | ||
8 | +[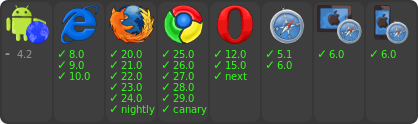](https://ci.testling.com/juliangruber/balanced-match) | ||
9 | + | ||
10 | +## Example | ||
11 | + | ||
12 | +Get the first matching pair of braces: | ||
13 | + | ||
14 | +```js | ||
15 | +var balanced = require('balanced-match'); | ||
16 | + | ||
17 | +console.log(balanced('{', '}', 'pre{in{nested}}post')); | ||
18 | +console.log(balanced('{', '}', 'pre{first}between{second}post')); | ||
19 | +console.log(balanced(/\s+\{\s+/, /\s+\}\s+/, 'pre { in{nest} } post')); | ||
20 | +``` | ||
21 | + | ||
22 | +The matches are: | ||
23 | + | ||
24 | +```bash | ||
25 | +$ node example.js | ||
26 | +{ start: 3, end: 14, pre: 'pre', body: 'in{nested}', post: 'post' } | ||
27 | +{ start: 3, | ||
28 | + end: 9, | ||
29 | + pre: 'pre', | ||
30 | + body: 'first', | ||
31 | + post: 'between{second}post' } | ||
32 | +{ start: 3, end: 17, pre: 'pre', body: 'in{nest}', post: 'post' } | ||
33 | +``` | ||
34 | + | ||
35 | +## API | ||
36 | + | ||
37 | +### var m = balanced(a, b, str) | ||
38 | + | ||
39 | +For the first non-nested matching pair of `a` and `b` in `str`, return an | ||
40 | +object with those keys: | ||
41 | + | ||
42 | +* **start** the index of the first match of `a` | ||
43 | +* **end** the index of the matching `b` | ||
44 | +* **pre** the preamble, `a` and `b` not included | ||
45 | +* **body** the match, `a` and `b` not included | ||
46 | +* **post** the postscript, `a` and `b` not included | ||
47 | + | ||
48 | +If there's no match, `undefined` will be returned. | ||
49 | + | ||
50 | +If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `['{', 'a', '']` and `{a}}` will match `['', 'a', '}']`. | ||
51 | + | ||
52 | +### var r = balanced.range(a, b, str) | ||
53 | + | ||
54 | +For the first non-nested matching pair of `a` and `b` in `str`, return an | ||
55 | +array with indexes: `[ <a index>, <b index> ]`. | ||
56 | + | ||
57 | +If there's no match, `undefined` will be returned. | ||
58 | + | ||
59 | +If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `[ 1, 3 ]` and `{a}}` will match `[0, 2]`. | ||
60 | + | ||
61 | +## Installation | ||
62 | + | ||
63 | +With [npm](https://npmjs.org) do: | ||
64 | + | ||
65 | +```bash | ||
66 | +npm install balanced-match | ||
67 | +``` | ||
68 | + | ||
69 | +## Security contact information | ||
70 | + | ||
71 | +To report a security vulnerability, please use the | ||
72 | +[Tidelift security contact](https://tidelift.com/security). | ||
73 | +Tidelift will coordinate the fix and disclosure. | ||
74 | + | ||
75 | +## License | ||
76 | + | ||
77 | +(MIT) | ||
78 | + | ||
79 | +Copyright (c) 2013 Julian Gruber <julian@juliangruber.com> | ||
80 | + | ||
81 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
82 | +this software and associated documentation files (the "Software"), to deal in | ||
83 | +the Software without restriction, including without limitation the rights to | ||
84 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies | ||
85 | +of the Software, and to permit persons to whom the Software is furnished to do | ||
86 | +so, subject to the following conditions: | ||
87 | + | ||
88 | +The above copyright notice and this permission notice shall be included in all | ||
89 | +copies or substantial portions of the Software. | ||
90 | + | ||
91 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
92 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
93 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
94 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
95 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
96 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
97 | +SOFTWARE. |
node_modules/balanced-match/index.js
0 → 100644
1 | +'use strict'; | ||
2 | +module.exports = balanced; | ||
3 | +function balanced(a, b, str) { | ||
4 | + if (a instanceof RegExp) a = maybeMatch(a, str); | ||
5 | + if (b instanceof RegExp) b = maybeMatch(b, str); | ||
6 | + | ||
7 | + var r = range(a, b, str); | ||
8 | + | ||
9 | + return r && { | ||
10 | + start: r[0], | ||
11 | + end: r[1], | ||
12 | + pre: str.slice(0, r[0]), | ||
13 | + body: str.slice(r[0] + a.length, r[1]), | ||
14 | + post: str.slice(r[1] + b.length) | ||
15 | + }; | ||
16 | +} | ||
17 | + | ||
18 | +function maybeMatch(reg, str) { | ||
19 | + var m = str.match(reg); | ||
20 | + return m ? m[0] : null; | ||
21 | +} | ||
22 | + | ||
23 | +balanced.range = range; | ||
24 | +function range(a, b, str) { | ||
25 | + var begs, beg, left, right, result; | ||
26 | + var ai = str.indexOf(a); | ||
27 | + var bi = str.indexOf(b, ai + 1); | ||
28 | + var i = ai; | ||
29 | + | ||
30 | + if (ai >= 0 && bi > 0) { | ||
31 | + if(a===b) { | ||
32 | + return [ai, bi]; | ||
33 | + } | ||
34 | + begs = []; | ||
35 | + left = str.length; | ||
36 | + | ||
37 | + while (i >= 0 && !result) { | ||
38 | + if (i == ai) { | ||
39 | + begs.push(i); | ||
40 | + ai = str.indexOf(a, i + 1); | ||
41 | + } else if (begs.length == 1) { | ||
42 | + result = [ begs.pop(), bi ]; | ||
43 | + } else { | ||
44 | + beg = begs.pop(); | ||
45 | + if (beg < left) { | ||
46 | + left = beg; | ||
47 | + right = bi; | ||
48 | + } | ||
49 | + | ||
50 | + bi = str.indexOf(b, i + 1); | ||
51 | + } | ||
52 | + | ||
53 | + i = ai < bi && ai >= 0 ? ai : bi; | ||
54 | + } | ||
55 | + | ||
56 | + if (begs.length) { | ||
57 | + result = [ left, right ]; | ||
58 | + } | ||
59 | + } | ||
60 | + | ||
61 | + return result; | ||
62 | +} |
node_modules/balanced-match/package.json
0 → 100644
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "balanced-match@1.0.2", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "balanced-match@1.0.2", | ||
9 | + "_id": "balanced-match@1.0.2", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha512-3oSeUO0TMV67hN1AmbXsK4yaqU7tjiHlbxRDZOpH0KW9+CeX4bRAaX0Anxt0tx2MrpRpWwQaPwIlISEJhYU5Pw==", | ||
12 | + "_location": "/balanced-match", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "balanced-match@1.0.2", | ||
18 | + "name": "balanced-match", | ||
19 | + "escapedName": "balanced-match", | ||
20 | + "rawSpec": "1.0.2", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "1.0.2" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/brace-expansion" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/balanced-match/-/balanced-match-1.0.2.tgz", | ||
28 | + "_spec": "1.0.2", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "author": { | ||
31 | + "name": "Julian Gruber", | ||
32 | + "email": "mail@juliangruber.com", | ||
33 | + "url": "http://juliangruber.com" | ||
34 | + }, | ||
35 | + "bugs": { | ||
36 | + "url": "https://github.com/juliangruber/balanced-match/issues" | ||
37 | + }, | ||
38 | + "description": "Match balanced character pairs, like \"{\" and \"}\"", | ||
39 | + "devDependencies": { | ||
40 | + "matcha": "^0.7.0", | ||
41 | + "tape": "^4.6.0" | ||
42 | + }, | ||
43 | + "homepage": "https://github.com/juliangruber/balanced-match", | ||
44 | + "keywords": [ | ||
45 | + "match", | ||
46 | + "regexp", | ||
47 | + "test", | ||
48 | + "balanced", | ||
49 | + "parse" | ||
50 | + ], | ||
51 | + "license": "MIT", | ||
52 | + "main": "index.js", | ||
53 | + "name": "balanced-match", | ||
54 | + "repository": { | ||
55 | + "type": "git", | ||
56 | + "url": "git://github.com/juliangruber/balanced-match.git" | ||
57 | + }, | ||
58 | + "scripts": { | ||
59 | + "bench": "matcha test/bench.js", | ||
60 | + "test": "tape test/test.js" | ||
61 | + }, | ||
62 | + "testling": { | ||
63 | + "files": "test/*.js", | ||
64 | + "browsers": [ | ||
65 | + "ie/8..latest", | ||
66 | + "firefox/20..latest", | ||
67 | + "firefox/nightly", | ||
68 | + "chrome/25..latest", | ||
69 | + "chrome/canary", | ||
70 | + "opera/12..latest", | ||
71 | + "opera/next", | ||
72 | + "safari/5.1..latest", | ||
73 | + "ipad/6.0..latest", | ||
74 | + "iphone/6.0..latest", | ||
75 | + "android-browser/4.2..latest" | ||
76 | + ] | ||
77 | + }, | ||
78 | + "version": "1.0.2" | ||
79 | +} |
node_modules/bignumber.js/CHANGELOG.md
0 → 100644
1 | +#### 9.0.0 | ||
2 | +* 27/05/2019 | ||
3 | +* For compatibility with legacy browsers, remove `Symbol` references. | ||
4 | + | ||
5 | +#### 8.1.1 | ||
6 | +* 24/02/2019 | ||
7 | +* [BUGFIX] #222 Restore missing `var` to `export BigNumber`. | ||
8 | +* Allow any key in BigNumber.Instance in *bignumber.d.ts*. | ||
9 | + | ||
10 | +#### 8.1.0 | ||
11 | +* 23/02/2019 | ||
12 | +* [NEW FEATURE] #220 Create a BigNumber using `{s, e, c}`. | ||
13 | +* [NEW FEATURE] `isBigNumber`: if `BigNumber.DEBUG` is `true`, also check that the BigNumber instance is well-formed. | ||
14 | +* Remove `instanceof` checks; just use `_isBigNumber` to identify a BigNumber instance. | ||
15 | +* Add `_isBigNumber` to prototype in *bignumber.mjs*. | ||
16 | +* Add tests for BigNumber creation from object. | ||
17 | +* Update *API.html*. | ||
18 | + | ||
19 | +#### 8.0.2 | ||
20 | +* 13/01/2019 | ||
21 | +* #209 `toPrecision` without argument should follow `toString`. | ||
22 | +* Improve *Use* section of *README*. | ||
23 | +* Optimise `toString(10)`. | ||
24 | +* Add verson number to API doc. | ||
25 | + | ||
26 | +#### 8.0.1 | ||
27 | +* 01/11/2018 | ||
28 | +* Rest parameter must be array type in *bignumber.d.ts*. | ||
29 | + | ||
30 | +#### 8.0.0 | ||
31 | +* 01/11/2018 | ||
32 | +* [NEW FEATURE] Add `BigNumber.sum` method. | ||
33 | +* [NEW FEATURE]`toFormat`: add `prefix` and `suffix` options. | ||
34 | +* [NEW FEATURE] #178 Pass custom formatting to `toFormat`. | ||
35 | +* [BREAKING CHANGE] #184 `toFraction`: return array of BigNumbers not strings. | ||
36 | +* [NEW FEATURE] #185 Enable overwrite of `valueOf` to prevent accidental addition to string. | ||
37 | +* #183 Add Node.js `crypto` requirement to documentation. | ||
38 | +* [BREAKING CHANGE] #198 Disallow signs and whitespace in custom alphabet. | ||
39 | +* [NEW FEATURE] #188 Implement `util.inspect.custom` for Node.js REPL. | ||
40 | +* #170 Make `isBigNumber` a type guard in *bignumber.d.ts*. | ||
41 | +* [BREAKING CHANGE] `BigNumber.min` and `BigNumber.max`: don't accept an array. | ||
42 | +* Update *.travis.yml*. | ||
43 | +* Remove *bower.json*. | ||
44 | + | ||
45 | +#### 7.2.1 | ||
46 | +* 24/05/2018 | ||
47 | +* Add `browser` field to *package.json*. | ||
48 | + | ||
49 | +#### 7.2.0 | ||
50 | +* 22/05/2018 | ||
51 | +* #166 Correct *.mjs* file. Remove extension from `main` field in *package.json*. | ||
52 | + | ||
53 | +#### 7.1.0 | ||
54 | +* 18/05/2018 | ||
55 | +* Add `module` field to *package.json* for *bignumber.mjs*. | ||
56 | + | ||
57 | +#### 7.0.2 | ||
58 | +* 17/05/2018 | ||
59 | +* #165 Bugfix: upper-case letters for bases 11-36 in a custom alphabet. | ||
60 | +* Add note to *README* regarding creating BigNumbers from Number values. | ||
61 | + | ||
62 | +#### 7.0.1 | ||
63 | +* 26/04/2018 | ||
64 | +* #158 Fix global object variable name typo. | ||
65 | + | ||
66 | +#### 7.0.0 | ||
67 | +* 26/04/2018 | ||
68 | +* #143 Remove global BigNumber from typings. | ||
69 | +* #144 Enable compatibility with `Object.freeze(Object.prototype)`. | ||
70 | +* #148 #123 #11 Only throw on a number primitive with more than 15 significant digits if `BigNumber.DEBUG` is `true`. | ||
71 | +* Only throw on an invalid BigNumber value if `BigNumber.DEBUG` is `true`. Return BigNumber `NaN` instead. | ||
72 | +* #154 `exponentiatedBy`: allow BigNumber exponent. | ||
73 | +* #156 Prevent Content Security Policy *unsafe-eval* issue. | ||
74 | +* `toFraction`: allow `Infinity` maximum denominator. | ||
75 | +* Comment-out some excess tests to reduce test time. | ||
76 | +* Amend indentation and other spacing. | ||
77 | + | ||
78 | +#### 6.0.0 | ||
79 | +* 26/01/2018 | ||
80 | +* #137 Implement `APLHABET` configuration option. | ||
81 | +* Remove `ERRORS` configuration option. | ||
82 | +* Remove `toDigits` method; extend `precision` method accordingly. | ||
83 | +* Remove s`round` method; extend `decimalPlaces` method accordingly. | ||
84 | +* Remove methods: `ceil`, `floor`, and `truncated`. | ||
85 | +* Remove method aliases: `add`, `cmp`, `isInt`, `isNeg`, `trunc`, `mul`, `neg` and `sub`. | ||
86 | +* Rename methods: `shift` to `shiftedBy`, `another` to `clone`, `toPower` to `exponentiatedBy`, and `equals` to `isEqualTo`. | ||
87 | +* Rename methods: add `is` prefix to `greaterThan`, `greaterThanOrEqualTo`, `lessThan` and `lessThanOrEqualTo`. | ||
88 | +* Add methods: `multipliedBy`, `isBigNumber`, `isPositive`, `integerValue`, `maximum` and `minimum`. | ||
89 | +* Refactor test suite. | ||
90 | +* Add *CHANGELOG.md*. | ||
91 | +* Rewrite *bignumber.d.ts*. | ||
92 | +* Redo API image. | ||
93 | + | ||
94 | +#### 5.0.0 | ||
95 | +* 27/11/2017 | ||
96 | +* #81 Don't throw on constructor call without `new`. | ||
97 | + | ||
98 | +#### 4.1.0 | ||
99 | +* 26/09/2017 | ||
100 | +* Remove node 0.6 from *.travis.yml*. | ||
101 | +* Add *bignumber.mjs*. | ||
102 | + | ||
103 | +#### 4.0.4 | ||
104 | +* 03/09/2017 | ||
105 | +* Add missing aliases to *bignumber.d.ts*. | ||
106 | + | ||
107 | +#### 4.0.3 | ||
108 | +* 30/08/2017 | ||
109 | +* Add types: *bignumber.d.ts*. | ||
110 | + | ||
111 | +#### 4.0.2 | ||
112 | +* 03/05/2017 | ||
113 | +* #120 Workaround Safari/Webkit bug. | ||
114 | + | ||
115 | +#### 4.0.1 | ||
116 | +* 05/04/2017 | ||
117 | +* #121 BigNumber.default to BigNumber['default']. | ||
118 | + | ||
119 | +#### 4.0.0 | ||
120 | +* 09/01/2017 | ||
121 | +* Replace BigNumber.isBigNumber method with isBigNumber prototype property. | ||
122 | + | ||
123 | +#### 3.1.2 | ||
124 | +* 08/01/2017 | ||
125 | +* Minor documentation edit. | ||
126 | + | ||
127 | +#### 3.1.1 | ||
128 | +* 08/01/2017 | ||
129 | +* Uncomment `isBigNumber` tests. | ||
130 | +* Ignore dot files. | ||
131 | + | ||
132 | +#### 3.1.0 | ||
133 | +* 08/01/2017 | ||
134 | +* Add `isBigNumber` method. | ||
135 | + | ||
136 | +#### 3.0.2 | ||
137 | +* 08/01/2017 | ||
138 | +* Bugfix: Possible incorrect value of `ERRORS` after a `BigNumber.another` call (due to `parseNumeric` declaration in outer scope). | ||
139 | + | ||
140 | +#### 3.0.1 | ||
141 | +* 23/11/2016 | ||
142 | +* Apply fix for old ipads with `%` issue, see #57 and #102. | ||
143 | +* Correct error message. | ||
144 | + | ||
145 | +#### 3.0.0 | ||
146 | +* 09/11/2016 | ||
147 | +* Remove `require('crypto')` - leave it to the user. | ||
148 | +* Add `BigNumber.set` as `BigNumber.config` alias. | ||
149 | +* Default `POW_PRECISION` to `0`. | ||
150 | + | ||
151 | +#### 2.4.0 | ||
152 | +* 14/07/2016 | ||
153 | +* #97 Add exports to support ES6 imports. | ||
154 | + | ||
155 | +#### 2.3.0 | ||
156 | +* 07/03/2016 | ||
157 | +* #86 Add modulus parameter to `toPower`. | ||
158 | + | ||
159 | +#### 2.2.0 | ||
160 | +* 03/03/2016 | ||
161 | +* #91 Permit larger JS integers. | ||
162 | + | ||
163 | +#### 2.1.4 | ||
164 | +* 15/12/2015 | ||
165 | +* Correct UMD. | ||
166 | + | ||
167 | +#### 2.1.3 | ||
168 | +* 13/12/2015 | ||
169 | +* Refactor re global object and crypto availability when bundling. | ||
170 | + | ||
171 | +#### 2.1.2 | ||
172 | +* 10/12/2015 | ||
173 | +* Bugfix: `window.crypto` not assigned to `crypto`. | ||
174 | + | ||
175 | +#### 2.1.1 | ||
176 | +* 09/12/2015 | ||
177 | +* Prevent code bundler from adding `crypto` shim. | ||
178 | + | ||
179 | +#### 2.1.0 | ||
180 | +* 26/10/2015 | ||
181 | +* For `valueOf` and `toJSON`, include the minus sign with negative zero. | ||
182 | + | ||
183 | +#### 2.0.8 | ||
184 | +* 2/10/2015 | ||
185 | +* Internal round function bugfix. | ||
186 | + | ||
187 | +#### 2.0.6 | ||
188 | +* 31/03/2015 | ||
189 | +* Add bower.json. Tweak division after in-depth review. | ||
190 | + | ||
191 | +#### 2.0.5 | ||
192 | +* 25/03/2015 | ||
193 | +* Amend README. Remove bitcoin address. | ||
194 | + | ||
195 | +#### 2.0.4 | ||
196 | +* 25/03/2015 | ||
197 | +* Critical bugfix #58: division. | ||
198 | + | ||
199 | +#### 2.0.3 | ||
200 | +* 18/02/2015 | ||
201 | +* Amend README. Add source map. | ||
202 | + | ||
203 | +#### 2.0.2 | ||
204 | +* 18/02/2015 | ||
205 | +* Correct links. | ||
206 | + | ||
207 | +#### 2.0.1 | ||
208 | +* 18/02/2015 | ||
209 | +* Add `max`, `min`, `precision`, `random`, `shiftedBy`, `toDigits` and `truncated` methods. | ||
210 | +* Add the short-forms: `add`, `mul`, `sd`, `sub` and `trunc`. | ||
211 | +* Add an `another` method to enable multiple independent constructors to be created. | ||
212 | +* Add support for the base 2, 8 and 16 prefixes `0b`, `0o` and `0x`. | ||
213 | +* Enable a rounding mode to be specified as a second parameter to `toExponential`, `toFixed`, `toFormat` and `toPrecision`. | ||
214 | +* Add a `CRYPTO` configuration property so cryptographically-secure pseudo-random number generation can be specified. | ||
215 | +* Add a `MODULO_MODE` configuration property to enable the rounding mode used by the `modulo` operation to be specified. | ||
216 | +* Add a `POW_PRECISION` configuration property to enable the number of significant digits calculated by the power operation to be limited. | ||
217 | +* Improve code quality. | ||
218 | +* Improve documentation. | ||
219 | + | ||
220 | +#### 2.0.0 | ||
221 | +* 29/12/2014 | ||
222 | +* Add `dividedToIntegerBy`, `isInteger` and `toFormat` methods. | ||
223 | +* Remove the following short-forms: `isF`, `isZ`, `toE`, `toF`, `toFr`, `toN`, `toP`, `toS`. | ||
224 | +* Store a BigNumber's coefficient in base 1e14, rather than base 10. | ||
225 | +* Add fast path for integers to BigNumber constructor. | ||
226 | +* Incorporate the library into the online documentation. | ||
227 | + | ||
228 | +#### 1.5.0 | ||
229 | +* 13/11/2014 | ||
230 | +* Add `toJSON` and `decimalPlaces` methods. | ||
231 | + | ||
232 | +#### 1.4.1 | ||
233 | +* 08/06/2014 | ||
234 | +* Amend README. | ||
235 | + | ||
236 | +#### 1.4.0 | ||
237 | +* 08/05/2014 | ||
238 | +* Add `toNumber`. | ||
239 | + | ||
240 | +#### 1.3.0 | ||
241 | +* 08/11/2013 | ||
242 | +* Ensure correct rounding of `sqrt` in all, rather than almost all, cases. | ||
243 | +* Maximum radix to 64. | ||
244 | + | ||
245 | +#### 1.2.1 | ||
246 | +* 17/10/2013 | ||
247 | +* Sign of zero when x < 0 and x + (-x) = 0. | ||
248 | + | ||
249 | +#### 1.2.0 | ||
250 | +* 19/9/2013 | ||
251 | +* Throw Error objects for stack. | ||
252 | + | ||
253 | +#### 1.1.1 | ||
254 | +* 22/8/2013 | ||
255 | +* Show original value in constructor error message. | ||
256 | + | ||
257 | +#### 1.1.0 | ||
258 | +* 1/8/2013 | ||
259 | +* Allow numbers with trailing radix point. | ||
260 | + | ||
261 | +#### 1.0.1 | ||
262 | +* Bugfix: error messages with incorrect method name | ||
263 | + | ||
264 | +#### 1.0.0 | ||
265 | +* 8/11/2012 | ||
266 | +* Initial release |
node_modules/bignumber.js/LICENCE
0 → 100644
1 | +The MIT Licence. | ||
2 | + | ||
3 | +Copyright (c) 2019 Michael Mclaughlin | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining | ||
6 | +a copy of this software and associated documentation files (the | ||
7 | +'Software'), to deal in the Software without restriction, including | ||
8 | +without limitation the rights to use, copy, modify, merge, publish, | ||
9 | +distribute, sublicense, and/or sell copies of the Software, and to | ||
10 | +permit persons to whom the Software is furnished to do so, subject to | ||
11 | +the following conditions: | ||
12 | + | ||
13 | +The above copyright notice and this permission notice shall be | ||
14 | +included in all copies or substantial portions of the Software. | ||
15 | + | ||
16 | +THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, | ||
17 | +EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF | ||
18 | +MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. | ||
19 | +IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY | ||
20 | +CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, | ||
21 | +TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE | ||
22 | +SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
23 | + |
node_modules/bignumber.js/README.md
0 → 100644
1 | + | ||
2 | + | ||
3 | +A JavaScript library for arbitrary-precision decimal and non-decimal arithmetic. | ||
4 | + | ||
5 | +[](https://travis-ci.org/MikeMcl/bignumber.js) | ||
6 | + | ||
7 | +<br /> | ||
8 | + | ||
9 | +## Features | ||
10 | + | ||
11 | + - Integers and decimals | ||
12 | + - Simple API but full-featured | ||
13 | + - Faster, smaller, and perhaps easier to use than JavaScript versions of Java's BigDecimal | ||
14 | + - 8 KB minified and gzipped | ||
15 | + - Replicates the `toExponential`, `toFixed`, `toPrecision` and `toString` methods of JavaScript's Number type | ||
16 | + - Includes a `toFraction` and a correctly-rounded `squareRoot` method | ||
17 | + - Supports cryptographically-secure pseudo-random number generation | ||
18 | + - No dependencies | ||
19 | + - Wide platform compatibility: uses JavaScript 1.5 (ECMAScript 3) features only | ||
20 | + - Comprehensive [documentation](http://mikemcl.github.io/bignumber.js/) and test set | ||
21 | + | ||
22 | +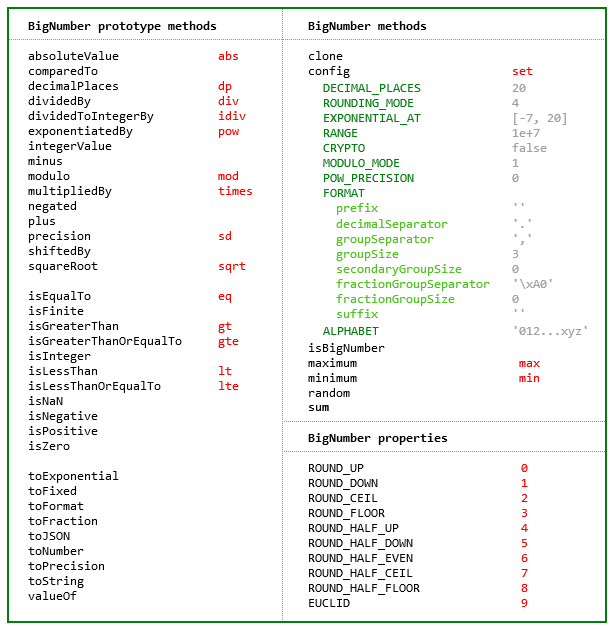 | ||
23 | + | ||
24 | +If a smaller and simpler library is required see [big.js](https://github.com/MikeMcl/big.js/). | ||
25 | +It's less than half the size but only works with decimal numbers and only has half the methods. | ||
26 | +It also does not allow `NaN` or `Infinity`, or have the configuration options of this library. | ||
27 | + | ||
28 | +See also [decimal.js](https://github.com/MikeMcl/decimal.js/), which among other things adds support for non-integer powers, and performs all operations to a specified number of significant digits. | ||
29 | + | ||
30 | +## Load | ||
31 | + | ||
32 | +The library is the single JavaScript file *bignumber.js* (or minified, *bignumber.min.js*). | ||
33 | + | ||
34 | +Browser: | ||
35 | + | ||
36 | +```html | ||
37 | +<script src='path/to/bignumber.js'></script> | ||
38 | +``` | ||
39 | + | ||
40 | +[Node.js](http://nodejs.org): | ||
41 | + | ||
42 | +```bash | ||
43 | +$ npm install bignumber.js | ||
44 | +``` | ||
45 | + | ||
46 | +```javascript | ||
47 | +const BigNumber = require('bignumber.js'); | ||
48 | +``` | ||
49 | + | ||
50 | +ES6 module: | ||
51 | + | ||
52 | +```javascript | ||
53 | +import BigNumber from "./bignumber.mjs" | ||
54 | +``` | ||
55 | + | ||
56 | +AMD loader libraries such as [requireJS](http://requirejs.org/): | ||
57 | + | ||
58 | +```javascript | ||
59 | +require(['bignumber'], function(BigNumber) { | ||
60 | + // Use BigNumber here in local scope. No global BigNumber. | ||
61 | +}); | ||
62 | +``` | ||
63 | + | ||
64 | +## Use | ||
65 | + | ||
66 | +The library exports a single constructor function, [`BigNumber`](http://mikemcl.github.io/bignumber.js/#bignumber), which accepts a value of type Number, String or BigNumber, | ||
67 | + | ||
68 | +```javascript | ||
69 | +let x = new BigNumber(123.4567); | ||
70 | +let y = BigNumber('123456.7e-3'); | ||
71 | +let z = new BigNumber(x); | ||
72 | +x.isEqualTo(y) && y.isEqualTo(z) && x.isEqualTo(z); // true | ||
73 | +``` | ||
74 | + | ||
75 | +To get the string value of a BigNumber use [`toString()`](http://mikemcl.github.io/bignumber.js/#toS) or [`toFixed()`](http://mikemcl.github.io/bignumber.js/#toFix). Using `toFixed()` prevents exponential notation being returned, no matter how large or small the value. | ||
76 | + | ||
77 | +```javascript | ||
78 | +let x = new BigNumber('1111222233334444555566'); | ||
79 | +x.toString(); // "1.111222233334444555566e+21" | ||
80 | +x.toFixed(); // "1111222233334444555566" | ||
81 | +``` | ||
82 | + | ||
83 | +If the limited precision of Number values is not well understood, it is recommended to create BigNumbers from String values rather than Number values to avoid a potential loss of precision. | ||
84 | + | ||
85 | +*In all further examples below, `let`, semicolons and `toString` calls are not shown. If a commented-out value is in quotes it means `toString` has been called on the preceding expression.* | ||
86 | + | ||
87 | +```javascript | ||
88 | +// Precision loss from using numeric literals with more than 15 significant digits. | ||
89 | +new BigNumber(1.0000000000000001) // '1' | ||
90 | +new BigNumber(88259496234518.57) // '88259496234518.56' | ||
91 | +new BigNumber(99999999999999999999) // '100000000000000000000' | ||
92 | + | ||
93 | +// Precision loss from using numeric literals outside the range of Number values. | ||
94 | +new BigNumber(2e+308) // 'Infinity' | ||
95 | +new BigNumber(1e-324) // '0' | ||
96 | + | ||
97 | +// Precision loss from the unexpected result of arithmetic with Number values. | ||
98 | +new BigNumber(0.7 + 0.1) // '0.7999999999999999' | ||
99 | +``` | ||
100 | + | ||
101 | +When creating a BigNumber from a Number, note that a BigNumber is created from a Number's decimal `toString()` value not from its underlying binary value. If the latter is required, then pass the Number's `toString(2)` value and specify base 2. | ||
102 | + | ||
103 | +```javascript | ||
104 | +new BigNumber(Number.MAX_VALUE.toString(2), 2) | ||
105 | +``` | ||
106 | + | ||
107 | +BigNumbers can be created from values in bases from 2 to 36. See [`ALPHABET`](http://mikemcl.github.io/bignumber.js/#alphabet) to extend this range. | ||
108 | + | ||
109 | +```javascript | ||
110 | +a = new BigNumber(1011, 2) // "11" | ||
111 | +b = new BigNumber('zz.9', 36) // "1295.25" | ||
112 | +c = a.plus(b) // "1306.25" | ||
113 | +``` | ||
114 | + | ||
115 | +Performance is better if base 10 is NOT specified for decimal values. Only specify base 10 when it is desired that the number of decimal places of the input value be limited to the current [`DECIMAL_PLACES`](http://mikemcl.github.io/bignumber.js/#decimal-places) setting. | ||
116 | + | ||
117 | +A BigNumber is immutable in the sense that it is not changed by its methods. | ||
118 | + | ||
119 | +```javascript | ||
120 | +0.3 - 0.1 // 0.19999999999999998 | ||
121 | +x = new BigNumber(0.3) | ||
122 | +x.minus(0.1) // "0.2" | ||
123 | +x // "0.3" | ||
124 | +``` | ||
125 | + | ||
126 | +The methods that return a BigNumber can be chained. | ||
127 | + | ||
128 | +```javascript | ||
129 | +x.dividedBy(y).plus(z).times(9) | ||
130 | +x.times('1.23456780123456789e+9').plus(9876.5432321).dividedBy('4444562598.111772').integerValue() | ||
131 | +``` | ||
132 | + | ||
133 | +Some of the longer method names have a shorter alias. | ||
134 | + | ||
135 | +```javascript | ||
136 | +x.squareRoot().dividedBy(y).exponentiatedBy(3).isEqualTo(x.sqrt().div(y).pow(3)) // true | ||
137 | +x.modulo(y).multipliedBy(z).eq(x.mod(y).times(z)) // true | ||
138 | +``` | ||
139 | + | ||
140 | +As with JavaScript's Number type, there are [`toExponential`](http://mikemcl.github.io/bignumber.js/#toE), [`toFixed`](http://mikemcl.github.io/bignumber.js/#toFix) and [`toPrecision`](http://mikemcl.github.io/bignumber.js/#toP) methods. | ||
141 | + | ||
142 | +```javascript | ||
143 | +x = new BigNumber(255.5) | ||
144 | +x.toExponential(5) // "2.55500e+2" | ||
145 | +x.toFixed(5) // "255.50000" | ||
146 | +x.toPrecision(5) // "255.50" | ||
147 | +x.toNumber() // 255.5 | ||
148 | +``` | ||
149 | + | ||
150 | + A base can be specified for [`toString`](http://mikemcl.github.io/bignumber.js/#toS). Performance is better if base 10 is NOT specified, i.e. use `toString()` not `toString(10)`. Only specify base 10 when it is desired that the number of decimal places be limited to the current [`DECIMAL_PLACES`](http://mikemcl.github.io/bignumber.js/#decimal-places) setting. | ||
151 | + | ||
152 | + ```javascript | ||
153 | + x.toString(16) // "ff.8" | ||
154 | + ``` | ||
155 | + | ||
156 | +There is a [`toFormat`](http://mikemcl.github.io/bignumber.js/#toFor) method which may be useful for internationalisation. | ||
157 | + | ||
158 | +```javascript | ||
159 | +y = new BigNumber('1234567.898765') | ||
160 | +y.toFormat(2) // "1,234,567.90" | ||
161 | +``` | ||
162 | + | ||
163 | +The maximum number of decimal places of the result of an operation involving division (i.e. a division, square root, base conversion or negative power operation) is set using the `set` or `config` method of the `BigNumber` constructor. | ||
164 | + | ||
165 | +The other arithmetic operations always give the exact result. | ||
166 | + | ||
167 | +```javascript | ||
168 | +BigNumber.set({ DECIMAL_PLACES: 10, ROUNDING_MODE: 4 }) | ||
169 | + | ||
170 | +x = new BigNumber(2) | ||
171 | +y = new BigNumber(3) | ||
172 | +z = x.dividedBy(y) // "0.6666666667" | ||
173 | +z.squareRoot() // "0.8164965809" | ||
174 | +z.exponentiatedBy(-3) // "3.3749999995" | ||
175 | +z.toString(2) // "0.1010101011" | ||
176 | +z.multipliedBy(z) // "0.44444444448888888889" | ||
177 | +z.multipliedBy(z).decimalPlaces(10) // "0.4444444445" | ||
178 | +``` | ||
179 | + | ||
180 | +There is a [`toFraction`](http://mikemcl.github.io/bignumber.js/#toFr) method with an optional *maximum denominator* argument | ||
181 | + | ||
182 | +```javascript | ||
183 | +y = new BigNumber(355) | ||
184 | +pi = y.dividedBy(113) // "3.1415929204" | ||
185 | +pi.toFraction() // [ "7853982301", "2500000000" ] | ||
186 | +pi.toFraction(1000) // [ "355", "113" ] | ||
187 | +``` | ||
188 | + | ||
189 | +and [`isNaN`](http://mikemcl.github.io/bignumber.js/#isNaN) and [`isFinite`](http://mikemcl.github.io/bignumber.js/#isF) methods, as `NaN` and `Infinity` are valid `BigNumber` values. | ||
190 | + | ||
191 | +```javascript | ||
192 | +x = new BigNumber(NaN) // "NaN" | ||
193 | +y = new BigNumber(Infinity) // "Infinity" | ||
194 | +x.isNaN() && !y.isNaN() && !x.isFinite() && !y.isFinite() // true | ||
195 | +``` | ||
196 | + | ||
197 | +The value of a BigNumber is stored in a decimal floating point format in terms of a coefficient, exponent and sign. | ||
198 | + | ||
199 | +```javascript | ||
200 | +x = new BigNumber(-123.456); | ||
201 | +x.c // [ 123, 45600000000000 ] coefficient (i.e. significand) | ||
202 | +x.e // 2 exponent | ||
203 | +x.s // -1 sign | ||
204 | +``` | ||
205 | + | ||
206 | +For advanced usage, multiple BigNumber constructors can be created, each with their own independent configuration. | ||
207 | + | ||
208 | +```javascript | ||
209 | +// Set DECIMAL_PLACES for the original BigNumber constructor | ||
210 | +BigNumber.set({ DECIMAL_PLACES: 10 }) | ||
211 | + | ||
212 | +// Create another BigNumber constructor, optionally passing in a configuration object | ||
213 | +BN = BigNumber.clone({ DECIMAL_PLACES: 5 }) | ||
214 | + | ||
215 | +x = new BigNumber(1) | ||
216 | +y = new BN(1) | ||
217 | + | ||
218 | +x.div(3) // '0.3333333333' | ||
219 | +y.div(3) // '0.33333' | ||
220 | +``` | ||
221 | + | ||
222 | +For further information see the [API](http://mikemcl.github.io/bignumber.js/) reference in the *doc* directory. | ||
223 | + | ||
224 | +## Test | ||
225 | + | ||
226 | +The *test/modules* directory contains the test scripts for each method. | ||
227 | + | ||
228 | +The tests can be run with Node.js or a browser. For Node.js use | ||
229 | + | ||
230 | + $ npm test | ||
231 | + | ||
232 | +or | ||
233 | + | ||
234 | + $ node test/test | ||
235 | + | ||
236 | +To test a single method, use, for example | ||
237 | + | ||
238 | + $ node test/methods/toFraction | ||
239 | + | ||
240 | +For the browser, open *test/test.html*. | ||
241 | + | ||
242 | +## Build | ||
243 | + | ||
244 | +For Node, if [uglify-js](https://github.com/mishoo/UglifyJS2) is installed | ||
245 | + | ||
246 | + npm install uglify-js -g | ||
247 | + | ||
248 | +then | ||
249 | + | ||
250 | + npm run build | ||
251 | + | ||
252 | +will create *bignumber.min.js*. | ||
253 | + | ||
254 | +A source map will also be created in the root directory. | ||
255 | + | ||
256 | +## Feedback | ||
257 | + | ||
258 | +Open an issue, or email | ||
259 | + | ||
260 | +Michael | ||
261 | + | ||
262 | +<a href="mailto:M8ch88l@gmail.com">M8ch88l@gmail.com</a> | ||
263 | + | ||
264 | +## Licence | ||
265 | + | ||
266 | +The MIT Licence. | ||
267 | + | ||
268 | +See [LICENCE](https://github.com/MikeMcl/bignumber.js/blob/master/LICENCE). |
node_modules/bignumber.js/bignumber.d.ts
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/bignumber.js/bignumber.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/bignumber.js/bignumber.min.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/bignumber.js/bignumber.mjs
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/bignumber.js/doc/API.html
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/bignumber.js/package.json
0 → 100644
1 | +{ | ||
2 | + "_from": "bignumber.js@9.0.0", | ||
3 | + "_id": "bignumber.js@9.0.0", | ||
4 | + "_inBundle": false, | ||
5 | + "_integrity": "sha512-t/OYhhJ2SD+YGBQcjY8GzzDHEk9f3nerxjtfa6tlMXfe7frs/WozhvCNoGvpM0P3bNf3Gq5ZRMlGr5f3r4/N8A==", | ||
6 | + "_location": "/bignumber.js", | ||
7 | + "_phantomChildren": {}, | ||
8 | + "_requested": { | ||
9 | + "type": "version", | ||
10 | + "registry": true, | ||
11 | + "raw": "bignumber.js@9.0.0", | ||
12 | + "name": "bignumber.js", | ||
13 | + "escapedName": "bignumber.js", | ||
14 | + "rawSpec": "9.0.0", | ||
15 | + "saveSpec": null, | ||
16 | + "fetchSpec": "9.0.0" | ||
17 | + }, | ||
18 | + "_requiredBy": [ | ||
19 | + "/mysql" | ||
20 | + ], | ||
21 | + "_resolved": "https://registry.npmjs.org/bignumber.js/-/bignumber.js-9.0.0.tgz", | ||
22 | + "_shasum": "805880f84a329b5eac6e7cb6f8274b6d82bdf075", | ||
23 | + "_spec": "bignumber.js@9.0.0", | ||
24 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사\\node_modules\\mysql", | ||
25 | + "author": { | ||
26 | + "name": "Michael Mclaughlin", | ||
27 | + "email": "M8ch88l@gmail.com" | ||
28 | + }, | ||
29 | + "browser": "bignumber.js", | ||
30 | + "bugs": { | ||
31 | + "url": "https://github.com/MikeMcl/bignumber.js/issues" | ||
32 | + }, | ||
33 | + "bundleDependencies": false, | ||
34 | + "dependencies": {}, | ||
35 | + "deprecated": false, | ||
36 | + "description": "A library for arbitrary-precision decimal and non-decimal arithmetic", | ||
37 | + "engines": { | ||
38 | + "node": "*" | ||
39 | + }, | ||
40 | + "homepage": "https://github.com/MikeMcl/bignumber.js#readme", | ||
41 | + "keywords": [ | ||
42 | + "arbitrary", | ||
43 | + "precision", | ||
44 | + "arithmetic", | ||
45 | + "big", | ||
46 | + "number", | ||
47 | + "decimal", | ||
48 | + "float", | ||
49 | + "biginteger", | ||
50 | + "bigdecimal", | ||
51 | + "bignumber", | ||
52 | + "bigint", | ||
53 | + "bignum" | ||
54 | + ], | ||
55 | + "license": "MIT", | ||
56 | + "main": "bignumber", | ||
57 | + "module": "bignumber.mjs", | ||
58 | + "name": "bignumber.js", | ||
59 | + "repository": { | ||
60 | + "type": "git", | ||
61 | + "url": "git+https://github.com/MikeMcl/bignumber.js.git" | ||
62 | + }, | ||
63 | + "scripts": { | ||
64 | + "build": "uglifyjs bignumber.js --source-map -c -m -o bignumber.min.js", | ||
65 | + "test": "node test/test" | ||
66 | + }, | ||
67 | + "types": "bignumber.d.ts", | ||
68 | + "version": "9.0.0" | ||
69 | +} |
node_modules/brace-expansion/LICENSE
0 → 100644
1 | +MIT License | ||
2 | + | ||
3 | +Copyright (c) 2013 Julian Gruber <julian@juliangruber.com> | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
6 | +of this software and associated documentation files (the "Software"), to deal | ||
7 | +in the Software without restriction, including without limitation the rights | ||
8 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
9 | +copies of the Software, and to permit persons to whom the Software is | ||
10 | +furnished to do so, subject to the following conditions: | ||
11 | + | ||
12 | +The above copyright notice and this permission notice shall be included in all | ||
13 | +copies or substantial portions of the Software. | ||
14 | + | ||
15 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
16 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
17 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
18 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
19 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
20 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
21 | +SOFTWARE. |
node_modules/brace-expansion/README.md
0 → 100644
1 | +# brace-expansion | ||
2 | + | ||
3 | +[Brace expansion](https://www.gnu.org/software/bash/manual/html_node/Brace-Expansion.html), | ||
4 | +as known from sh/bash, in JavaScript. | ||
5 | + | ||
6 | +[](http://travis-ci.org/juliangruber/brace-expansion) | ||
7 | +[](https://www.npmjs.org/package/brace-expansion) | ||
8 | +[](https://greenkeeper.io/) | ||
9 | + | ||
10 | +[](https://ci.testling.com/juliangruber/brace-expansion) | ||
11 | + | ||
12 | +## Example | ||
13 | + | ||
14 | +```js | ||
15 | +var expand = require('brace-expansion'); | ||
16 | + | ||
17 | +expand('file-{a,b,c}.jpg') | ||
18 | +// => ['file-a.jpg', 'file-b.jpg', 'file-c.jpg'] | ||
19 | + | ||
20 | +expand('-v{,,}') | ||
21 | +// => ['-v', '-v', '-v'] | ||
22 | + | ||
23 | +expand('file{0..2}.jpg') | ||
24 | +// => ['file0.jpg', 'file1.jpg', 'file2.jpg'] | ||
25 | + | ||
26 | +expand('file-{a..c}.jpg') | ||
27 | +// => ['file-a.jpg', 'file-b.jpg', 'file-c.jpg'] | ||
28 | + | ||
29 | +expand('file{2..0}.jpg') | ||
30 | +// => ['file2.jpg', 'file1.jpg', 'file0.jpg'] | ||
31 | + | ||
32 | +expand('file{0..4..2}.jpg') | ||
33 | +// => ['file0.jpg', 'file2.jpg', 'file4.jpg'] | ||
34 | + | ||
35 | +expand('file-{a..e..2}.jpg') | ||
36 | +// => ['file-a.jpg', 'file-c.jpg', 'file-e.jpg'] | ||
37 | + | ||
38 | +expand('file{00..10..5}.jpg') | ||
39 | +// => ['file00.jpg', 'file05.jpg', 'file10.jpg'] | ||
40 | + | ||
41 | +expand('{{A..C},{a..c}}') | ||
42 | +// => ['A', 'B', 'C', 'a', 'b', 'c'] | ||
43 | + | ||
44 | +expand('ppp{,config,oe{,conf}}') | ||
45 | +// => ['ppp', 'pppconfig', 'pppoe', 'pppoeconf'] | ||
46 | +``` | ||
47 | + | ||
48 | +## API | ||
49 | + | ||
50 | +```js | ||
51 | +var expand = require('brace-expansion'); | ||
52 | +``` | ||
53 | + | ||
54 | +### var expanded = expand(str) | ||
55 | + | ||
56 | +Return an array of all possible and valid expansions of `str`. If none are | ||
57 | +found, `[str]` is returned. | ||
58 | + | ||
59 | +Valid expansions are: | ||
60 | + | ||
61 | +```js | ||
62 | +/^(.*,)+(.+)?$/ | ||
63 | +// {a,b,...} | ||
64 | +``` | ||
65 | + | ||
66 | +A comma separated list of options, like `{a,b}` or `{a,{b,c}}` or `{,a,}`. | ||
67 | + | ||
68 | +```js | ||
69 | +/^-?\d+\.\.-?\d+(\.\.-?\d+)?$/ | ||
70 | +// {x..y[..incr]} | ||
71 | +``` | ||
72 | + | ||
73 | +A numeric sequence from `x` to `y` inclusive, with optional increment. | ||
74 | +If `x` or `y` start with a leading `0`, all the numbers will be padded | ||
75 | +to have equal length. Negative numbers and backwards iteration work too. | ||
76 | + | ||
77 | +```js | ||
78 | +/^-?\d+\.\.-?\d+(\.\.-?\d+)?$/ | ||
79 | +// {x..y[..incr]} | ||
80 | +``` | ||
81 | + | ||
82 | +An alphabetic sequence from `x` to `y` inclusive, with optional increment. | ||
83 | +`x` and `y` must be exactly one character, and if given, `incr` must be a | ||
84 | +number. | ||
85 | + | ||
86 | +For compatibility reasons, the string `${` is not eligible for brace expansion. | ||
87 | + | ||
88 | +## Installation | ||
89 | + | ||
90 | +With [npm](https://npmjs.org) do: | ||
91 | + | ||
92 | +```bash | ||
93 | +npm install brace-expansion | ||
94 | +``` | ||
95 | + | ||
96 | +## Contributors | ||
97 | + | ||
98 | +- [Julian Gruber](https://github.com/juliangruber) | ||
99 | +- [Isaac Z. Schlueter](https://github.com/isaacs) | ||
100 | + | ||
101 | +## Sponsors | ||
102 | + | ||
103 | +This module is proudly supported by my [Sponsors](https://github.com/juliangruber/sponsors)! | ||
104 | + | ||
105 | +Do you want to support modules like this to improve their quality, stability and weigh in on new features? Then please consider donating to my [Patreon](https://www.patreon.com/juliangruber). Not sure how much of my modules you're using? Try [feross/thanks](https://github.com/feross/thanks)! | ||
106 | + | ||
107 | +## License | ||
108 | + | ||
109 | +(MIT) | ||
110 | + | ||
111 | +Copyright (c) 2013 Julian Gruber <julian@juliangruber.com> | ||
112 | + | ||
113 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
114 | +this software and associated documentation files (the "Software"), to deal in | ||
115 | +the Software without restriction, including without limitation the rights to | ||
116 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies | ||
117 | +of the Software, and to permit persons to whom the Software is furnished to do | ||
118 | +so, subject to the following conditions: | ||
119 | + | ||
120 | +The above copyright notice and this permission notice shall be included in all | ||
121 | +copies or substantial portions of the Software. | ||
122 | + | ||
123 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
124 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
125 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
126 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
127 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
128 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
129 | +SOFTWARE. |
node_modules/brace-expansion/index.js
0 → 100644
1 | +var concatMap = require('concat-map'); | ||
2 | +var balanced = require('balanced-match'); | ||
3 | + | ||
4 | +module.exports = expandTop; | ||
5 | + | ||
6 | +var escSlash = '\0SLASH'+Math.random()+'\0'; | ||
7 | +var escOpen = '\0OPEN'+Math.random()+'\0'; | ||
8 | +var escClose = '\0CLOSE'+Math.random()+'\0'; | ||
9 | +var escComma = '\0COMMA'+Math.random()+'\0'; | ||
10 | +var escPeriod = '\0PERIOD'+Math.random()+'\0'; | ||
11 | + | ||
12 | +function numeric(str) { | ||
13 | + return parseInt(str, 10) == str | ||
14 | + ? parseInt(str, 10) | ||
15 | + : str.charCodeAt(0); | ||
16 | +} | ||
17 | + | ||
18 | +function escapeBraces(str) { | ||
19 | + return str.split('\\\\').join(escSlash) | ||
20 | + .split('\\{').join(escOpen) | ||
21 | + .split('\\}').join(escClose) | ||
22 | + .split('\\,').join(escComma) | ||
23 | + .split('\\.').join(escPeriod); | ||
24 | +} | ||
25 | + | ||
26 | +function unescapeBraces(str) { | ||
27 | + return str.split(escSlash).join('\\') | ||
28 | + .split(escOpen).join('{') | ||
29 | + .split(escClose).join('}') | ||
30 | + .split(escComma).join(',') | ||
31 | + .split(escPeriod).join('.'); | ||
32 | +} | ||
33 | + | ||
34 | + | ||
35 | +// Basically just str.split(","), but handling cases | ||
36 | +// where we have nested braced sections, which should be | ||
37 | +// treated as individual members, like {a,{b,c},d} | ||
38 | +function parseCommaParts(str) { | ||
39 | + if (!str) | ||
40 | + return ['']; | ||
41 | + | ||
42 | + var parts = []; | ||
43 | + var m = balanced('{', '}', str); | ||
44 | + | ||
45 | + if (!m) | ||
46 | + return str.split(','); | ||
47 | + | ||
48 | + var pre = m.pre; | ||
49 | + var body = m.body; | ||
50 | + var post = m.post; | ||
51 | + var p = pre.split(','); | ||
52 | + | ||
53 | + p[p.length-1] += '{' + body + '}'; | ||
54 | + var postParts = parseCommaParts(post); | ||
55 | + if (post.length) { | ||
56 | + p[p.length-1] += postParts.shift(); | ||
57 | + p.push.apply(p, postParts); | ||
58 | + } | ||
59 | + | ||
60 | + parts.push.apply(parts, p); | ||
61 | + | ||
62 | + return parts; | ||
63 | +} | ||
64 | + | ||
65 | +function expandTop(str) { | ||
66 | + if (!str) | ||
67 | + return []; | ||
68 | + | ||
69 | + // I don't know why Bash 4.3 does this, but it does. | ||
70 | + // Anything starting with {} will have the first two bytes preserved | ||
71 | + // but *only* at the top level, so {},a}b will not expand to anything, | ||
72 | + // but a{},b}c will be expanded to [a}c,abc]. | ||
73 | + // One could argue that this is a bug in Bash, but since the goal of | ||
74 | + // this module is to match Bash's rules, we escape a leading {} | ||
75 | + if (str.substr(0, 2) === '{}') { | ||
76 | + str = '\\{\\}' + str.substr(2); | ||
77 | + } | ||
78 | + | ||
79 | + return expand(escapeBraces(str), true).map(unescapeBraces); | ||
80 | +} | ||
81 | + | ||
82 | +function identity(e) { | ||
83 | + return e; | ||
84 | +} | ||
85 | + | ||
86 | +function embrace(str) { | ||
87 | + return '{' + str + '}'; | ||
88 | +} | ||
89 | +function isPadded(el) { | ||
90 | + return /^-?0\d/.test(el); | ||
91 | +} | ||
92 | + | ||
93 | +function lte(i, y) { | ||
94 | + return i <= y; | ||
95 | +} | ||
96 | +function gte(i, y) { | ||
97 | + return i >= y; | ||
98 | +} | ||
99 | + | ||
100 | +function expand(str, isTop) { | ||
101 | + var expansions = []; | ||
102 | + | ||
103 | + var m = balanced('{', '}', str); | ||
104 | + if (!m || /\$$/.test(m.pre)) return [str]; | ||
105 | + | ||
106 | + var isNumericSequence = /^-?\d+\.\.-?\d+(?:\.\.-?\d+)?$/.test(m.body); | ||
107 | + var isAlphaSequence = /^[a-zA-Z]\.\.[a-zA-Z](?:\.\.-?\d+)?$/.test(m.body); | ||
108 | + var isSequence = isNumericSequence || isAlphaSequence; | ||
109 | + var isOptions = m.body.indexOf(',') >= 0; | ||
110 | + if (!isSequence && !isOptions) { | ||
111 | + // {a},b} | ||
112 | + if (m.post.match(/,.*\}/)) { | ||
113 | + str = m.pre + '{' + m.body + escClose + m.post; | ||
114 | + return expand(str); | ||
115 | + } | ||
116 | + return [str]; | ||
117 | + } | ||
118 | + | ||
119 | + var n; | ||
120 | + if (isSequence) { | ||
121 | + n = m.body.split(/\.\./); | ||
122 | + } else { | ||
123 | + n = parseCommaParts(m.body); | ||
124 | + if (n.length === 1) { | ||
125 | + // x{{a,b}}y ==> x{a}y x{b}y | ||
126 | + n = expand(n[0], false).map(embrace); | ||
127 | + if (n.length === 1) { | ||
128 | + var post = m.post.length | ||
129 | + ? expand(m.post, false) | ||
130 | + : ['']; | ||
131 | + return post.map(function(p) { | ||
132 | + return m.pre + n[0] + p; | ||
133 | + }); | ||
134 | + } | ||
135 | + } | ||
136 | + } | ||
137 | + | ||
138 | + // at this point, n is the parts, and we know it's not a comma set | ||
139 | + // with a single entry. | ||
140 | + | ||
141 | + // no need to expand pre, since it is guaranteed to be free of brace-sets | ||
142 | + var pre = m.pre; | ||
143 | + var post = m.post.length | ||
144 | + ? expand(m.post, false) | ||
145 | + : ['']; | ||
146 | + | ||
147 | + var N; | ||
148 | + | ||
149 | + if (isSequence) { | ||
150 | + var x = numeric(n[0]); | ||
151 | + var y = numeric(n[1]); | ||
152 | + var width = Math.max(n[0].length, n[1].length) | ||
153 | + var incr = n.length == 3 | ||
154 | + ? Math.abs(numeric(n[2])) | ||
155 | + : 1; | ||
156 | + var test = lte; | ||
157 | + var reverse = y < x; | ||
158 | + if (reverse) { | ||
159 | + incr *= -1; | ||
160 | + test = gte; | ||
161 | + } | ||
162 | + var pad = n.some(isPadded); | ||
163 | + | ||
164 | + N = []; | ||
165 | + | ||
166 | + for (var i = x; test(i, y); i += incr) { | ||
167 | + var c; | ||
168 | + if (isAlphaSequence) { | ||
169 | + c = String.fromCharCode(i); | ||
170 | + if (c === '\\') | ||
171 | + c = ''; | ||
172 | + } else { | ||
173 | + c = String(i); | ||
174 | + if (pad) { | ||
175 | + var need = width - c.length; | ||
176 | + if (need > 0) { | ||
177 | + var z = new Array(need + 1).join('0'); | ||
178 | + if (i < 0) | ||
179 | + c = '-' + z + c.slice(1); | ||
180 | + else | ||
181 | + c = z + c; | ||
182 | + } | ||
183 | + } | ||
184 | + } | ||
185 | + N.push(c); | ||
186 | + } | ||
187 | + } else { | ||
188 | + N = concatMap(n, function(el) { return expand(el, false) }); | ||
189 | + } | ||
190 | + | ||
191 | + for (var j = 0; j < N.length; j++) { | ||
192 | + for (var k = 0; k < post.length; k++) { | ||
193 | + var expansion = pre + N[j] + post[k]; | ||
194 | + if (!isTop || isSequence || expansion) | ||
195 | + expansions.push(expansion); | ||
196 | + } | ||
197 | + } | ||
198 | + | ||
199 | + return expansions; | ||
200 | +} | ||
201 | + |
node_modules/brace-expansion/package.json
0 → 100644
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "brace-expansion@1.1.11", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "brace-expansion@1.1.11", | ||
9 | + "_id": "brace-expansion@1.1.11", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha512-iCuPHDFgrHX7H2vEI/5xpz07zSHB00TpugqhmYtVmMO6518mCuRMoOYFldEBl0g187ufozdaHgWKcYFb61qGiA==", | ||
12 | + "_location": "/brace-expansion", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "brace-expansion@1.1.11", | ||
18 | + "name": "brace-expansion", | ||
19 | + "escapedName": "brace-expansion", | ||
20 | + "rawSpec": "1.1.11", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "1.1.11" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/minimatch" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-1.1.11.tgz", | ||
28 | + "_spec": "1.1.11", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "author": { | ||
31 | + "name": "Julian Gruber", | ||
32 | + "email": "mail@juliangruber.com", | ||
33 | + "url": "http://juliangruber.com" | ||
34 | + }, | ||
35 | + "bugs": { | ||
36 | + "url": "https://github.com/juliangruber/brace-expansion/issues" | ||
37 | + }, | ||
38 | + "dependencies": { | ||
39 | + "balanced-match": "^1.0.0", | ||
40 | + "concat-map": "0.0.1" | ||
41 | + }, | ||
42 | + "description": "Brace expansion as known from sh/bash", | ||
43 | + "devDependencies": { | ||
44 | + "matcha": "^0.7.0", | ||
45 | + "tape": "^4.6.0" | ||
46 | + }, | ||
47 | + "homepage": "https://github.com/juliangruber/brace-expansion", | ||
48 | + "keywords": [], | ||
49 | + "license": "MIT", | ||
50 | + "main": "index.js", | ||
51 | + "name": "brace-expansion", | ||
52 | + "repository": { | ||
53 | + "type": "git", | ||
54 | + "url": "git://github.com/juliangruber/brace-expansion.git" | ||
55 | + }, | ||
56 | + "scripts": { | ||
57 | + "bench": "matcha test/perf/bench.js", | ||
58 | + "gentest": "bash test/generate.sh", | ||
59 | + "test": "tape test/*.js" | ||
60 | + }, | ||
61 | + "testling": { | ||
62 | + "files": "test/*.js", | ||
63 | + "browsers": [ | ||
64 | + "ie/8..latest", | ||
65 | + "firefox/20..latest", | ||
66 | + "firefox/nightly", | ||
67 | + "chrome/25..latest", | ||
68 | + "chrome/canary", | ||
69 | + "opera/12..latest", | ||
70 | + "opera/next", | ||
71 | + "safari/5.1..latest", | ||
72 | + "ipad/6.0..latest", | ||
73 | + "iphone/6.0..latest", | ||
74 | + "android-browser/4.2..latest" | ||
75 | + ] | ||
76 | + }, | ||
77 | + "version": "1.1.11" | ||
78 | +} |
node_modules/chalk/index.js
0 → 100644
1 | +'use strict'; | ||
2 | +const escapeStringRegexp = require('escape-string-regexp'); | ||
3 | +const ansiStyles = require('ansi-styles'); | ||
4 | +const stdoutColor = require('supports-color').stdout; | ||
5 | + | ||
6 | +const template = require('./templates.js'); | ||
7 | + | ||
8 | +const isSimpleWindowsTerm = process.platform === 'win32' && !(process.env.TERM || '').toLowerCase().startsWith('xterm'); | ||
9 | + | ||
10 | +// `supportsColor.level` → `ansiStyles.color[name]` mapping | ||
11 | +const levelMapping = ['ansi', 'ansi', 'ansi256', 'ansi16m']; | ||
12 | + | ||
13 | +// `color-convert` models to exclude from the Chalk API due to conflicts and such | ||
14 | +const skipModels = new Set(['gray']); | ||
15 | + | ||
16 | +const styles = Object.create(null); | ||
17 | + | ||
18 | +function applyOptions(obj, options) { | ||
19 | + options = options || {}; | ||
20 | + | ||
21 | + // Detect level if not set manually | ||
22 | + const scLevel = stdoutColor ? stdoutColor.level : 0; | ||
23 | + obj.level = options.level === undefined ? scLevel : options.level; | ||
24 | + obj.enabled = 'enabled' in options ? options.enabled : obj.level > 0; | ||
25 | +} | ||
26 | + | ||
27 | +function Chalk(options) { | ||
28 | + // We check for this.template here since calling `chalk.constructor()` | ||
29 | + // by itself will have a `this` of a previously constructed chalk object | ||
30 | + if (!this || !(this instanceof Chalk) || this.template) { | ||
31 | + const chalk = {}; | ||
32 | + applyOptions(chalk, options); | ||
33 | + | ||
34 | + chalk.template = function () { | ||
35 | + const args = [].slice.call(arguments); | ||
36 | + return chalkTag.apply(null, [chalk.template].concat(args)); | ||
37 | + }; | ||
38 | + | ||
39 | + Object.setPrototypeOf(chalk, Chalk.prototype); | ||
40 | + Object.setPrototypeOf(chalk.template, chalk); | ||
41 | + | ||
42 | + chalk.template.constructor = Chalk; | ||
43 | + | ||
44 | + return chalk.template; | ||
45 | + } | ||
46 | + | ||
47 | + applyOptions(this, options); | ||
48 | +} | ||
49 | + | ||
50 | +// Use bright blue on Windows as the normal blue color is illegible | ||
51 | +if (isSimpleWindowsTerm) { | ||
52 | + ansiStyles.blue.open = '\u001B[94m'; | ||
53 | +} | ||
54 | + | ||
55 | +for (const key of Object.keys(ansiStyles)) { | ||
56 | + ansiStyles[key].closeRe = new RegExp(escapeStringRegexp(ansiStyles[key].close), 'g'); | ||
57 | + | ||
58 | + styles[key] = { | ||
59 | + get() { | ||
60 | + const codes = ansiStyles[key]; | ||
61 | + return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, key); | ||
62 | + } | ||
63 | + }; | ||
64 | +} | ||
65 | + | ||
66 | +styles.visible = { | ||
67 | + get() { | ||
68 | + return build.call(this, this._styles || [], true, 'visible'); | ||
69 | + } | ||
70 | +}; | ||
71 | + | ||
72 | +ansiStyles.color.closeRe = new RegExp(escapeStringRegexp(ansiStyles.color.close), 'g'); | ||
73 | +for (const model of Object.keys(ansiStyles.color.ansi)) { | ||
74 | + if (skipModels.has(model)) { | ||
75 | + continue; | ||
76 | + } | ||
77 | + | ||
78 | + styles[model] = { | ||
79 | + get() { | ||
80 | + const level = this.level; | ||
81 | + return function () { | ||
82 | + const open = ansiStyles.color[levelMapping[level]][model].apply(null, arguments); | ||
83 | + const codes = { | ||
84 | + open, | ||
85 | + close: ansiStyles.color.close, | ||
86 | + closeRe: ansiStyles.color.closeRe | ||
87 | + }; | ||
88 | + return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, model); | ||
89 | + }; | ||
90 | + } | ||
91 | + }; | ||
92 | +} | ||
93 | + | ||
94 | +ansiStyles.bgColor.closeRe = new RegExp(escapeStringRegexp(ansiStyles.bgColor.close), 'g'); | ||
95 | +for (const model of Object.keys(ansiStyles.bgColor.ansi)) { | ||
96 | + if (skipModels.has(model)) { | ||
97 | + continue; | ||
98 | + } | ||
99 | + | ||
100 | + const bgModel = 'bg' + model[0].toUpperCase() + model.slice(1); | ||
101 | + styles[bgModel] = { | ||
102 | + get() { | ||
103 | + const level = this.level; | ||
104 | + return function () { | ||
105 | + const open = ansiStyles.bgColor[levelMapping[level]][model].apply(null, arguments); | ||
106 | + const codes = { | ||
107 | + open, | ||
108 | + close: ansiStyles.bgColor.close, | ||
109 | + closeRe: ansiStyles.bgColor.closeRe | ||
110 | + }; | ||
111 | + return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, model); | ||
112 | + }; | ||
113 | + } | ||
114 | + }; | ||
115 | +} | ||
116 | + | ||
117 | +const proto = Object.defineProperties(() => {}, styles); | ||
118 | + | ||
119 | +function build(_styles, _empty, key) { | ||
120 | + const builder = function () { | ||
121 | + return applyStyle.apply(builder, arguments); | ||
122 | + }; | ||
123 | + | ||
124 | + builder._styles = _styles; | ||
125 | + builder._empty = _empty; | ||
126 | + | ||
127 | + const self = this; | ||
128 | + | ||
129 | + Object.defineProperty(builder, 'level', { | ||
130 | + enumerable: true, | ||
131 | + get() { | ||
132 | + return self.level; | ||
133 | + }, | ||
134 | + set(level) { | ||
135 | + self.level = level; | ||
136 | + } | ||
137 | + }); | ||
138 | + | ||
139 | + Object.defineProperty(builder, 'enabled', { | ||
140 | + enumerable: true, | ||
141 | + get() { | ||
142 | + return self.enabled; | ||
143 | + }, | ||
144 | + set(enabled) { | ||
145 | + self.enabled = enabled; | ||
146 | + } | ||
147 | + }); | ||
148 | + | ||
149 | + // See below for fix regarding invisible grey/dim combination on Windows | ||
150 | + builder.hasGrey = this.hasGrey || key === 'gray' || key === 'grey'; | ||
151 | + | ||
152 | + // `__proto__` is used because we must return a function, but there is | ||
153 | + // no way to create a function with a different prototype | ||
154 | + builder.__proto__ = proto; // eslint-disable-line no-proto | ||
155 | + | ||
156 | + return builder; | ||
157 | +} | ||
158 | + | ||
159 | +function applyStyle() { | ||
160 | + // Support varags, but simply cast to string in case there's only one arg | ||
161 | + const args = arguments; | ||
162 | + const argsLen = args.length; | ||
163 | + let str = String(arguments[0]); | ||
164 | + | ||
165 | + if (argsLen === 0) { | ||
166 | + return ''; | ||
167 | + } | ||
168 | + | ||
169 | + if (argsLen > 1) { | ||
170 | + // Don't slice `arguments`, it prevents V8 optimizations | ||
171 | + for (let a = 1; a < argsLen; a++) { | ||
172 | + str += ' ' + args[a]; | ||
173 | + } | ||
174 | + } | ||
175 | + | ||
176 | + if (!this.enabled || this.level <= 0 || !str) { | ||
177 | + return this._empty ? '' : str; | ||
178 | + } | ||
179 | + | ||
180 | + // Turns out that on Windows dimmed gray text becomes invisible in cmd.exe, | ||
181 | + // see https://github.com/chalk/chalk/issues/58 | ||
182 | + // If we're on Windows and we're dealing with a gray color, temporarily make 'dim' a noop. | ||
183 | + const originalDim = ansiStyles.dim.open; | ||
184 | + if (isSimpleWindowsTerm && this.hasGrey) { | ||
185 | + ansiStyles.dim.open = ''; | ||
186 | + } | ||
187 | + | ||
188 | + for (const code of this._styles.slice().reverse()) { | ||
189 | + // Replace any instances already present with a re-opening code | ||
190 | + // otherwise only the part of the string until said closing code | ||
191 | + // will be colored, and the rest will simply be 'plain'. | ||
192 | + str = code.open + str.replace(code.closeRe, code.open) + code.close; | ||
193 | + | ||
194 | + // Close the styling before a linebreak and reopen | ||
195 | + // after next line to fix a bleed issue on macOS | ||
196 | + // https://github.com/chalk/chalk/pull/92 | ||
197 | + str = str.replace(/\r?\n/g, `${code.close}$&${code.open}`); | ||
198 | + } | ||
199 | + | ||
200 | + // Reset the original `dim` if we changed it to work around the Windows dimmed gray issue | ||
201 | + ansiStyles.dim.open = originalDim; | ||
202 | + | ||
203 | + return str; | ||
204 | +} | ||
205 | + | ||
206 | +function chalkTag(chalk, strings) { | ||
207 | + if (!Array.isArray(strings)) { | ||
208 | + // If chalk() was called by itself or with a string, | ||
209 | + // return the string itself as a string. | ||
210 | + return [].slice.call(arguments, 1).join(' '); | ||
211 | + } | ||
212 | + | ||
213 | + const args = [].slice.call(arguments, 2); | ||
214 | + const parts = [strings.raw[0]]; | ||
215 | + | ||
216 | + for (let i = 1; i < strings.length; i++) { | ||
217 | + parts.push(String(args[i - 1]).replace(/[{}\\]/g, '\\$&')); | ||
218 | + parts.push(String(strings.raw[i])); | ||
219 | + } | ||
220 | + | ||
221 | + return template(chalk, parts.join('')); | ||
222 | +} | ||
223 | + | ||
224 | +Object.defineProperties(Chalk.prototype, styles); | ||
225 | + | ||
226 | +module.exports = Chalk(); // eslint-disable-line new-cap | ||
227 | +module.exports.supportsColor = stdoutColor; | ||
228 | +module.exports.default = module.exports; // For TypeScript |
node_modules/chalk/index.js.flow
0 → 100644
1 | +// @flow strict | ||
2 | + | ||
3 | +type TemplateStringsArray = $ReadOnlyArray<string>; | ||
4 | + | ||
5 | +export type Level = $Values<{ | ||
6 | + None: 0, | ||
7 | + Basic: 1, | ||
8 | + Ansi256: 2, | ||
9 | + TrueColor: 3 | ||
10 | +}>; | ||
11 | + | ||
12 | +export type ChalkOptions = {| | ||
13 | + enabled?: boolean, | ||
14 | + level?: Level | ||
15 | +|}; | ||
16 | + | ||
17 | +export type ColorSupport = {| | ||
18 | + level: Level, | ||
19 | + hasBasic: boolean, | ||
20 | + has256: boolean, | ||
21 | + has16m: boolean | ||
22 | +|}; | ||
23 | + | ||
24 | +export interface Chalk { | ||
25 | + (...text: string[]): string, | ||
26 | + (text: TemplateStringsArray, ...placeholders: string[]): string, | ||
27 | + constructor(options?: ChalkOptions): Chalk, | ||
28 | + enabled: boolean, | ||
29 | + level: Level, | ||
30 | + rgb(r: number, g: number, b: number): Chalk, | ||
31 | + hsl(h: number, s: number, l: number): Chalk, | ||
32 | + hsv(h: number, s: number, v: number): Chalk, | ||
33 | + hwb(h: number, w: number, b: number): Chalk, | ||
34 | + bgHex(color: string): Chalk, | ||
35 | + bgKeyword(color: string): Chalk, | ||
36 | + bgRgb(r: number, g: number, b: number): Chalk, | ||
37 | + bgHsl(h: number, s: number, l: number): Chalk, | ||
38 | + bgHsv(h: number, s: number, v: number): Chalk, | ||
39 | + bgHwb(h: number, w: number, b: number): Chalk, | ||
40 | + hex(color: string): Chalk, | ||
41 | + keyword(color: string): Chalk, | ||
42 | + | ||
43 | + +reset: Chalk, | ||
44 | + +bold: Chalk, | ||
45 | + +dim: Chalk, | ||
46 | + +italic: Chalk, | ||
47 | + +underline: Chalk, | ||
48 | + +inverse: Chalk, | ||
49 | + +hidden: Chalk, | ||
50 | + +strikethrough: Chalk, | ||
51 | + | ||
52 | + +visible: Chalk, | ||
53 | + | ||
54 | + +black: Chalk, | ||
55 | + +red: Chalk, | ||
56 | + +green: Chalk, | ||
57 | + +yellow: Chalk, | ||
58 | + +blue: Chalk, | ||
59 | + +magenta: Chalk, | ||
60 | + +cyan: Chalk, | ||
61 | + +white: Chalk, | ||
62 | + +gray: Chalk, | ||
63 | + +grey: Chalk, | ||
64 | + +blackBright: Chalk, | ||
65 | + +redBright: Chalk, | ||
66 | + +greenBright: Chalk, | ||
67 | + +yellowBright: Chalk, | ||
68 | + +blueBright: Chalk, | ||
69 | + +magentaBright: Chalk, | ||
70 | + +cyanBright: Chalk, | ||
71 | + +whiteBright: Chalk, | ||
72 | + | ||
73 | + +bgBlack: Chalk, | ||
74 | + +bgRed: Chalk, | ||
75 | + +bgGreen: Chalk, | ||
76 | + +bgYellow: Chalk, | ||
77 | + +bgBlue: Chalk, | ||
78 | + +bgMagenta: Chalk, | ||
79 | + +bgCyan: Chalk, | ||
80 | + +bgWhite: Chalk, | ||
81 | + +bgBlackBright: Chalk, | ||
82 | + +bgRedBright: Chalk, | ||
83 | + +bgGreenBright: Chalk, | ||
84 | + +bgYellowBright: Chalk, | ||
85 | + +bgBlueBright: Chalk, | ||
86 | + +bgMagentaBright: Chalk, | ||
87 | + +bgCyanBright: Chalk, | ||
88 | + +bgWhiteBrigh: Chalk, | ||
89 | + | ||
90 | + supportsColor: ColorSupport | ||
91 | +}; | ||
92 | + | ||
93 | +declare module.exports: Chalk; |
node_modules/chalk/license
0 → 100644
1 | +MIT License | ||
2 | + | ||
3 | +Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: | ||
6 | + | ||
7 | +The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. | ||
8 | + | ||
9 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
node_modules/chalk/package.json
0 → 100644
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "chalk@2.4.2", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "chalk@2.4.2", | ||
9 | + "_id": "chalk@2.4.2", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha512-Mti+f9lpJNcwF4tWV8/OrTTtF1gZi+f8FqlyAdouralcFWFQWF2+NgCHShjkCb+IFBLq9buZwE1xckQU4peSuQ==", | ||
12 | + "_location": "/chalk", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "chalk@2.4.2", | ||
18 | + "name": "chalk", | ||
19 | + "escapedName": "chalk", | ||
20 | + "rawSpec": "2.4.2", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "2.4.2" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/jake" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/chalk/-/chalk-2.4.2.tgz", | ||
28 | + "_spec": "2.4.2", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "bugs": { | ||
31 | + "url": "https://github.com/chalk/chalk/issues" | ||
32 | + }, | ||
33 | + "dependencies": { | ||
34 | + "ansi-styles": "^3.2.1", | ||
35 | + "escape-string-regexp": "^1.0.5", | ||
36 | + "supports-color": "^5.3.0" | ||
37 | + }, | ||
38 | + "description": "Terminal string styling done right", | ||
39 | + "devDependencies": { | ||
40 | + "ava": "*", | ||
41 | + "coveralls": "^3.0.0", | ||
42 | + "execa": "^0.9.0", | ||
43 | + "flow-bin": "^0.68.0", | ||
44 | + "import-fresh": "^2.0.0", | ||
45 | + "matcha": "^0.7.0", | ||
46 | + "nyc": "^11.0.2", | ||
47 | + "resolve-from": "^4.0.0", | ||
48 | + "typescript": "^2.5.3", | ||
49 | + "xo": "*" | ||
50 | + }, | ||
51 | + "engines": { | ||
52 | + "node": ">=4" | ||
53 | + }, | ||
54 | + "files": [ | ||
55 | + "index.js", | ||
56 | + "templates.js", | ||
57 | + "types/index.d.ts", | ||
58 | + "index.js.flow" | ||
59 | + ], | ||
60 | + "homepage": "https://github.com/chalk/chalk#readme", | ||
61 | + "keywords": [ | ||
62 | + "color", | ||
63 | + "colour", | ||
64 | + "colors", | ||
65 | + "terminal", | ||
66 | + "console", | ||
67 | + "cli", | ||
68 | + "string", | ||
69 | + "str", | ||
70 | + "ansi", | ||
71 | + "style", | ||
72 | + "styles", | ||
73 | + "tty", | ||
74 | + "formatting", | ||
75 | + "rgb", | ||
76 | + "256", | ||
77 | + "shell", | ||
78 | + "xterm", | ||
79 | + "log", | ||
80 | + "logging", | ||
81 | + "command-line", | ||
82 | + "text" | ||
83 | + ], | ||
84 | + "license": "MIT", | ||
85 | + "name": "chalk", | ||
86 | + "repository": { | ||
87 | + "type": "git", | ||
88 | + "url": "git+https://github.com/chalk/chalk.git" | ||
89 | + }, | ||
90 | + "scripts": { | ||
91 | + "bench": "matcha benchmark.js", | ||
92 | + "coveralls": "nyc report --reporter=text-lcov | coveralls", | ||
93 | + "test": "xo && tsc --project types && flow --max-warnings=0 && nyc ava" | ||
94 | + }, | ||
95 | + "types": "types/index.d.ts", | ||
96 | + "version": "2.4.2", | ||
97 | + "xo": { | ||
98 | + "envs": [ | ||
99 | + "node", | ||
100 | + "mocha" | ||
101 | + ], | ||
102 | + "ignores": [ | ||
103 | + "test/_flow.js" | ||
104 | + ] | ||
105 | + } | ||
106 | +} |
node_modules/chalk/readme.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/chalk/templates.js
0 → 100644
1 | +'use strict'; | ||
2 | +const TEMPLATE_REGEX = /(?:\\(u[a-f\d]{4}|x[a-f\d]{2}|.))|(?:\{(~)?(\w+(?:\([^)]*\))?(?:\.\w+(?:\([^)]*\))?)*)(?:[ \t]|(?=\r?\n)))|(\})|((?:.|[\r\n\f])+?)/gi; | ||
3 | +const STYLE_REGEX = /(?:^|\.)(\w+)(?:\(([^)]*)\))?/g; | ||
4 | +const STRING_REGEX = /^(['"])((?:\\.|(?!\1)[^\\])*)\1$/; | ||
5 | +const ESCAPE_REGEX = /\\(u[a-f\d]{4}|x[a-f\d]{2}|.)|([^\\])/gi; | ||
6 | + | ||
7 | +const ESCAPES = new Map([ | ||
8 | + ['n', '\n'], | ||
9 | + ['r', '\r'], | ||
10 | + ['t', '\t'], | ||
11 | + ['b', '\b'], | ||
12 | + ['f', '\f'], | ||
13 | + ['v', '\v'], | ||
14 | + ['0', '\0'], | ||
15 | + ['\\', '\\'], | ||
16 | + ['e', '\u001B'], | ||
17 | + ['a', '\u0007'] | ||
18 | +]); | ||
19 | + | ||
20 | +function unescape(c) { | ||
21 | + if ((c[0] === 'u' && c.length === 5) || (c[0] === 'x' && c.length === 3)) { | ||
22 | + return String.fromCharCode(parseInt(c.slice(1), 16)); | ||
23 | + } | ||
24 | + | ||
25 | + return ESCAPES.get(c) || c; | ||
26 | +} | ||
27 | + | ||
28 | +function parseArguments(name, args) { | ||
29 | + const results = []; | ||
30 | + const chunks = args.trim().split(/\s*,\s*/g); | ||
31 | + let matches; | ||
32 | + | ||
33 | + for (const chunk of chunks) { | ||
34 | + if (!isNaN(chunk)) { | ||
35 | + results.push(Number(chunk)); | ||
36 | + } else if ((matches = chunk.match(STRING_REGEX))) { | ||
37 | + results.push(matches[2].replace(ESCAPE_REGEX, (m, escape, chr) => escape ? unescape(escape) : chr)); | ||
38 | + } else { | ||
39 | + throw new Error(`Invalid Chalk template style argument: ${chunk} (in style '${name}')`); | ||
40 | + } | ||
41 | + } | ||
42 | + | ||
43 | + return results; | ||
44 | +} | ||
45 | + | ||
46 | +function parseStyle(style) { | ||
47 | + STYLE_REGEX.lastIndex = 0; | ||
48 | + | ||
49 | + const results = []; | ||
50 | + let matches; | ||
51 | + | ||
52 | + while ((matches = STYLE_REGEX.exec(style)) !== null) { | ||
53 | + const name = matches[1]; | ||
54 | + | ||
55 | + if (matches[2]) { | ||
56 | + const args = parseArguments(name, matches[2]); | ||
57 | + results.push([name].concat(args)); | ||
58 | + } else { | ||
59 | + results.push([name]); | ||
60 | + } | ||
61 | + } | ||
62 | + | ||
63 | + return results; | ||
64 | +} | ||
65 | + | ||
66 | +function buildStyle(chalk, styles) { | ||
67 | + const enabled = {}; | ||
68 | + | ||
69 | + for (const layer of styles) { | ||
70 | + for (const style of layer.styles) { | ||
71 | + enabled[style[0]] = layer.inverse ? null : style.slice(1); | ||
72 | + } | ||
73 | + } | ||
74 | + | ||
75 | + let current = chalk; | ||
76 | + for (const styleName of Object.keys(enabled)) { | ||
77 | + if (Array.isArray(enabled[styleName])) { | ||
78 | + if (!(styleName in current)) { | ||
79 | + throw new Error(`Unknown Chalk style: ${styleName}`); | ||
80 | + } | ||
81 | + | ||
82 | + if (enabled[styleName].length > 0) { | ||
83 | + current = current[styleName].apply(current, enabled[styleName]); | ||
84 | + } else { | ||
85 | + current = current[styleName]; | ||
86 | + } | ||
87 | + } | ||
88 | + } | ||
89 | + | ||
90 | + return current; | ||
91 | +} | ||
92 | + | ||
93 | +module.exports = (chalk, tmp) => { | ||
94 | + const styles = []; | ||
95 | + const chunks = []; | ||
96 | + let chunk = []; | ||
97 | + | ||
98 | + // eslint-disable-next-line max-params | ||
99 | + tmp.replace(TEMPLATE_REGEX, (m, escapeChar, inverse, style, close, chr) => { | ||
100 | + if (escapeChar) { | ||
101 | + chunk.push(unescape(escapeChar)); | ||
102 | + } else if (style) { | ||
103 | + const str = chunk.join(''); | ||
104 | + chunk = []; | ||
105 | + chunks.push(styles.length === 0 ? str : buildStyle(chalk, styles)(str)); | ||
106 | + styles.push({inverse, styles: parseStyle(style)}); | ||
107 | + } else if (close) { | ||
108 | + if (styles.length === 0) { | ||
109 | + throw new Error('Found extraneous } in Chalk template literal'); | ||
110 | + } | ||
111 | + | ||
112 | + chunks.push(buildStyle(chalk, styles)(chunk.join(''))); | ||
113 | + chunk = []; | ||
114 | + styles.pop(); | ||
115 | + } else { | ||
116 | + chunk.push(chr); | ||
117 | + } | ||
118 | + }); | ||
119 | + | ||
120 | + chunks.push(chunk.join('')); | ||
121 | + | ||
122 | + if (styles.length > 0) { | ||
123 | + const errMsg = `Chalk template literal is missing ${styles.length} closing bracket${styles.length === 1 ? '' : 's'} (\`}\`)`; | ||
124 | + throw new Error(errMsg); | ||
125 | + } | ||
126 | + | ||
127 | + return chunks.join(''); | ||
128 | +}; |
node_modules/chalk/types/index.d.ts
0 → 100644
1 | +// Type definitions for Chalk | ||
2 | +// Definitions by: Thomas Sauer <https://github.com/t-sauer> | ||
3 | + | ||
4 | +export const enum Level { | ||
5 | + None = 0, | ||
6 | + Basic = 1, | ||
7 | + Ansi256 = 2, | ||
8 | + TrueColor = 3 | ||
9 | +} | ||
10 | + | ||
11 | +export interface ChalkOptions { | ||
12 | + enabled?: boolean; | ||
13 | + level?: Level; | ||
14 | +} | ||
15 | + | ||
16 | +export interface ChalkConstructor { | ||
17 | + new (options?: ChalkOptions): Chalk; | ||
18 | + (options?: ChalkOptions): Chalk; | ||
19 | +} | ||
20 | + | ||
21 | +export interface ColorSupport { | ||
22 | + level: Level; | ||
23 | + hasBasic: boolean; | ||
24 | + has256: boolean; | ||
25 | + has16m: boolean; | ||
26 | +} | ||
27 | + | ||
28 | +export interface Chalk { | ||
29 | + (...text: string[]): string; | ||
30 | + (text: TemplateStringsArray, ...placeholders: string[]): string; | ||
31 | + constructor: ChalkConstructor; | ||
32 | + enabled: boolean; | ||
33 | + level: Level; | ||
34 | + rgb(r: number, g: number, b: number): this; | ||
35 | + hsl(h: number, s: number, l: number): this; | ||
36 | + hsv(h: number, s: number, v: number): this; | ||
37 | + hwb(h: number, w: number, b: number): this; | ||
38 | + bgHex(color: string): this; | ||
39 | + bgKeyword(color: string): this; | ||
40 | + bgRgb(r: number, g: number, b: number): this; | ||
41 | + bgHsl(h: number, s: number, l: number): this; | ||
42 | + bgHsv(h: number, s: number, v: number): this; | ||
43 | + bgHwb(h: number, w: number, b: number): this; | ||
44 | + hex(color: string): this; | ||
45 | + keyword(color: string): this; | ||
46 | + | ||
47 | + readonly reset: this; | ||
48 | + readonly bold: this; | ||
49 | + readonly dim: this; | ||
50 | + readonly italic: this; | ||
51 | + readonly underline: this; | ||
52 | + readonly inverse: this; | ||
53 | + readonly hidden: this; | ||
54 | + readonly strikethrough: this; | ||
55 | + | ||
56 | + readonly visible: this; | ||
57 | + | ||
58 | + readonly black: this; | ||
59 | + readonly red: this; | ||
60 | + readonly green: this; | ||
61 | + readonly yellow: this; | ||
62 | + readonly blue: this; | ||
63 | + readonly magenta: this; | ||
64 | + readonly cyan: this; | ||
65 | + readonly white: this; | ||
66 | + readonly gray: this; | ||
67 | + readonly grey: this; | ||
68 | + readonly blackBright: this; | ||
69 | + readonly redBright: this; | ||
70 | + readonly greenBright: this; | ||
71 | + readonly yellowBright: this; | ||
72 | + readonly blueBright: this; | ||
73 | + readonly magentaBright: this; | ||
74 | + readonly cyanBright: this; | ||
75 | + readonly whiteBright: this; | ||
76 | + | ||
77 | + readonly bgBlack: this; | ||
78 | + readonly bgRed: this; | ||
79 | + readonly bgGreen: this; | ||
80 | + readonly bgYellow: this; | ||
81 | + readonly bgBlue: this; | ||
82 | + readonly bgMagenta: this; | ||
83 | + readonly bgCyan: this; | ||
84 | + readonly bgWhite: this; | ||
85 | + readonly bgBlackBright: this; | ||
86 | + readonly bgRedBright: this; | ||
87 | + readonly bgGreenBright: this; | ||
88 | + readonly bgYellowBright: this; | ||
89 | + readonly bgBlueBright: this; | ||
90 | + readonly bgMagentaBright: this; | ||
91 | + readonly bgCyanBright: this; | ||
92 | + readonly bgWhiteBright: this; | ||
93 | +} | ||
94 | + | ||
95 | +declare const chalk: Chalk & { supportsColor: ColorSupport }; | ||
96 | + | ||
97 | +export default chalk |
node_modules/color-convert/CHANGELOG.md
0 → 100644
1 | +# 1.0.0 - 2016-01-07 | ||
2 | + | ||
3 | +- Removed: unused speed test | ||
4 | +- Added: Automatic routing between previously unsupported conversions | ||
5 | +([#27](https://github.com/Qix-/color-convert/pull/27)) | ||
6 | +- Removed: `xxx2xxx()` and `xxx2xxxRaw()` functions | ||
7 | +([#27](https://github.com/Qix-/color-convert/pull/27)) | ||
8 | +- Removed: `convert()` class | ||
9 | +([#27](https://github.com/Qix-/color-convert/pull/27)) | ||
10 | +- Changed: all functions to lookup dictionary | ||
11 | +([#27](https://github.com/Qix-/color-convert/pull/27)) | ||
12 | +- Changed: `ansi` to `ansi256` | ||
13 | +([#27](https://github.com/Qix-/color-convert/pull/27)) | ||
14 | +- Fixed: argument grouping for functions requiring only one argument | ||
15 | +([#27](https://github.com/Qix-/color-convert/pull/27)) | ||
16 | + | ||
17 | +# 0.6.0 - 2015-07-23 | ||
18 | + | ||
19 | +- Added: methods to handle | ||
20 | +[ANSI](https://en.wikipedia.org/wiki/ANSI_escape_code#Colors) 16/256 colors: | ||
21 | + - rgb2ansi16 | ||
22 | + - rgb2ansi | ||
23 | + - hsl2ansi16 | ||
24 | + - hsl2ansi | ||
25 | + - hsv2ansi16 | ||
26 | + - hsv2ansi | ||
27 | + - hwb2ansi16 | ||
28 | + - hwb2ansi | ||
29 | + - cmyk2ansi16 | ||
30 | + - cmyk2ansi | ||
31 | + - keyword2ansi16 | ||
32 | + - keyword2ansi | ||
33 | + - ansi162rgb | ||
34 | + - ansi162hsl | ||
35 | + - ansi162hsv | ||
36 | + - ansi162hwb | ||
37 | + - ansi162cmyk | ||
38 | + - ansi162keyword | ||
39 | + - ansi2rgb | ||
40 | + - ansi2hsl | ||
41 | + - ansi2hsv | ||
42 | + - ansi2hwb | ||
43 | + - ansi2cmyk | ||
44 | + - ansi2keyword | ||
45 | +([#18](https://github.com/harthur/color-convert/pull/18)) | ||
46 | + | ||
47 | +# 0.5.3 - 2015-06-02 | ||
48 | + | ||
49 | +- Fixed: hsl2hsv does not return `NaN` anymore when using `[0,0,0]` | ||
50 | +([#15](https://github.com/harthur/color-convert/issues/15)) | ||
51 | + | ||
52 | +--- | ||
53 | + | ||
54 | +Check out commit logs for older releases |
node_modules/color-convert/LICENSE
0 → 100644
1 | +Copyright (c) 2011-2016 Heather Arthur <fayearthur@gmail.com> | ||
2 | + | ||
3 | +Permission is hereby granted, free of charge, to any person obtaining | ||
4 | +a copy of this software and associated documentation files (the | ||
5 | +"Software"), to deal in the Software without restriction, including | ||
6 | +without limitation the rights to use, copy, modify, merge, publish, | ||
7 | +distribute, sublicense, and/or sell copies of the Software, and to | ||
8 | +permit persons to whom the Software is furnished to do so, subject to | ||
9 | +the following conditions: | ||
10 | + | ||
11 | +The above copyright notice and this permission notice shall be | ||
12 | +included in all copies or substantial portions of the Software. | ||
13 | + | ||
14 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, | ||
15 | +EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF | ||
16 | +MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND | ||
17 | +NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE | ||
18 | +LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION | ||
19 | +OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION | ||
20 | +WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
21 | + |
node_modules/color-convert/README.md
0 → 100644
1 | +# color-convert | ||
2 | + | ||
3 | +[](https://travis-ci.org/Qix-/color-convert) | ||
4 | + | ||
5 | +Color-convert is a color conversion library for JavaScript and node. | ||
6 | +It converts all ways between `rgb`, `hsl`, `hsv`, `hwb`, `cmyk`, `ansi`, `ansi16`, `hex` strings, and CSS `keyword`s (will round to closest): | ||
7 | + | ||
8 | +```js | ||
9 | +var convert = require('color-convert'); | ||
10 | + | ||
11 | +convert.rgb.hsl(140, 200, 100); // [96, 48, 59] | ||
12 | +convert.keyword.rgb('blue'); // [0, 0, 255] | ||
13 | + | ||
14 | +var rgbChannels = convert.rgb.channels; // 3 | ||
15 | +var cmykChannels = convert.cmyk.channels; // 4 | ||
16 | +var ansiChannels = convert.ansi16.channels; // 1 | ||
17 | +``` | ||
18 | + | ||
19 | +# Install | ||
20 | + | ||
21 | +```console | ||
22 | +$ npm install color-convert | ||
23 | +``` | ||
24 | + | ||
25 | +# API | ||
26 | + | ||
27 | +Simply get the property of the _from_ and _to_ conversion that you're looking for. | ||
28 | + | ||
29 | +All functions have a rounded and unrounded variant. By default, return values are rounded. To get the unrounded (raw) results, simply tack on `.raw` to the function. | ||
30 | + | ||
31 | +All 'from' functions have a hidden property called `.channels` that indicates the number of channels the function expects (not including alpha). | ||
32 | + | ||
33 | +```js | ||
34 | +var convert = require('color-convert'); | ||
35 | + | ||
36 | +// Hex to LAB | ||
37 | +convert.hex.lab('DEADBF'); // [ 76, 21, -2 ] | ||
38 | +convert.hex.lab.raw('DEADBF'); // [ 75.56213190997677, 20.653827952644754, -2.290532499330533 ] | ||
39 | + | ||
40 | +// RGB to CMYK | ||
41 | +convert.rgb.cmyk(167, 255, 4); // [ 35, 0, 98, 0 ] | ||
42 | +convert.rgb.cmyk.raw(167, 255, 4); // [ 34.509803921568626, 0, 98.43137254901961, 0 ] | ||
43 | +``` | ||
44 | + | ||
45 | +### Arrays | ||
46 | +All functions that accept multiple arguments also support passing an array. | ||
47 | + | ||
48 | +Note that this does **not** apply to functions that convert from a color that only requires one value (e.g. `keyword`, `ansi256`, `hex`, etc.) | ||
49 | + | ||
50 | +```js | ||
51 | +var convert = require('color-convert'); | ||
52 | + | ||
53 | +convert.rgb.hex(123, 45, 67); // '7B2D43' | ||
54 | +convert.rgb.hex([123, 45, 67]); // '7B2D43' | ||
55 | +``` | ||
56 | + | ||
57 | +## Routing | ||
58 | + | ||
59 | +Conversions that don't have an _explicitly_ defined conversion (in [conversions.js](conversions.js)), but can be converted by means of sub-conversions (e.g. XYZ -> **RGB** -> CMYK), are automatically routed together. This allows just about any color model supported by `color-convert` to be converted to any other model, so long as a sub-conversion path exists. This is also true for conversions requiring more than one step in between (e.g. LCH -> **LAB** -> **XYZ** -> **RGB** -> Hex). | ||
60 | + | ||
61 | +Keep in mind that extensive conversions _may_ result in a loss of precision, and exist only to be complete. For a list of "direct" (single-step) conversions, see [conversions.js](conversions.js). | ||
62 | + | ||
63 | +# Contribute | ||
64 | + | ||
65 | +If there is a new model you would like to support, or want to add a direct conversion between two existing models, please send us a pull request. | ||
66 | + | ||
67 | +# License | ||
68 | +Copyright © 2011-2016, Heather Arthur and Josh Junon. Licensed under the [MIT License](LICENSE). |
node_modules/color-convert/conversions.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/color-convert/index.js
0 → 100644
1 | +var conversions = require('./conversions'); | ||
2 | +var route = require('./route'); | ||
3 | + | ||
4 | +var convert = {}; | ||
5 | + | ||
6 | +var models = Object.keys(conversions); | ||
7 | + | ||
8 | +function wrapRaw(fn) { | ||
9 | + var wrappedFn = function (args) { | ||
10 | + if (args === undefined || args === null) { | ||
11 | + return args; | ||
12 | + } | ||
13 | + | ||
14 | + if (arguments.length > 1) { | ||
15 | + args = Array.prototype.slice.call(arguments); | ||
16 | + } | ||
17 | + | ||
18 | + return fn(args); | ||
19 | + }; | ||
20 | + | ||
21 | + // preserve .conversion property if there is one | ||
22 | + if ('conversion' in fn) { | ||
23 | + wrappedFn.conversion = fn.conversion; | ||
24 | + } | ||
25 | + | ||
26 | + return wrappedFn; | ||
27 | +} | ||
28 | + | ||
29 | +function wrapRounded(fn) { | ||
30 | + var wrappedFn = function (args) { | ||
31 | + if (args === undefined || args === null) { | ||
32 | + return args; | ||
33 | + } | ||
34 | + | ||
35 | + if (arguments.length > 1) { | ||
36 | + args = Array.prototype.slice.call(arguments); | ||
37 | + } | ||
38 | + | ||
39 | + var result = fn(args); | ||
40 | + | ||
41 | + // we're assuming the result is an array here. | ||
42 | + // see notice in conversions.js; don't use box types | ||
43 | + // in conversion functions. | ||
44 | + if (typeof result === 'object') { | ||
45 | + for (var len = result.length, i = 0; i < len; i++) { | ||
46 | + result[i] = Math.round(result[i]); | ||
47 | + } | ||
48 | + } | ||
49 | + | ||
50 | + return result; | ||
51 | + }; | ||
52 | + | ||
53 | + // preserve .conversion property if there is one | ||
54 | + if ('conversion' in fn) { | ||
55 | + wrappedFn.conversion = fn.conversion; | ||
56 | + } | ||
57 | + | ||
58 | + return wrappedFn; | ||
59 | +} | ||
60 | + | ||
61 | +models.forEach(function (fromModel) { | ||
62 | + convert[fromModel] = {}; | ||
63 | + | ||
64 | + Object.defineProperty(convert[fromModel], 'channels', {value: conversions[fromModel].channels}); | ||
65 | + Object.defineProperty(convert[fromModel], 'labels', {value: conversions[fromModel].labels}); | ||
66 | + | ||
67 | + var routes = route(fromModel); | ||
68 | + var routeModels = Object.keys(routes); | ||
69 | + | ||
70 | + routeModels.forEach(function (toModel) { | ||
71 | + var fn = routes[toModel]; | ||
72 | + | ||
73 | + convert[fromModel][toModel] = wrapRounded(fn); | ||
74 | + convert[fromModel][toModel].raw = wrapRaw(fn); | ||
75 | + }); | ||
76 | +}); | ||
77 | + | ||
78 | +module.exports = convert; |
node_modules/color-convert/package.json
0 → 100644
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "color-convert@1.9.3", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "color-convert@1.9.3", | ||
9 | + "_id": "color-convert@1.9.3", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha512-QfAUtd+vFdAtFQcC8CCyYt1fYWxSqAiK2cSD6zDB8N3cpsEBAvRxp9zOGg6G/SHHJYAT88/az/IuDGALsNVbGg==", | ||
12 | + "_location": "/color-convert", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "color-convert@1.9.3", | ||
18 | + "name": "color-convert", | ||
19 | + "escapedName": "color-convert", | ||
20 | + "rawSpec": "1.9.3", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "1.9.3" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/ansi-styles" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/color-convert/-/color-convert-1.9.3.tgz", | ||
28 | + "_spec": "1.9.3", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "author": { | ||
31 | + "name": "Heather Arthur", | ||
32 | + "email": "fayearthur@gmail.com" | ||
33 | + }, | ||
34 | + "bugs": { | ||
35 | + "url": "https://github.com/Qix-/color-convert/issues" | ||
36 | + }, | ||
37 | + "dependencies": { | ||
38 | + "color-name": "1.1.3" | ||
39 | + }, | ||
40 | + "description": "Plain color conversion functions", | ||
41 | + "devDependencies": { | ||
42 | + "chalk": "1.1.1", | ||
43 | + "xo": "0.11.2" | ||
44 | + }, | ||
45 | + "files": [ | ||
46 | + "index.js", | ||
47 | + "conversions.js", | ||
48 | + "css-keywords.js", | ||
49 | + "route.js" | ||
50 | + ], | ||
51 | + "homepage": "https://github.com/Qix-/color-convert#readme", | ||
52 | + "keywords": [ | ||
53 | + "color", | ||
54 | + "colour", | ||
55 | + "convert", | ||
56 | + "converter", | ||
57 | + "conversion", | ||
58 | + "rgb", | ||
59 | + "hsl", | ||
60 | + "hsv", | ||
61 | + "hwb", | ||
62 | + "cmyk", | ||
63 | + "ansi", | ||
64 | + "ansi16" | ||
65 | + ], | ||
66 | + "license": "MIT", | ||
67 | + "name": "color-convert", | ||
68 | + "repository": { | ||
69 | + "type": "git", | ||
70 | + "url": "git+https://github.com/Qix-/color-convert.git" | ||
71 | + }, | ||
72 | + "scripts": { | ||
73 | + "pretest": "xo", | ||
74 | + "test": "node test/basic.js" | ||
75 | + }, | ||
76 | + "version": "1.9.3", | ||
77 | + "xo": { | ||
78 | + "rules": { | ||
79 | + "default-case": 0, | ||
80 | + "no-inline-comments": 0, | ||
81 | + "operator-linebreak": 0 | ||
82 | + } | ||
83 | + } | ||
84 | +} |
node_modules/color-convert/route.js
0 → 100644
1 | +var conversions = require('./conversions'); | ||
2 | + | ||
3 | +/* | ||
4 | + this function routes a model to all other models. | ||
5 | + | ||
6 | + all functions that are routed have a property `.conversion` attached | ||
7 | + to the returned synthetic function. This property is an array | ||
8 | + of strings, each with the steps in between the 'from' and 'to' | ||
9 | + color models (inclusive). | ||
10 | + | ||
11 | + conversions that are not possible simply are not included. | ||
12 | +*/ | ||
13 | + | ||
14 | +function buildGraph() { | ||
15 | + var graph = {}; | ||
16 | + // https://jsperf.com/object-keys-vs-for-in-with-closure/3 | ||
17 | + var models = Object.keys(conversions); | ||
18 | + | ||
19 | + for (var len = models.length, i = 0; i < len; i++) { | ||
20 | + graph[models[i]] = { | ||
21 | + // http://jsperf.com/1-vs-infinity | ||
22 | + // micro-opt, but this is simple. | ||
23 | + distance: -1, | ||
24 | + parent: null | ||
25 | + }; | ||
26 | + } | ||
27 | + | ||
28 | + return graph; | ||
29 | +} | ||
30 | + | ||
31 | +// https://en.wikipedia.org/wiki/Breadth-first_search | ||
32 | +function deriveBFS(fromModel) { | ||
33 | + var graph = buildGraph(); | ||
34 | + var queue = [fromModel]; // unshift -> queue -> pop | ||
35 | + | ||
36 | + graph[fromModel].distance = 0; | ||
37 | + | ||
38 | + while (queue.length) { | ||
39 | + var current = queue.pop(); | ||
40 | + var adjacents = Object.keys(conversions[current]); | ||
41 | + | ||
42 | + for (var len = adjacents.length, i = 0; i < len; i++) { | ||
43 | + var adjacent = adjacents[i]; | ||
44 | + var node = graph[adjacent]; | ||
45 | + | ||
46 | + if (node.distance === -1) { | ||
47 | + node.distance = graph[current].distance + 1; | ||
48 | + node.parent = current; | ||
49 | + queue.unshift(adjacent); | ||
50 | + } | ||
51 | + } | ||
52 | + } | ||
53 | + | ||
54 | + return graph; | ||
55 | +} | ||
56 | + | ||
57 | +function link(from, to) { | ||
58 | + return function (args) { | ||
59 | + return to(from(args)); | ||
60 | + }; | ||
61 | +} | ||
62 | + | ||
63 | +function wrapConversion(toModel, graph) { | ||
64 | + var path = [graph[toModel].parent, toModel]; | ||
65 | + var fn = conversions[graph[toModel].parent][toModel]; | ||
66 | + | ||
67 | + var cur = graph[toModel].parent; | ||
68 | + while (graph[cur].parent) { | ||
69 | + path.unshift(graph[cur].parent); | ||
70 | + fn = link(conversions[graph[cur].parent][cur], fn); | ||
71 | + cur = graph[cur].parent; | ||
72 | + } | ||
73 | + | ||
74 | + fn.conversion = path; | ||
75 | + return fn; | ||
76 | +} | ||
77 | + | ||
78 | +module.exports = function (fromModel) { | ||
79 | + var graph = deriveBFS(fromModel); | ||
80 | + var conversion = {}; | ||
81 | + | ||
82 | + var models = Object.keys(graph); | ||
83 | + for (var len = models.length, i = 0; i < len; i++) { | ||
84 | + var toModel = models[i]; | ||
85 | + var node = graph[toModel]; | ||
86 | + | ||
87 | + if (node.parent === null) { | ||
88 | + // no possible conversion, or this node is the source model. | ||
89 | + continue; | ||
90 | + } | ||
91 | + | ||
92 | + conversion[toModel] = wrapConversion(toModel, graph); | ||
93 | + } | ||
94 | + | ||
95 | + return conversion; | ||
96 | +}; | ||
97 | + |
node_modules/color-name/.eslintrc.json
0 → 100644
1 | +{ | ||
2 | + "env": { | ||
3 | + "browser": true, | ||
4 | + "node": true, | ||
5 | + "commonjs": true, | ||
6 | + "es6": true | ||
7 | + }, | ||
8 | + "extends": "eslint:recommended", | ||
9 | + "rules": { | ||
10 | + "strict": 2, | ||
11 | + "indent": 0, | ||
12 | + "linebreak-style": 0, | ||
13 | + "quotes": 0, | ||
14 | + "semi": 0, | ||
15 | + "no-cond-assign": 1, | ||
16 | + "no-constant-condition": 1, | ||
17 | + "no-duplicate-case": 1, | ||
18 | + "no-empty": 1, | ||
19 | + "no-ex-assign": 1, | ||
20 | + "no-extra-boolean-cast": 1, | ||
21 | + "no-extra-semi": 1, | ||
22 | + "no-fallthrough": 1, | ||
23 | + "no-func-assign": 1, | ||
24 | + "no-global-assign": 1, | ||
25 | + "no-implicit-globals": 2, | ||
26 | + "no-inner-declarations": ["error", "functions"], | ||
27 | + "no-irregular-whitespace": 2, | ||
28 | + "no-loop-func": 1, | ||
29 | + "no-multi-str": 1, | ||
30 | + "no-mixed-spaces-and-tabs": 1, | ||
31 | + "no-proto": 1, | ||
32 | + "no-sequences": 1, | ||
33 | + "no-throw-literal": 1, | ||
34 | + "no-unmodified-loop-condition": 1, | ||
35 | + "no-useless-call": 1, | ||
36 | + "no-void": 1, | ||
37 | + "no-with": 2, | ||
38 | + "wrap-iife": 1, | ||
39 | + "no-redeclare": 1, | ||
40 | + "no-unused-vars": ["error", { "vars": "all", "args": "none" }], | ||
41 | + "no-sparse-arrays": 1 | ||
42 | + } | ||
43 | +} |
node_modules/color-name/.npmignore
0 → 100644
1 | +//this will affect all the git repos | ||
2 | +git config --global core.excludesfile ~/.gitignore | ||
3 | + | ||
4 | + | ||
5 | +//update files since .ignore won't if already tracked | ||
6 | +git rm --cached <file> | ||
7 | + | ||
8 | +# Compiled source # | ||
9 | +################### | ||
10 | +*.com | ||
11 | +*.class | ||
12 | +*.dll | ||
13 | +*.exe | ||
14 | +*.o | ||
15 | +*.so | ||
16 | + | ||
17 | +# Packages # | ||
18 | +############ | ||
19 | +# it's better to unpack these files and commit the raw source | ||
20 | +# git has its own built in compression methods | ||
21 | +*.7z | ||
22 | +*.dmg | ||
23 | +*.gz | ||
24 | +*.iso | ||
25 | +*.jar | ||
26 | +*.rar | ||
27 | +*.tar | ||
28 | +*.zip | ||
29 | + | ||
30 | +# Logs and databases # | ||
31 | +###################### | ||
32 | +*.log | ||
33 | +*.sql | ||
34 | +*.sqlite | ||
35 | + | ||
36 | +# OS generated files # | ||
37 | +###################### | ||
38 | +.DS_Store | ||
39 | +.DS_Store? | ||
40 | +._* | ||
41 | +.Spotlight-V100 | ||
42 | +.Trashes | ||
43 | +# Icon? | ||
44 | +ehthumbs.db | ||
45 | +Thumbs.db | ||
46 | +.cache | ||
47 | +.project | ||
48 | +.settings | ||
49 | +.tmproj | ||
50 | +*.esproj | ||
51 | +nbproject | ||
52 | + | ||
53 | +# Numerous always-ignore extensions # | ||
54 | +##################################### | ||
55 | +*.diff | ||
56 | +*.err | ||
57 | +*.orig | ||
58 | +*.rej | ||
59 | +*.swn | ||
60 | +*.swo | ||
61 | +*.swp | ||
62 | +*.vi | ||
63 | +*~ | ||
64 | +*.sass-cache | ||
65 | +*.grunt | ||
66 | +*.tmp | ||
67 | + | ||
68 | +# Dreamweaver added files # | ||
69 | +########################### | ||
70 | +_notes | ||
71 | +dwsync.xml | ||
72 | + | ||
73 | +# Komodo # | ||
74 | +########################### | ||
75 | +*.komodoproject | ||
76 | +.komodotools | ||
77 | + | ||
78 | +# Node # | ||
79 | +##################### | ||
80 | +node_modules | ||
81 | + | ||
82 | +# Bower # | ||
83 | +##################### | ||
84 | +bower_components | ||
85 | + | ||
86 | +# Folders to ignore # | ||
87 | +##################### | ||
88 | +.hg | ||
89 | +.svn | ||
90 | +.CVS | ||
91 | +intermediate | ||
92 | +publish | ||
93 | +.idea | ||
94 | +.graphics | ||
95 | +_test | ||
96 | +_archive | ||
97 | +uploads | ||
98 | +tmp | ||
99 | + | ||
100 | +# Vim files to ignore # | ||
101 | +####################### | ||
102 | +.VimballRecord | ||
103 | +.netrwhist | ||
104 | + | ||
105 | +bundle.* | ||
106 | + | ||
107 | +_demo | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
node_modules/color-name/LICENSE
0 → 100644
1 | +The MIT License (MIT) | ||
2 | +Copyright (c) 2015 Dmitry Ivanov | ||
3 | + | ||
4 | +Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: | ||
5 | + | ||
6 | +The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. | ||
7 | + | ||
8 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
node_modules/color-name/README.md
0 → 100644
1 | +A JSON with color names and its values. Based on http://dev.w3.org/csswg/css-color/#named-colors. | ||
2 | + | ||
3 | +[](https://nodei.co/npm/color-name/) | ||
4 | + | ||
5 | + | ||
6 | +```js | ||
7 | +var colors = require('color-name'); | ||
8 | +colors.red //[255,0,0] | ||
9 | +``` | ||
10 | + | ||
11 | +<a href="LICENSE"><img src="https://upload.wikimedia.org/wikipedia/commons/0/0c/MIT_logo.svg" width="120"/></a> |
node_modules/color-name/index.js
0 → 100644
1 | +'use strict' | ||
2 | + | ||
3 | +module.exports = { | ||
4 | + "aliceblue": [240, 248, 255], | ||
5 | + "antiquewhite": [250, 235, 215], | ||
6 | + "aqua": [0, 255, 255], | ||
7 | + "aquamarine": [127, 255, 212], | ||
8 | + "azure": [240, 255, 255], | ||
9 | + "beige": [245, 245, 220], | ||
10 | + "bisque": [255, 228, 196], | ||
11 | + "black": [0, 0, 0], | ||
12 | + "blanchedalmond": [255, 235, 205], | ||
13 | + "blue": [0, 0, 255], | ||
14 | + "blueviolet": [138, 43, 226], | ||
15 | + "brown": [165, 42, 42], | ||
16 | + "burlywood": [222, 184, 135], | ||
17 | + "cadetblue": [95, 158, 160], | ||
18 | + "chartreuse": [127, 255, 0], | ||
19 | + "chocolate": [210, 105, 30], | ||
20 | + "coral": [255, 127, 80], | ||
21 | + "cornflowerblue": [100, 149, 237], | ||
22 | + "cornsilk": [255, 248, 220], | ||
23 | + "crimson": [220, 20, 60], | ||
24 | + "cyan": [0, 255, 255], | ||
25 | + "darkblue": [0, 0, 139], | ||
26 | + "darkcyan": [0, 139, 139], | ||
27 | + "darkgoldenrod": [184, 134, 11], | ||
28 | + "darkgray": [169, 169, 169], | ||
29 | + "darkgreen": [0, 100, 0], | ||
30 | + "darkgrey": [169, 169, 169], | ||
31 | + "darkkhaki": [189, 183, 107], | ||
32 | + "darkmagenta": [139, 0, 139], | ||
33 | + "darkolivegreen": [85, 107, 47], | ||
34 | + "darkorange": [255, 140, 0], | ||
35 | + "darkorchid": [153, 50, 204], | ||
36 | + "darkred": [139, 0, 0], | ||
37 | + "darksalmon": [233, 150, 122], | ||
38 | + "darkseagreen": [143, 188, 143], | ||
39 | + "darkslateblue": [72, 61, 139], | ||
40 | + "darkslategray": [47, 79, 79], | ||
41 | + "darkslategrey": [47, 79, 79], | ||
42 | + "darkturquoise": [0, 206, 209], | ||
43 | + "darkviolet": [148, 0, 211], | ||
44 | + "deeppink": [255, 20, 147], | ||
45 | + "deepskyblue": [0, 191, 255], | ||
46 | + "dimgray": [105, 105, 105], | ||
47 | + "dimgrey": [105, 105, 105], | ||
48 | + "dodgerblue": [30, 144, 255], | ||
49 | + "firebrick": [178, 34, 34], | ||
50 | + "floralwhite": [255, 250, 240], | ||
51 | + "forestgreen": [34, 139, 34], | ||
52 | + "fuchsia": [255, 0, 255], | ||
53 | + "gainsboro": [220, 220, 220], | ||
54 | + "ghostwhite": [248, 248, 255], | ||
55 | + "gold": [255, 215, 0], | ||
56 | + "goldenrod": [218, 165, 32], | ||
57 | + "gray": [128, 128, 128], | ||
58 | + "green": [0, 128, 0], | ||
59 | + "greenyellow": [173, 255, 47], | ||
60 | + "grey": [128, 128, 128], | ||
61 | + "honeydew": [240, 255, 240], | ||
62 | + "hotpink": [255, 105, 180], | ||
63 | + "indianred": [205, 92, 92], | ||
64 | + "indigo": [75, 0, 130], | ||
65 | + "ivory": [255, 255, 240], | ||
66 | + "khaki": [240, 230, 140], | ||
67 | + "lavender": [230, 230, 250], | ||
68 | + "lavenderblush": [255, 240, 245], | ||
69 | + "lawngreen": [124, 252, 0], | ||
70 | + "lemonchiffon": [255, 250, 205], | ||
71 | + "lightblue": [173, 216, 230], | ||
72 | + "lightcoral": [240, 128, 128], | ||
73 | + "lightcyan": [224, 255, 255], | ||
74 | + "lightgoldenrodyellow": [250, 250, 210], | ||
75 | + "lightgray": [211, 211, 211], | ||
76 | + "lightgreen": [144, 238, 144], | ||
77 | + "lightgrey": [211, 211, 211], | ||
78 | + "lightpink": [255, 182, 193], | ||
79 | + "lightsalmon": [255, 160, 122], | ||
80 | + "lightseagreen": [32, 178, 170], | ||
81 | + "lightskyblue": [135, 206, 250], | ||
82 | + "lightslategray": [119, 136, 153], | ||
83 | + "lightslategrey": [119, 136, 153], | ||
84 | + "lightsteelblue": [176, 196, 222], | ||
85 | + "lightyellow": [255, 255, 224], | ||
86 | + "lime": [0, 255, 0], | ||
87 | + "limegreen": [50, 205, 50], | ||
88 | + "linen": [250, 240, 230], | ||
89 | + "magenta": [255, 0, 255], | ||
90 | + "maroon": [128, 0, 0], | ||
91 | + "mediumaquamarine": [102, 205, 170], | ||
92 | + "mediumblue": [0, 0, 205], | ||
93 | + "mediumorchid": [186, 85, 211], | ||
94 | + "mediumpurple": [147, 112, 219], | ||
95 | + "mediumseagreen": [60, 179, 113], | ||
96 | + "mediumslateblue": [123, 104, 238], | ||
97 | + "mediumspringgreen": [0, 250, 154], | ||
98 | + "mediumturquoise": [72, 209, 204], | ||
99 | + "mediumvioletred": [199, 21, 133], | ||
100 | + "midnightblue": [25, 25, 112], | ||
101 | + "mintcream": [245, 255, 250], | ||
102 | + "mistyrose": [255, 228, 225], | ||
103 | + "moccasin": [255, 228, 181], | ||
104 | + "navajowhite": [255, 222, 173], | ||
105 | + "navy": [0, 0, 128], | ||
106 | + "oldlace": [253, 245, 230], | ||
107 | + "olive": [128, 128, 0], | ||
108 | + "olivedrab": [107, 142, 35], | ||
109 | + "orange": [255, 165, 0], | ||
110 | + "orangered": [255, 69, 0], | ||
111 | + "orchid": [218, 112, 214], | ||
112 | + "palegoldenrod": [238, 232, 170], | ||
113 | + "palegreen": [152, 251, 152], | ||
114 | + "paleturquoise": [175, 238, 238], | ||
115 | + "palevioletred": [219, 112, 147], | ||
116 | + "papayawhip": [255, 239, 213], | ||
117 | + "peachpuff": [255, 218, 185], | ||
118 | + "peru": [205, 133, 63], | ||
119 | + "pink": [255, 192, 203], | ||
120 | + "plum": [221, 160, 221], | ||
121 | + "powderblue": [176, 224, 230], | ||
122 | + "purple": [128, 0, 128], | ||
123 | + "rebeccapurple": [102, 51, 153], | ||
124 | + "red": [255, 0, 0], | ||
125 | + "rosybrown": [188, 143, 143], | ||
126 | + "royalblue": [65, 105, 225], | ||
127 | + "saddlebrown": [139, 69, 19], | ||
128 | + "salmon": [250, 128, 114], | ||
129 | + "sandybrown": [244, 164, 96], | ||
130 | + "seagreen": [46, 139, 87], | ||
131 | + "seashell": [255, 245, 238], | ||
132 | + "sienna": [160, 82, 45], | ||
133 | + "silver": [192, 192, 192], | ||
134 | + "skyblue": [135, 206, 235], | ||
135 | + "slateblue": [106, 90, 205], | ||
136 | + "slategray": [112, 128, 144], | ||
137 | + "slategrey": [112, 128, 144], | ||
138 | + "snow": [255, 250, 250], | ||
139 | + "springgreen": [0, 255, 127], | ||
140 | + "steelblue": [70, 130, 180], | ||
141 | + "tan": [210, 180, 140], | ||
142 | + "teal": [0, 128, 128], | ||
143 | + "thistle": [216, 191, 216], | ||
144 | + "tomato": [255, 99, 71], | ||
145 | + "turquoise": [64, 224, 208], | ||
146 | + "violet": [238, 130, 238], | ||
147 | + "wheat": [245, 222, 179], | ||
148 | + "white": [255, 255, 255], | ||
149 | + "whitesmoke": [245, 245, 245], | ||
150 | + "yellow": [255, 255, 0], | ||
151 | + "yellowgreen": [154, 205, 50] | ||
152 | +}; |
node_modules/color-name/package.json
0 → 100644
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "color-name@1.1.3", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "color-name@1.1.3", | ||
9 | + "_id": "color-name@1.1.3", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha1-p9BVi9icQveV3UIyj3QIMcpTvCU=", | ||
12 | + "_location": "/color-name", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "color-name@1.1.3", | ||
18 | + "name": "color-name", | ||
19 | + "escapedName": "color-name", | ||
20 | + "rawSpec": "1.1.3", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "1.1.3" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/color-convert" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/color-name/-/color-name-1.1.3.tgz", | ||
28 | + "_spec": "1.1.3", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "author": { | ||
31 | + "name": "DY", | ||
32 | + "email": "dfcreative@gmail.com" | ||
33 | + }, | ||
34 | + "bugs": { | ||
35 | + "url": "https://github.com/dfcreative/color-name/issues" | ||
36 | + }, | ||
37 | + "description": "A list of color names and its values", | ||
38 | + "homepage": "https://github.com/dfcreative/color-name", | ||
39 | + "keywords": [ | ||
40 | + "color-name", | ||
41 | + "color", | ||
42 | + "color-keyword", | ||
43 | + "keyword" | ||
44 | + ], | ||
45 | + "license": "MIT", | ||
46 | + "main": "index.js", | ||
47 | + "name": "color-name", | ||
48 | + "repository": { | ||
49 | + "type": "git", | ||
50 | + "url": "git+ssh://git@github.com/dfcreative/color-name.git" | ||
51 | + }, | ||
52 | + "scripts": { | ||
53 | + "test": "node test.js" | ||
54 | + }, | ||
55 | + "version": "1.1.3" | ||
56 | +} |
node_modules/color-name/test.js
0 → 100644
node_modules/concat-map/.travis.yml
0 → 100644
node_modules/concat-map/LICENSE
0 → 100644
1 | +This software is released under the MIT license: | ||
2 | + | ||
3 | +Permission is hereby granted, free of charge, to any person obtaining a copy of | ||
4 | +this software and associated documentation files (the "Software"), to deal in | ||
5 | +the Software without restriction, including without limitation the rights to | ||
6 | +use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of | ||
7 | +the Software, and to permit persons to whom the Software is furnished to do so, | ||
8 | +subject to the following conditions: | ||
9 | + | ||
10 | +The above copyright notice and this permission notice shall be included in all | ||
11 | +copies or substantial portions of the Software. | ||
12 | + | ||
13 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
14 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS | ||
15 | +FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR | ||
16 | +COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER | ||
17 | +IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN | ||
18 | +CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
node_modules/concat-map/README.markdown
0 → 100644
1 | +concat-map | ||
2 | +========== | ||
3 | + | ||
4 | +Concatenative mapdashery. | ||
5 | + | ||
6 | +[](http://ci.testling.com/substack/node-concat-map) | ||
7 | + | ||
8 | +[](http://travis-ci.org/substack/node-concat-map) | ||
9 | + | ||
10 | +example | ||
11 | +======= | ||
12 | + | ||
13 | +``` js | ||
14 | +var concatMap = require('concat-map'); | ||
15 | +var xs = [ 1, 2, 3, 4, 5, 6 ]; | ||
16 | +var ys = concatMap(xs, function (x) { | ||
17 | + return x % 2 ? [ x - 0.1, x, x + 0.1 ] : []; | ||
18 | +}); | ||
19 | +console.dir(ys); | ||
20 | +``` | ||
21 | + | ||
22 | +*** | ||
23 | + | ||
24 | +``` | ||
25 | +[ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ] | ||
26 | +``` | ||
27 | + | ||
28 | +methods | ||
29 | +======= | ||
30 | + | ||
31 | +``` js | ||
32 | +var concatMap = require('concat-map') | ||
33 | +``` | ||
34 | + | ||
35 | +concatMap(xs, fn) | ||
36 | +----------------- | ||
37 | + | ||
38 | +Return an array of concatenated elements by calling `fn(x, i)` for each element | ||
39 | +`x` and each index `i` in the array `xs`. | ||
40 | + | ||
41 | +When `fn(x, i)` returns an array, its result will be concatenated with the | ||
42 | +result array. If `fn(x, i)` returns anything else, that value will be pushed | ||
43 | +onto the end of the result array. | ||
44 | + | ||
45 | +install | ||
46 | +======= | ||
47 | + | ||
48 | +With [npm](http://npmjs.org) do: | ||
49 | + | ||
50 | +``` | ||
51 | +npm install concat-map | ||
52 | +``` | ||
53 | + | ||
54 | +license | ||
55 | +======= | ||
56 | + | ||
57 | +MIT | ||
58 | + | ||
59 | +notes | ||
60 | +===== | ||
61 | + | ||
62 | +This module was written while sitting high above the ground in a tree. |
node_modules/concat-map/example/map.js
0 → 100644
node_modules/concat-map/index.js
0 → 100644
1 | +module.exports = function (xs, fn) { | ||
2 | + var res = []; | ||
3 | + for (var i = 0; i < xs.length; i++) { | ||
4 | + var x = fn(xs[i], i); | ||
5 | + if (isArray(x)) res.push.apply(res, x); | ||
6 | + else res.push(x); | ||
7 | + } | ||
8 | + return res; | ||
9 | +}; | ||
10 | + | ||
11 | +var isArray = Array.isArray || function (xs) { | ||
12 | + return Object.prototype.toString.call(xs) === '[object Array]'; | ||
13 | +}; |
node_modules/concat-map/package.json
0 → 100644
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "concat-map@0.0.1", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "concat-map@0.0.1", | ||
9 | + "_id": "concat-map@0.0.1", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha1-2Klr13/Wjfd5OnMDajug1UBdR3s=", | ||
12 | + "_location": "/concat-map", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "concat-map@0.0.1", | ||
18 | + "name": "concat-map", | ||
19 | + "escapedName": "concat-map", | ||
20 | + "rawSpec": "0.0.1", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "0.0.1" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/brace-expansion" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/concat-map/-/concat-map-0.0.1.tgz", | ||
28 | + "_spec": "0.0.1", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "author": { | ||
31 | + "name": "James Halliday", | ||
32 | + "email": "mail@substack.net", | ||
33 | + "url": "http://substack.net" | ||
34 | + }, | ||
35 | + "bugs": { | ||
36 | + "url": "https://github.com/substack/node-concat-map/issues" | ||
37 | + }, | ||
38 | + "description": "concatenative mapdashery", | ||
39 | + "devDependencies": { | ||
40 | + "tape": "~2.4.0" | ||
41 | + }, | ||
42 | + "directories": { | ||
43 | + "example": "example", | ||
44 | + "test": "test" | ||
45 | + }, | ||
46 | + "homepage": "https://github.com/substack/node-concat-map#readme", | ||
47 | + "keywords": [ | ||
48 | + "concat", | ||
49 | + "concatMap", | ||
50 | + "map", | ||
51 | + "functional", | ||
52 | + "higher-order" | ||
53 | + ], | ||
54 | + "license": "MIT", | ||
55 | + "main": "index.js", | ||
56 | + "name": "concat-map", | ||
57 | + "repository": { | ||
58 | + "type": "git", | ||
59 | + "url": "git://github.com/substack/node-concat-map.git" | ||
60 | + }, | ||
61 | + "scripts": { | ||
62 | + "test": "tape test/*.js" | ||
63 | + }, | ||
64 | + "testling": { | ||
65 | + "files": "test/*.js", | ||
66 | + "browsers": { | ||
67 | + "ie": [ | ||
68 | + 6, | ||
69 | + 7, | ||
70 | + 8, | ||
71 | + 9 | ||
72 | + ], | ||
73 | + "ff": [ | ||
74 | + 3.5, | ||
75 | + 10, | ||
76 | + 15 | ||
77 | + ], | ||
78 | + "chrome": [ | ||
79 | + 10, | ||
80 | + 22 | ||
81 | + ], | ||
82 | + "safari": [ | ||
83 | + 5.1 | ||
84 | + ], | ||
85 | + "opera": [ | ||
86 | + 12 | ||
87 | + ] | ||
88 | + } | ||
89 | + }, | ||
90 | + "version": "0.0.1" | ||
91 | +} |
node_modules/concat-map/test/map.js
0 → 100644
1 | +var concatMap = require('../'); | ||
2 | +var test = require('tape'); | ||
3 | + | ||
4 | +test('empty or not', function (t) { | ||
5 | + var xs = [ 1, 2, 3, 4, 5, 6 ]; | ||
6 | + var ixes = []; | ||
7 | + var ys = concatMap(xs, function (x, ix) { | ||
8 | + ixes.push(ix); | ||
9 | + return x % 2 ? [ x - 0.1, x, x + 0.1 ] : []; | ||
10 | + }); | ||
11 | + t.same(ys, [ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ]); | ||
12 | + t.same(ixes, [ 0, 1, 2, 3, 4, 5 ]); | ||
13 | + t.end(); | ||
14 | +}); | ||
15 | + | ||
16 | +test('always something', function (t) { | ||
17 | + var xs = [ 'a', 'b', 'c', 'd' ]; | ||
18 | + var ys = concatMap(xs, function (x) { | ||
19 | + return x === 'b' ? [ 'B', 'B', 'B' ] : [ x ]; | ||
20 | + }); | ||
21 | + t.same(ys, [ 'a', 'B', 'B', 'B', 'c', 'd' ]); | ||
22 | + t.end(); | ||
23 | +}); | ||
24 | + | ||
25 | +test('scalars', function (t) { | ||
26 | + var xs = [ 'a', 'b', 'c', 'd' ]; | ||
27 | + var ys = concatMap(xs, function (x) { | ||
28 | + return x === 'b' ? [ 'B', 'B', 'B' ] : x; | ||
29 | + }); | ||
30 | + t.same(ys, [ 'a', 'B', 'B', 'B', 'c', 'd' ]); | ||
31 | + t.end(); | ||
32 | +}); | ||
33 | + | ||
34 | +test('undefs', function (t) { | ||
35 | + var xs = [ 'a', 'b', 'c', 'd' ]; | ||
36 | + var ys = concatMap(xs, function () {}); | ||
37 | + t.same(ys, [ undefined, undefined, undefined, undefined ]); | ||
38 | + t.end(); | ||
39 | +}); |
node_modules/core-util-is/LICENSE
0 → 100644
1 | +Copyright Node.js contributors. All rights reserved. | ||
2 | + | ||
3 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
4 | +of this software and associated documentation files (the "Software"), to | ||
5 | +deal in the Software without restriction, including without limitation the | ||
6 | +rights to use, copy, modify, merge, publish, distribute, sublicense, and/or | ||
7 | +sell copies of the Software, and to permit persons to whom the Software is | ||
8 | +furnished to do so, subject to the following conditions: | ||
9 | + | ||
10 | +The above copyright notice and this permission notice shall be included in | ||
11 | +all copies or substantial portions of the Software. | ||
12 | + | ||
13 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
14 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
15 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
16 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
17 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING | ||
18 | +FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS | ||
19 | +IN THE SOFTWARE. |
node_modules/core-util-is/README.md
0 → 100644
node_modules/core-util-is/lib/util.js
0 → 100644
1 | +// Copyright Joyent, Inc. and other Node contributors. | ||
2 | +// | ||
3 | +// Permission is hereby granted, free of charge, to any person obtaining a | ||
4 | +// copy of this software and associated documentation files (the | ||
5 | +// "Software"), to deal in the Software without restriction, including | ||
6 | +// without limitation the rights to use, copy, modify, merge, publish, | ||
7 | +// distribute, sublicense, and/or sell copies of the Software, and to permit | ||
8 | +// persons to whom the Software is furnished to do so, subject to the | ||
9 | +// following conditions: | ||
10 | +// | ||
11 | +// The above copyright notice and this permission notice shall be included | ||
12 | +// in all copies or substantial portions of the Software. | ||
13 | +// | ||
14 | +// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS | ||
15 | +// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF | ||
16 | +// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN | ||
17 | +// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, | ||
18 | +// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR | ||
19 | +// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE | ||
20 | +// USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
21 | + | ||
22 | +// NOTE: These type checking functions intentionally don't use `instanceof` | ||
23 | +// because it is fragile and can be easily faked with `Object.create()`. | ||
24 | + | ||
25 | +function isArray(arg) { | ||
26 | + if (Array.isArray) { | ||
27 | + return Array.isArray(arg); | ||
28 | + } | ||
29 | + return objectToString(arg) === '[object Array]'; | ||
30 | +} | ||
31 | +exports.isArray = isArray; | ||
32 | + | ||
33 | +function isBoolean(arg) { | ||
34 | + return typeof arg === 'boolean'; | ||
35 | +} | ||
36 | +exports.isBoolean = isBoolean; | ||
37 | + | ||
38 | +function isNull(arg) { | ||
39 | + return arg === null; | ||
40 | +} | ||
41 | +exports.isNull = isNull; | ||
42 | + | ||
43 | +function isNullOrUndefined(arg) { | ||
44 | + return arg == null; | ||
45 | +} | ||
46 | +exports.isNullOrUndefined = isNullOrUndefined; | ||
47 | + | ||
48 | +function isNumber(arg) { | ||
49 | + return typeof arg === 'number'; | ||
50 | +} | ||
51 | +exports.isNumber = isNumber; | ||
52 | + | ||
53 | +function isString(arg) { | ||
54 | + return typeof arg === 'string'; | ||
55 | +} | ||
56 | +exports.isString = isString; | ||
57 | + | ||
58 | +function isSymbol(arg) { | ||
59 | + return typeof arg === 'symbol'; | ||
60 | +} | ||
61 | +exports.isSymbol = isSymbol; | ||
62 | + | ||
63 | +function isUndefined(arg) { | ||
64 | + return arg === void 0; | ||
65 | +} | ||
66 | +exports.isUndefined = isUndefined; | ||
67 | + | ||
68 | +function isRegExp(re) { | ||
69 | + return objectToString(re) === '[object RegExp]'; | ||
70 | +} | ||
71 | +exports.isRegExp = isRegExp; | ||
72 | + | ||
73 | +function isObject(arg) { | ||
74 | + return typeof arg === 'object' && arg !== null; | ||
75 | +} | ||
76 | +exports.isObject = isObject; | ||
77 | + | ||
78 | +function isDate(d) { | ||
79 | + return objectToString(d) === '[object Date]'; | ||
80 | +} | ||
81 | +exports.isDate = isDate; | ||
82 | + | ||
83 | +function isError(e) { | ||
84 | + return (objectToString(e) === '[object Error]' || e instanceof Error); | ||
85 | +} | ||
86 | +exports.isError = isError; | ||
87 | + | ||
88 | +function isFunction(arg) { | ||
89 | + return typeof arg === 'function'; | ||
90 | +} | ||
91 | +exports.isFunction = isFunction; | ||
92 | + | ||
93 | +function isPrimitive(arg) { | ||
94 | + return arg === null || | ||
95 | + typeof arg === 'boolean' || | ||
96 | + typeof arg === 'number' || | ||
97 | + typeof arg === 'string' || | ||
98 | + typeof arg === 'symbol' || // ES6 symbol | ||
99 | + typeof arg === 'undefined'; | ||
100 | +} | ||
101 | +exports.isPrimitive = isPrimitive; | ||
102 | + | ||
103 | +exports.isBuffer = require('buffer').Buffer.isBuffer; | ||
104 | + | ||
105 | +function objectToString(o) { | ||
106 | + return Object.prototype.toString.call(o); | ||
107 | +} |
node_modules/core-util-is/package.json
0 → 100644
1 | +{ | ||
2 | + "_from": "core-util-is@~1.0.0", | ||
3 | + "_id": "core-util-is@1.0.3", | ||
4 | + "_inBundle": false, | ||
5 | + "_integrity": "sha512-ZQBvi1DcpJ4GDqanjucZ2Hj3wEO5pZDS89BWbkcrvdxksJorwUDDZamX9ldFkp9aw2lmBDLgkObEA4DWNJ9FYQ==", | ||
6 | + "_location": "/core-util-is", | ||
7 | + "_phantomChildren": {}, | ||
8 | + "_requested": { | ||
9 | + "type": "range", | ||
10 | + "registry": true, | ||
11 | + "raw": "core-util-is@~1.0.0", | ||
12 | + "name": "core-util-is", | ||
13 | + "escapedName": "core-util-is", | ||
14 | + "rawSpec": "~1.0.0", | ||
15 | + "saveSpec": null, | ||
16 | + "fetchSpec": "~1.0.0" | ||
17 | + }, | ||
18 | + "_requiredBy": [ | ||
19 | + "/readable-stream" | ||
20 | + ], | ||
21 | + "_resolved": "https://registry.npmjs.org/core-util-is/-/core-util-is-1.0.3.tgz", | ||
22 | + "_shasum": "a6042d3634c2b27e9328f837b965fac83808db85", | ||
23 | + "_spec": "core-util-is@~1.0.0", | ||
24 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사\\node_modules\\readable-stream", | ||
25 | + "author": { | ||
26 | + "name": "Isaac Z. Schlueter", | ||
27 | + "email": "i@izs.me", | ||
28 | + "url": "http://blog.izs.me/" | ||
29 | + }, | ||
30 | + "bugs": { | ||
31 | + "url": "https://github.com/isaacs/core-util-is/issues" | ||
32 | + }, | ||
33 | + "bundleDependencies": false, | ||
34 | + "deprecated": false, | ||
35 | + "description": "The `util.is*` functions introduced in Node v0.12.", | ||
36 | + "devDependencies": { | ||
37 | + "tap": "^15.0.9" | ||
38 | + }, | ||
39 | + "files": [ | ||
40 | + "lib" | ||
41 | + ], | ||
42 | + "homepage": "https://github.com/isaacs/core-util-is#readme", | ||
43 | + "keywords": [ | ||
44 | + "util", | ||
45 | + "isBuffer", | ||
46 | + "isArray", | ||
47 | + "isNumber", | ||
48 | + "isString", | ||
49 | + "isRegExp", | ||
50 | + "isThis", | ||
51 | + "isThat", | ||
52 | + "polyfill" | ||
53 | + ], | ||
54 | + "license": "MIT", | ||
55 | + "main": "lib/util.js", | ||
56 | + "name": "core-util-is", | ||
57 | + "repository": { | ||
58 | + "type": "git", | ||
59 | + "url": "git://github.com/isaacs/core-util-is.git" | ||
60 | + }, | ||
61 | + "scripts": { | ||
62 | + "postversion": "npm publish", | ||
63 | + "prepublishOnly": "git push origin --follow-tags", | ||
64 | + "preversion": "npm test", | ||
65 | + "test": "tap test.js" | ||
66 | + }, | ||
67 | + "version": "1.0.3" | ||
68 | +} |
node_modules/ejs/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/ejs/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/ejs/bin/cli.js
0 → 100644
1 | +#!/usr/bin/env node | ||
2 | +/* | ||
3 | + * EJS Embedded JavaScript templates | ||
4 | + * Copyright 2112 Matthew Eernisse (mde@fleegix.org) | ||
5 | + * | ||
6 | + * Licensed under the Apache License, Version 2.0 (the "License"); | ||
7 | + * you may not use this file except in compliance with the License. | ||
8 | + * You may obtain a copy of the License at | ||
9 | + * | ||
10 | + * http://www.apache.org/licenses/LICENSE-2.0 | ||
11 | + * | ||
12 | + * Unless required by applicable law or agreed to in writing, software | ||
13 | + * distributed under the License is distributed on an "AS IS" BASIS, | ||
14 | + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
15 | + * See the License for the specific language governing permissions and | ||
16 | + * limitations under the License. | ||
17 | + * | ||
18 | +*/ | ||
19 | + | ||
20 | + | ||
21 | +let program = require('jake').program; | ||
22 | +delete global.jake; // NO NOT WANT | ||
23 | +program.setTaskNames = function (n) { this.taskNames = n; }; | ||
24 | + | ||
25 | +let ejs = require('../lib/ejs'); | ||
26 | +let { hyphenToCamel } = require('../lib/utils'); | ||
27 | +let fs = require('fs'); | ||
28 | +let args = process.argv.slice(2); | ||
29 | +let usage = fs.readFileSync(`${__dirname}/../usage.txt`).toString(); | ||
30 | + | ||
31 | +const CLI_OPTS = [ | ||
32 | + { full: 'output-file', | ||
33 | + abbr: 'o', | ||
34 | + expectValue: true, | ||
35 | + }, | ||
36 | + { full: 'data-file', | ||
37 | + abbr: 'f', | ||
38 | + expectValue: true, | ||
39 | + }, | ||
40 | + { full: 'data-input', | ||
41 | + abbr: 'i', | ||
42 | + expectValue: true, | ||
43 | + }, | ||
44 | + { full: 'delimiter', | ||
45 | + abbr: 'm', | ||
46 | + expectValue: true, | ||
47 | + passThrough: true, | ||
48 | + }, | ||
49 | + { full: 'open-delimiter', | ||
50 | + abbr: 'p', | ||
51 | + expectValue: true, | ||
52 | + passThrough: true, | ||
53 | + }, | ||
54 | + { full: 'close-delimiter', | ||
55 | + abbr: 'c', | ||
56 | + expectValue: true, | ||
57 | + passThrough: true, | ||
58 | + }, | ||
59 | + { full: 'strict', | ||
60 | + abbr: 's', | ||
61 | + expectValue: false, | ||
62 | + allowValue: false, | ||
63 | + passThrough: true, | ||
64 | + }, | ||
65 | + { full: 'no-with', | ||
66 | + abbr: 'n', | ||
67 | + expectValue: false, | ||
68 | + allowValue: false, | ||
69 | + }, | ||
70 | + { full: 'locals-name', | ||
71 | + abbr: 'l', | ||
72 | + expectValue: true, | ||
73 | + passThrough: true, | ||
74 | + }, | ||
75 | + { full: 'rm-whitespace', | ||
76 | + abbr: 'w', | ||
77 | + expectValue: false, | ||
78 | + allowValue: false, | ||
79 | + passThrough: true, | ||
80 | + }, | ||
81 | + { full: 'debug', | ||
82 | + abbr: 'd', | ||
83 | + expectValue: false, | ||
84 | + allowValue: false, | ||
85 | + passThrough: true, | ||
86 | + }, | ||
87 | + { full: 'help', | ||
88 | + abbr: 'h', | ||
89 | + passThrough: true, | ||
90 | + }, | ||
91 | + { full: 'version', | ||
92 | + abbr: 'V', | ||
93 | + passThrough: true, | ||
94 | + }, | ||
95 | + // Alias lowercase v | ||
96 | + { full: 'version', | ||
97 | + abbr: 'v', | ||
98 | + passThrough: true, | ||
99 | + }, | ||
100 | +]; | ||
101 | + | ||
102 | +let preempts = { | ||
103 | + version: function () { | ||
104 | + program.die(ejs.VERSION); | ||
105 | + }, | ||
106 | + help: function () { | ||
107 | + program.die(usage); | ||
108 | + } | ||
109 | +}; | ||
110 | + | ||
111 | +let stdin = ''; | ||
112 | +process.stdin.setEncoding('utf8'); | ||
113 | +process.stdin.on('readable', () => { | ||
114 | + let chunk; | ||
115 | + while ((chunk = process.stdin.read()) !== null) { | ||
116 | + stdin += chunk; | ||
117 | + } | ||
118 | +}); | ||
119 | + | ||
120 | +function run() { | ||
121 | + | ||
122 | + program.availableOpts = CLI_OPTS; | ||
123 | + program.parseArgs(args); | ||
124 | + | ||
125 | + let templatePath = program.taskNames[0]; | ||
126 | + let pVals = program.envVars; | ||
127 | + let pOpts = {}; | ||
128 | + | ||
129 | + for (let p in program.opts) { | ||
130 | + let name = hyphenToCamel(p); | ||
131 | + pOpts[name] = program.opts[p]; | ||
132 | + } | ||
133 | + | ||
134 | + let opts = {}; | ||
135 | + let vals = {}; | ||
136 | + | ||
137 | + // Same-named 'passthrough' opts | ||
138 | + CLI_OPTS.forEach((opt) => { | ||
139 | + let optName = hyphenToCamel(opt.full); | ||
140 | + if (opt.passThrough && typeof pOpts[optName] != 'undefined') { | ||
141 | + opts[optName] = pOpts[optName]; | ||
142 | + } | ||
143 | + }); | ||
144 | + | ||
145 | + // Bail out for help/version | ||
146 | + for (let p in opts) { | ||
147 | + if (preempts[p]) { | ||
148 | + return preempts[p](); | ||
149 | + } | ||
150 | + } | ||
151 | + | ||
152 | + // Default to having views relative from the current working directory | ||
153 | + opts.views = ['.']; | ||
154 | + | ||
155 | + // Ensure there's a template to render | ||
156 | + if (!templatePath) { | ||
157 | + throw new Error('Please provide a template path. (Run ejs -h for help)'); | ||
158 | + } | ||
159 | + | ||
160 | + if (opts.strict) { | ||
161 | + pOpts.noWith = true; | ||
162 | + } | ||
163 | + if (pOpts.noWith) { | ||
164 | + opts._with = false; | ||
165 | + } | ||
166 | + | ||
167 | + // Grab and parse any input data, in order of precedence: | ||
168 | + // 1. Stdin | ||
169 | + // 2. CLI arg via -i | ||
170 | + // 3. Data file via -f | ||
171 | + // Any individual vals passed at the end (e.g., foo=bar) will override | ||
172 | + // any vals previously set | ||
173 | + let input; | ||
174 | + let err = new Error('Please do not pass data multiple ways. Pick one of stdin, -f, or -i.'); | ||
175 | + if (stdin) { | ||
176 | + input = stdin; | ||
177 | + } | ||
178 | + else if (pOpts.dataInput) { | ||
179 | + if (input) { | ||
180 | + throw err; | ||
181 | + } | ||
182 | + input = decodeURIComponent(pOpts.dataInput); | ||
183 | + } | ||
184 | + else if (pOpts.dataFile) { | ||
185 | + if (input) { | ||
186 | + throw err; | ||
187 | + } | ||
188 | + input = fs.readFileSync(pOpts.dataFile).toString(); | ||
189 | + } | ||
190 | + | ||
191 | + if (input) { | ||
192 | + vals = JSON.parse(input); | ||
193 | + } | ||
194 | + | ||
195 | + // Override / set any individual values passed from the command line | ||
196 | + for (let p in pVals) { | ||
197 | + vals[p] = pVals[p]; | ||
198 | + } | ||
199 | + | ||
200 | + let template = fs.readFileSync(templatePath).toString(); | ||
201 | + let output = ejs.render(template, vals, opts); | ||
202 | + if (pOpts.outputFile) { | ||
203 | + fs.writeFileSync(pOpts.outputFile, output); | ||
204 | + } | ||
205 | + else { | ||
206 | + process.stdout.write(output); | ||
207 | + } | ||
208 | + process.exit(); | ||
209 | +} | ||
210 | + | ||
211 | +// Defer execution so that stdin can be read if necessary | ||
212 | +setImmediate(run); |
node_modules/ejs/ejs.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/ejs/ejs.min.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/ejs/jakefile.js
0 → 100644
1 | +var fs = require('fs'); | ||
2 | +var execSync = require('child_process').execSync; | ||
3 | +var exec = function (cmd) { | ||
4 | + execSync(cmd, {stdio: 'inherit'}); | ||
5 | +}; | ||
6 | + | ||
7 | +/* global jake, task, desc, publishTask */ | ||
8 | + | ||
9 | +task('build', ['lint', 'clean', 'browserify', 'minify'], function () { | ||
10 | + console.log('Build completed.'); | ||
11 | +}); | ||
12 | + | ||
13 | +desc('Cleans browerified/minified files and package files'); | ||
14 | +task('clean', ['clobber'], function () { | ||
15 | + jake.rmRf('./ejs.js'); | ||
16 | + jake.rmRf('./ejs.min.js'); | ||
17 | + console.log('Cleaned up compiled files.'); | ||
18 | +}); | ||
19 | + | ||
20 | +desc('Lints the source code'); | ||
21 | +task('lint', ['clean'], function () { | ||
22 | + exec('./node_modules/.bin/eslint "**/*.js"'); | ||
23 | + console.log('Linting completed.'); | ||
24 | +}); | ||
25 | + | ||
26 | +task('browserify', function () { | ||
27 | + exec('./node_modules/browserify/bin/cmd.js --standalone ejs lib/ejs.js > ejs.js'); | ||
28 | + console.log('Browserification completed.'); | ||
29 | +}); | ||
30 | + | ||
31 | +task('minify', function () { | ||
32 | + exec('./node_modules/uglify-js/bin/uglifyjs ejs.js > ejs.min.js'); | ||
33 | + console.log('Minification completed.'); | ||
34 | +}); | ||
35 | + | ||
36 | +desc('Generates the EJS API docs for the public API'); | ||
37 | +task('doc', function () { | ||
38 | + jake.rmRf('out'); | ||
39 | + exec('./node_modules/.bin/jsdoc --verbose -c jsdoc.json lib/* docs/jsdoc/*'); | ||
40 | + console.log('Documentation generated in ./out.'); | ||
41 | +}); | ||
42 | + | ||
43 | +desc('Generates the EJS API docs for the public and private API'); | ||
44 | +task('devdoc', function () { | ||
45 | + jake.rmRf('out'); | ||
46 | + exec('./node_modules/.bin/jsdoc --verbose -p -c jsdoc.json lib/* docs/jsdoc/*'); | ||
47 | + console.log('Documentation generated in ./out.'); | ||
48 | +}); | ||
49 | + | ||
50 | +desc('Publishes the EJS API docs'); | ||
51 | +task('docPublish', ['doc'], function () { | ||
52 | + fs.writeFileSync('out/CNAME', 'api.ejs.co'); | ||
53 | + console.log('Pushing docs to gh-pages...'); | ||
54 | + exec('./node_modules/.bin/git-directory-deploy --directory out/'); | ||
55 | + console.log('Docs published to gh-pages.'); | ||
56 | +}); | ||
57 | + | ||
58 | +desc('Runs the EJS test suite'); | ||
59 | +task('test', ['lint'], function () { | ||
60 | + exec('./node_modules/.bin/mocha'); | ||
61 | +}); | ||
62 | + | ||
63 | +publishTask('ejs', ['build'], function () { | ||
64 | + this.packageFiles.include([ | ||
65 | + 'jakefile.js', | ||
66 | + 'README.md', | ||
67 | + 'LICENSE', | ||
68 | + 'package.json', | ||
69 | + 'ejs.js', | ||
70 | + 'ejs.min.js', | ||
71 | + 'lib/**', | ||
72 | + 'bin/**', | ||
73 | + 'usage.txt' | ||
74 | + ]); | ||
75 | +}); | ||
76 | + | ||
77 | +jake.Task.publish.on('complete', function () { | ||
78 | + console.log('Updating hosted docs...'); | ||
79 | + console.log('If this fails, run jake docPublish to re-try.'); | ||
80 | + jake.Task.docPublish.invoke(); | ||
81 | +}); |
node_modules/ejs/lib/ejs.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/ejs/lib/utils.js
0 → 100644
1 | +/* | ||
2 | + * EJS Embedded JavaScript templates | ||
3 | + * Copyright 2112 Matthew Eernisse (mde@fleegix.org) | ||
4 | + * | ||
5 | + * Licensed under the Apache License, Version 2.0 (the "License"); | ||
6 | + * you may not use this file except in compliance with the License. | ||
7 | + * You may obtain a copy of the License at | ||
8 | + * | ||
9 | + * http://www.apache.org/licenses/LICENSE-2.0 | ||
10 | + * | ||
11 | + * Unless required by applicable law or agreed to in writing, software | ||
12 | + * distributed under the License is distributed on an "AS IS" BASIS, | ||
13 | + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
14 | + * See the License for the specific language governing permissions and | ||
15 | + * limitations under the License. | ||
16 | + * | ||
17 | +*/ | ||
18 | + | ||
19 | +/** | ||
20 | + * Private utility functions | ||
21 | + * @module utils | ||
22 | + * @private | ||
23 | + */ | ||
24 | + | ||
25 | +'use strict'; | ||
26 | + | ||
27 | +var regExpChars = /[|\\{}()[\]^$+*?.]/g; | ||
28 | + | ||
29 | +/** | ||
30 | + * Escape characters reserved in regular expressions. | ||
31 | + * | ||
32 | + * If `string` is `undefined` or `null`, the empty string is returned. | ||
33 | + * | ||
34 | + * @param {String} string Input string | ||
35 | + * @return {String} Escaped string | ||
36 | + * @static | ||
37 | + * @private | ||
38 | + */ | ||
39 | +exports.escapeRegExpChars = function (string) { | ||
40 | + // istanbul ignore if | ||
41 | + if (!string) { | ||
42 | + return ''; | ||
43 | + } | ||
44 | + return String(string).replace(regExpChars, '\\$&'); | ||
45 | +}; | ||
46 | + | ||
47 | +var _ENCODE_HTML_RULES = { | ||
48 | + '&': '&', | ||
49 | + '<': '<', | ||
50 | + '>': '>', | ||
51 | + '"': '"', | ||
52 | + "'": ''' | ||
53 | +}; | ||
54 | +var _MATCH_HTML = /[&<>'"]/g; | ||
55 | + | ||
56 | +function encode_char(c) { | ||
57 | + return _ENCODE_HTML_RULES[c] || c; | ||
58 | +} | ||
59 | + | ||
60 | +/** | ||
61 | + * Stringified version of constants used by {@link module:utils.escapeXML}. | ||
62 | + * | ||
63 | + * It is used in the process of generating {@link ClientFunction}s. | ||
64 | + * | ||
65 | + * @readonly | ||
66 | + * @type {String} | ||
67 | + */ | ||
68 | + | ||
69 | +var escapeFuncStr = | ||
70 | + 'var _ENCODE_HTML_RULES = {\n' | ||
71 | ++ ' "&": "&"\n' | ||
72 | ++ ' , "<": "<"\n' | ||
73 | ++ ' , ">": ">"\n' | ||
74 | ++ ' , \'"\': """\n' | ||
75 | ++ ' , "\'": "'"\n' | ||
76 | ++ ' }\n' | ||
77 | ++ ' , _MATCH_HTML = /[&<>\'"]/g;\n' | ||
78 | ++ 'function encode_char(c) {\n' | ||
79 | ++ ' return _ENCODE_HTML_RULES[c] || c;\n' | ||
80 | ++ '};\n'; | ||
81 | + | ||
82 | +/** | ||
83 | + * Escape characters reserved in XML. | ||
84 | + * | ||
85 | + * If `markup` is `undefined` or `null`, the empty string is returned. | ||
86 | + * | ||
87 | + * @implements {EscapeCallback} | ||
88 | + * @param {String} markup Input string | ||
89 | + * @return {String} Escaped string | ||
90 | + * @static | ||
91 | + * @private | ||
92 | + */ | ||
93 | + | ||
94 | +exports.escapeXML = function (markup) { | ||
95 | + return markup == undefined | ||
96 | + ? '' | ||
97 | + : String(markup) | ||
98 | + .replace(_MATCH_HTML, encode_char); | ||
99 | +}; | ||
100 | +exports.escapeXML.toString = function () { | ||
101 | + return Function.prototype.toString.call(this) + ';\n' + escapeFuncStr; | ||
102 | +}; | ||
103 | + | ||
104 | +/** | ||
105 | + * Naive copy of properties from one object to another. | ||
106 | + * Does not recurse into non-scalar properties | ||
107 | + * Does not check to see if the property has a value before copying | ||
108 | + * | ||
109 | + * @param {Object} to Destination object | ||
110 | + * @param {Object} from Source object | ||
111 | + * @return {Object} Destination object | ||
112 | + * @static | ||
113 | + * @private | ||
114 | + */ | ||
115 | +exports.shallowCopy = function (to, from) { | ||
116 | + from = from || {}; | ||
117 | + for (var p in from) { | ||
118 | + to[p] = from[p]; | ||
119 | + } | ||
120 | + return to; | ||
121 | +}; | ||
122 | + | ||
123 | +/** | ||
124 | + * Naive copy of a list of key names, from one object to another. | ||
125 | + * Only copies property if it is actually defined | ||
126 | + * Does not recurse into non-scalar properties | ||
127 | + * | ||
128 | + * @param {Object} to Destination object | ||
129 | + * @param {Object} from Source object | ||
130 | + * @param {Array} list List of properties to copy | ||
131 | + * @return {Object} Destination object | ||
132 | + * @static | ||
133 | + * @private | ||
134 | + */ | ||
135 | +exports.shallowCopyFromList = function (to, from, list) { | ||
136 | + for (var i = 0; i < list.length; i++) { | ||
137 | + var p = list[i]; | ||
138 | + if (typeof from[p] != 'undefined') { | ||
139 | + to[p] = from[p]; | ||
140 | + } | ||
141 | + } | ||
142 | + return to; | ||
143 | +}; | ||
144 | + | ||
145 | +/** | ||
146 | + * Simple in-process cache implementation. Does not implement limits of any | ||
147 | + * sort. | ||
148 | + * | ||
149 | + * @implements {Cache} | ||
150 | + * @static | ||
151 | + * @private | ||
152 | + */ | ||
153 | +exports.cache = { | ||
154 | + _data: {}, | ||
155 | + set: function (key, val) { | ||
156 | + this._data[key] = val; | ||
157 | + }, | ||
158 | + get: function (key) { | ||
159 | + return this._data[key]; | ||
160 | + }, | ||
161 | + remove: function (key) { | ||
162 | + delete this._data[key]; | ||
163 | + }, | ||
164 | + reset: function () { | ||
165 | + this._data = {}; | ||
166 | + } | ||
167 | +}; | ||
168 | + | ||
169 | +/** | ||
170 | + * Transforms hyphen case variable into camel case. | ||
171 | + * | ||
172 | + * @param {String} string Hyphen case string | ||
173 | + * @return {String} Camel case string | ||
174 | + * @static | ||
175 | + * @private | ||
176 | + */ | ||
177 | +exports.hyphenToCamel = function (str) { | ||
178 | + return str.replace(/-[a-z]/g, function (match) { return match[1].toUpperCase(); }); | ||
179 | +}; |
node_modules/ejs/package.json
0 → 100644
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "ejs@3.1.6", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "ejs@3.1.6", | ||
9 | + "_id": "ejs@3.1.6", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha512-9lt9Zse4hPucPkoP7FHDF0LQAlGyF9JVpnClFLFH3aSSbxmyoqINRpp/9wePWJTUl4KOQwRL72Iw3InHPDkoGw==", | ||
12 | + "_location": "/ejs", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "ejs@3.1.6", | ||
18 | + "name": "ejs", | ||
19 | + "escapedName": "ejs", | ||
20 | + "rawSpec": "3.1.6", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "3.1.6" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/ejs/-/ejs-3.1.6.tgz", | ||
28 | + "_spec": "3.1.6", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "author": { | ||
31 | + "name": "Matthew Eernisse", | ||
32 | + "email": "mde@fleegix.org", | ||
33 | + "url": "http://fleegix.org" | ||
34 | + }, | ||
35 | + "bin": { | ||
36 | + "ejs": "bin/cli.js" | ||
37 | + }, | ||
38 | + "bugs": { | ||
39 | + "url": "https://github.com/mde/ejs/issues" | ||
40 | + }, | ||
41 | + "dependencies": { | ||
42 | + "jake": "^10.6.1" | ||
43 | + }, | ||
44 | + "description": "Embedded JavaScript templates", | ||
45 | + "devDependencies": { | ||
46 | + "browserify": "^16.5.1", | ||
47 | + "eslint": "^6.8.0", | ||
48 | + "git-directory-deploy": "^1.5.1", | ||
49 | + "jsdoc": "^3.6.4", | ||
50 | + "lru-cache": "^4.0.1", | ||
51 | + "mocha": "^7.1.1", | ||
52 | + "uglify-js": "^3.3.16" | ||
53 | + }, | ||
54 | + "engines": { | ||
55 | + "node": ">=0.10.0" | ||
56 | + }, | ||
57 | + "homepage": "https://github.com/mde/ejs", | ||
58 | + "jsdelivr": "ejs.min.js", | ||
59 | + "keywords": [ | ||
60 | + "template", | ||
61 | + "engine", | ||
62 | + "ejs" | ||
63 | + ], | ||
64 | + "license": "Apache-2.0", | ||
65 | + "main": "./lib/ejs.js", | ||
66 | + "name": "ejs", | ||
67 | + "repository": { | ||
68 | + "type": "git", | ||
69 | + "url": "git://github.com/mde/ejs.git" | ||
70 | + }, | ||
71 | + "scripts": { | ||
72 | + "test": "mocha" | ||
73 | + }, | ||
74 | + "unpkg": "ejs.min.js", | ||
75 | + "version": "3.1.6" | ||
76 | +} |
node_modules/ejs/usage.txt
0 → 100644
1 | +EJS Embedded JavaScript templates | ||
2 | +{Usage}: ejs [options ...] template-file [data variables ...] | ||
3 | + | ||
4 | +{Options}: | ||
5 | + -o, --output-file FILE Write the rendered output to FILE rather than stdout. | ||
6 | + -f, --data-file FILE Must be JSON-formatted. Use parsed input from FILE as data for rendering. | ||
7 | + -i, --data-input STRING Must be JSON-formatted and URI-encoded. Use parsed input from STRING as data for rendering. | ||
8 | + -m, --delimiter CHARACTER Use CHARACTER with angle brackets for open/close (defaults to %). | ||
9 | + -p, --open-delimiter CHARACTER Use CHARACTER instead of left angle bracket to open. | ||
10 | + -c, --close-delimiter CHARACTER Use CHARACTER instead of right angle bracket to close. | ||
11 | + -s, --strict When set to `true`, generated function is in strict mode | ||
12 | + -n --no-with Use 'locals' object for vars rather than using `with` (implies --strict). | ||
13 | + -l --locals-name Name to use for the object storing local variables when not using `with`. | ||
14 | + -w --rm-whitespace Remove all safe-to-remove whitespace, including leading and trailing whitespace. | ||
15 | + -d --debug Outputs generated function body | ||
16 | + -h, --help Display this help message. | ||
17 | + -V/v, --version Display the EJS version. | ||
18 | + | ||
19 | +{Examples}: | ||
20 | + ejs -m $ ./test/fixtures/user.ejs -f ./user_data.json | ||
21 | + ejs -m $ ./test/fixtures/user.ejs name=Lerxst | ||
22 | + ejs -p [ -c ] ./template_file.ejs -o ./output.html | ||
23 | + ejs -n -l _ ./some_template.ejs -f ./data_file.json | ||
24 | + ejs -w ./template_with_whitspace.ejs -o ./output_file.html |
node_modules/escape-string-regexp/index.js
0 → 100644
node_modules/escape-string-regexp/license
0 → 100644
1 | +The MIT License (MIT) | ||
2 | + | ||
3 | +Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) | ||
4 | + | ||
5 | +Permission is hereby granted, free of charge, to any person obtaining a copy | ||
6 | +of this software and associated documentation files (the "Software"), to deal | ||
7 | +in the Software without restriction, including without limitation the rights | ||
8 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
9 | +copies of the Software, and to permit persons to whom the Software is | ||
10 | +furnished to do so, subject to the following conditions: | ||
11 | + | ||
12 | +The above copyright notice and this permission notice shall be included in | ||
13 | +all copies or substantial portions of the Software. | ||
14 | + | ||
15 | +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
16 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
17 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
18 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
19 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
20 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN | ||
21 | +THE SOFTWARE. |
1 | +{ | ||
2 | + "_args": [ | ||
3 | + [ | ||
4 | + "escape-string-regexp@1.0.5", | ||
5 | + "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사" | ||
6 | + ] | ||
7 | + ], | ||
8 | + "_from": "escape-string-regexp@1.0.5", | ||
9 | + "_id": "escape-string-regexp@1.0.5", | ||
10 | + "_inBundle": false, | ||
11 | + "_integrity": "sha1-G2HAViGQqN/2rjuyzwIAyhMLhtQ=", | ||
12 | + "_location": "/escape-string-regexp", | ||
13 | + "_phantomChildren": {}, | ||
14 | + "_requested": { | ||
15 | + "type": "version", | ||
16 | + "registry": true, | ||
17 | + "raw": "escape-string-regexp@1.0.5", | ||
18 | + "name": "escape-string-regexp", | ||
19 | + "escapedName": "escape-string-regexp", | ||
20 | + "rawSpec": "1.0.5", | ||
21 | + "saveSpec": null, | ||
22 | + "fetchSpec": "1.0.5" | ||
23 | + }, | ||
24 | + "_requiredBy": [ | ||
25 | + "/chalk" | ||
26 | + ], | ||
27 | + "_resolved": "https://registry.npmjs.org/escape-string-regexp/-/escape-string-regexp-1.0.5.tgz", | ||
28 | + "_spec": "1.0.5", | ||
29 | + "_where": "C:\\Users\\KoMoGoon\\Desktop\\실전모의고사", | ||
30 | + "author": { | ||
31 | + "name": "Sindre Sorhus", | ||
32 | + "email": "sindresorhus@gmail.com", | ||
33 | + "url": "sindresorhus.com" | ||
34 | + }, | ||
35 | + "bugs": { | ||
36 | + "url": "https://github.com/sindresorhus/escape-string-regexp/issues" | ||
37 | + }, | ||
38 | + "description": "Escape RegExp special characters", | ||
39 | + "devDependencies": { | ||
40 | + "ava": "*", | ||
41 | + "xo": "*" | ||
42 | + }, | ||
43 | + "engines": { | ||
44 | + "node": ">=0.8.0" | ||
45 | + }, | ||
46 | + "files": [ | ||
47 | + "index.js" | ||
48 | + ], | ||
49 | + "homepage": "https://github.com/sindresorhus/escape-string-regexp#readme", | ||
50 | + "keywords": [ | ||
51 | + "escape", | ||
52 | + "regex", | ||
53 | + "regexp", | ||
54 | + "re", | ||
55 | + "regular", | ||
56 | + "expression", | ||
57 | + "string", | ||
58 | + "str", | ||
59 | + "special", | ||
60 | + "characters" | ||
61 | + ], | ||
62 | + "license": "MIT", | ||
63 | + "maintainers": [ | ||
64 | + { | ||
65 | + "name": "Sindre Sorhus", | ||
66 | + "email": "sindresorhus@gmail.com", | ||
67 | + "url": "sindresorhus.com" | ||
68 | + }, | ||
69 | + { | ||
70 | + "name": "Joshua Boy Nicolai Appelman", | ||
71 | + "email": "joshua@jbna.nl", | ||
72 | + "url": "jbna.nl" | ||
73 | + } | ||
74 | + ], | ||
75 | + "name": "escape-string-regexp", | ||
76 | + "repository": { | ||
77 | + "type": "git", | ||
78 | + "url": "git+https://github.com/sindresorhus/escape-string-regexp.git" | ||
79 | + }, | ||
80 | + "scripts": { | ||
81 | + "test": "xo && ava" | ||
82 | + }, | ||
83 | + "version": "1.0.5" | ||
84 | +} |
node_modules/escape-string-regexp/readme.md
0 → 100644
1 | +# escape-string-regexp [](https://travis-ci.org/sindresorhus/escape-string-regexp) | ||
2 | + | ||
3 | +> Escape RegExp special characters | ||
4 | + | ||
5 | + | ||
6 | +## Install | ||
7 | + | ||
8 | +``` | ||
9 | +$ npm install --save escape-string-regexp | ||
10 | +``` | ||
11 | + | ||
12 | + | ||
13 | +## Usage | ||
14 | + | ||
15 | +```js | ||
16 | +const escapeStringRegexp = require('escape-string-regexp'); | ||
17 | + | ||
18 | +const escapedString = escapeStringRegexp('how much $ for a unicorn?'); | ||
19 | +//=> 'how much \$ for a unicorn\?' | ||
20 | + | ||
21 | +new RegExp(escapedString); | ||
22 | +``` | ||
23 | + | ||
24 | + | ||
25 | +## License | ||
26 | + | ||
27 | +MIT © [Sindre Sorhus](http://sindresorhus.com) |
node_modules/filelist/README.md
0 → 100644
1 | +## FileList | ||
2 | + | ||
3 | +A FileList is a lazy-evaluated list of files. When given a list | ||
4 | +of glob patterns for possible files to be included in the file | ||
5 | +list, instead of searching the file structures to find the files, | ||
6 | +a FileList holds the pattern for latter use. | ||
7 | + | ||
8 | +This allows you to define a FileList to match any number of | ||
9 | +files, but only search out the actual files when then FileList | ||
10 | +itself is actually used. The key is that the first time an | ||
11 | +element of the FileList/Array is requested, the pending patterns | ||
12 | +are resolved into a real list of file names. | ||
13 | + | ||
14 | +### Usage | ||
15 | + | ||
16 | +Add files to the list with the `include` method. You can add glob | ||
17 | +patterns, individual files, or RegExp objects. When the Array | ||
18 | +methods are invoked on the FileList, these items are resolved to | ||
19 | +an actual list of files. | ||
20 | + | ||
21 | +```javascript | ||
22 | +var fl = new FileList(); | ||
23 | +fl.include('test/*.js'); | ||
24 | +fl.exclude('test/helpers.js'); | ||
25 | +``` | ||
26 | + | ||
27 | +Use the `exclude` method to override inclusions. You can use this | ||
28 | +when your inclusions are too broad. | ||
29 | + | ||
30 | +### Array methods | ||
31 | + | ||
32 | +FileList has lazy-evaluated versions of most of the array | ||
33 | +methods, including the following: | ||
34 | + | ||
35 | +* join | ||
36 | +* pop | ||
37 | +* push | ||
38 | +* concat | ||
39 | +* reverse | ||
40 | +* shift | ||
41 | +* unshift | ||
42 | +* slice | ||
43 | +* splice | ||
44 | +* sort | ||
45 | +* filter | ||
46 | +* forEach | ||
47 | +* some | ||
48 | +* every | ||
49 | +* map | ||
50 | +* indexOf | ||
51 | +* lastIndexOf | ||
52 | +* reduce | ||
53 | +* reduceRight | ||
54 | + | ||
55 | +When you call one of these methods, the items in the FileList | ||
56 | +will be resolved to the full list of files, and the method will | ||
57 | +be invoked on that result. | ||
58 | + | ||
59 | +### Special `length` method | ||
60 | + | ||
61 | +`length`: FileList includes a length *method* (instead of a | ||
62 | +property) which returns the number of actual files in the list | ||
63 | +once it's been resolved. | ||
64 | + | ||
65 | +### FileList-specific methods | ||
66 | + | ||
67 | +`include`: Add a filename/glob/regex to the list | ||
68 | + | ||
69 | +`exclude`: Override inclusions by excluding a filename/glob/regex | ||
70 | + | ||
71 | +`resolve`: Resolve the items in the FileList to the full list of | ||
72 | +files. This method is invoked automatically when one of the array | ||
73 | +methods is called. | ||
74 | + | ||
75 | +`toArray`: Immediately resolves the list of items, and returns an | ||
76 | +actual array of filepaths. | ||
77 | + | ||
78 | +`clearInclusions`: Clears any pending items -- must be used | ||
79 | +before resolving the list. | ||
80 | + | ||
81 | +`clearExclusions`: Clears the list of exclusions rules. | ||
82 | + | ||
83 | + | ||
84 | + |
node_modules/filelist/index.d.ts
0 → 100644
1 | +// Type definitions for filelist v0.0.6 | ||
2 | +// Project: https://github.com/mde/filelist | ||
3 | +// Definitions by: Christophe MASSOLIN <https://github.com/FuriouZz> | ||
4 | +// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped | ||
5 | + | ||
6 | +declare module "filelist" { | ||
7 | + export class FileList { | ||
8 | + pendingAdd: string[] | ||
9 | + pending: boolean | ||
10 | + excludes: { | ||
11 | + pats: RegExp[], | ||
12 | + funcs: Function[], | ||
13 | + regex: null | RegExp | ||
14 | + } | ||
15 | + items: string[] | ||
16 | + static clone(): FileList | ||
17 | + static verbose: boolean | ||
18 | + toArray(): string[] | ||
19 | + include(options: any, ...items: string[]): void | ||
20 | + exclude(...items: string[]): void | ||
21 | + resolve(): void | ||
22 | + clearInclusions(): void | ||
23 | + clearExclusions(): void | ||
24 | + length(): number | ||
25 | + toString(): string; | ||
26 | + toLocaleString(): string; | ||
27 | + push(...items: string[]): number; | ||
28 | + pop(): string | undefined; | ||
29 | + concat(...items: ReadonlyArray<string>[]): string[]; | ||
30 | + concat(...items: (string | ReadonlyArray<string>)[]): string[]; | ||
31 | + join(separator?: string): string; | ||
32 | + reverse(): string[]; | ||
33 | + shift(): string | undefined; | ||
34 | + slice(start?: number, end?: number): string[]; | ||
35 | + sort(compareFn?: (a: string, b: string) => number): this; | ||
36 | + splice(start: number, deleteCount?: number): string[]; | ||
37 | + splice(start: number, deleteCount: number, ...items: string[]): string[]; | ||
38 | + unshift(...items: string[]): number; | ||
39 | + indexOf(searchElement: string, fromIndex?: number): number; | ||
40 | + lastIndexOf(searchElement: string, fromIndex?: number): number; | ||
41 | + every(callbackfn: (value: string, index: number, array: string[]) => boolean, thisArg?: any): boolean; | ||
42 | + some(callbackfn: (value: string, index: number, array: string[]) => boolean, thisArg?: any): boolean; | ||
43 | + forEach(callbackfn: (value: string, index: number, array: string[]) => void, thisArg?: any): void; | ||
44 | + map<U>(callbackfn: (value: string, index: number, array: string[]) => U, thisArg?: any): U[]; | ||
45 | + filter<S extends string>(callbackfn: (value: string, index: number, array: string[]) => value is S, thisArg?: any): S[]; | ||
46 | + filter(callbackfn: (value: string, index: number, array: string[]) => any, thisArg?: any): string[]; | ||
47 | + reduce(callbackfn: (previousValue: string, currentValue: string, currentIndex: number, array: string[]) => string): string; | ||
48 | + reduce(callbackfn: (previousValue: string, currentValue: string, currentIndex: number, array: string[]) => string, initialValue: string): string; | ||
49 | + reduce<U>(callbackfn: (previousValue: U, currentValue: string, currentIndex: number, array: string[]) => U, initialValue: U): U; | ||
50 | + reduceRight(callbackfn: (previousValue: string, currentValue: string, currentIndex: number, array: string[]) => string): string; | ||
51 | + reduceRight(callbackfn: (previousValue: string, currentValue: string, currentIndex: number, array: string[]) => string, initialValue: string): string; | ||
52 | + reduceRight<U>(callbackfn: (previousValue: U, currentValue: string, currentIndex: number, array: string[]) => U, initialValue: U): U; | ||
53 | + } | ||
54 | +} | ||
... | \ No newline at end of file | ... | \ No newline at end of file |
node_modules/filelist/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/filelist/jakefile.js
0 → 100644
node_modules/filelist/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/has-flag/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/has-flag/license
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/has-flag/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/has-flag/readme.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/isarray/.npmignore
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/isarray/.travis.yml
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/isarray/Makefile
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/isarray/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/isarray/component.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/isarray/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/isarray/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/isarray/test.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/Makefile
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/bin/bash_completion.sh
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/bin/cli.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/jakefile.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/api.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/jake.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/loader.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/namespace.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/package_task.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/parseargs.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/program.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/publish_task.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/rule.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/task/directory_task.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/task/file_task.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/task/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/task/task.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/test_task.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/utils/file.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/utils/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/lib/utils/logger.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/package.json
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/jake/test/integration/file.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/jake/test/integration/rule.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/jake/test/unit/jakefile.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/test/unit/namespace.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/test/unit/parseargs.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/jake/usage.txt
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/Changes.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/License
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/Readme.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/Connection.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/ConnectionConfig.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/Pool.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/PoolCluster.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/PoolConfig.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/PoolConnection.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/PoolNamespace.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/PoolSelector.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/protocol/Auth.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/protocol/Parser.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/protocol/Protocol.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/protocol/ResultSet.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/protocol/SqlString.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/mysql/lib/protocol/Timer.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff could not be displayed because it is too large.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/mysql/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/process-nextick-args/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/process-nextick-args/license.md
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/process-nextick-args/readme.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/readable-stream/.travis.yml
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/readable-stream/CONTRIBUTING.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/readable-stream/GOVERNANCE.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/readable-stream/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/readable-stream/README.md
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/readable-stream/duplex.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/readable-stream/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/readable-stream/passthrough.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/readable-stream/readable.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/readable-stream/transform.js
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/readable-stream/writable.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/sqlstring/HISTORY.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/sqlstring/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/sqlstring/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/sqlstring/index.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/sqlstring/lib/SqlString.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/sqlstring/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/string_decoder/.travis.yml
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/string_decoder/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/string_decoder/README.md
0 → 100644
This diff is collapsed. Click to expand it.
This diff is collapsed. Click to expand it.
node_modules/string_decoder/package.json
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/util-deprecate/History.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/util-deprecate/LICENSE
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/util-deprecate/README.md
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/util-deprecate/browser.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/util-deprecate/node.js
0 → 100644
This diff is collapsed. Click to expand it.
node_modules/util-deprecate/package.json
0 → 100644
This diff is collapsed. Click to expand it.
-
Please register or login to post a comment